How to Remove Class in jQuery
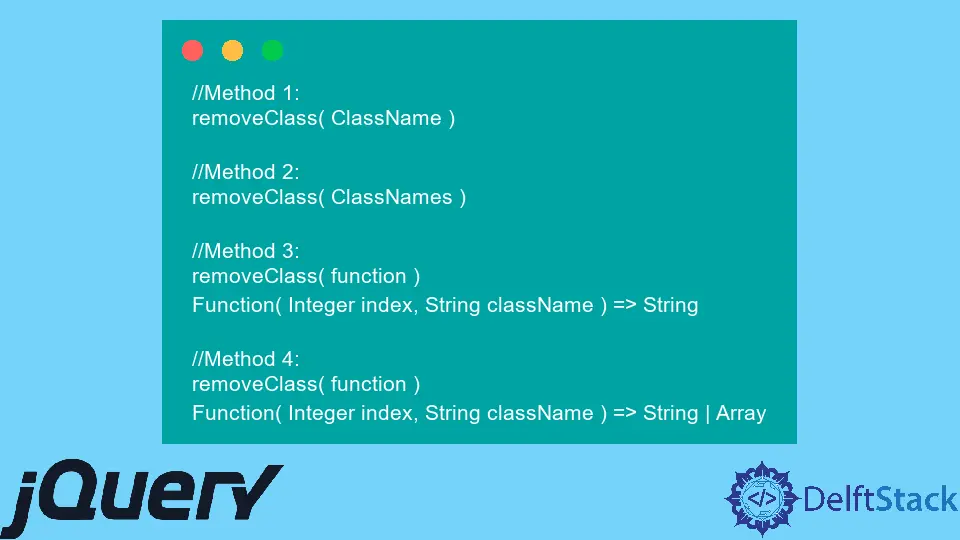
The removeclass()
method in jQuery removes single or multiple class names.
This tutorial demonstrates how to use the removeclass()
method in jQuery.
jQuery Remove Class
The removeclass()
method removes a single class or multiple classes from each HTML element from a group of elements or set of matched elements. The method can be used in several ways based on the number of classes.
See the syntax below.
// Method 1:
removeClass(ClassName)
// Method 2:
removeClass(ClassNames)
// Method 3:
removeClass(function)
Function(Integer index, String className) => String
// Method 4:
removeClass(function)
Function(Integer index, String className) => String | Array
- Method 1 removes one or more space-separated classes with the class attribute of every matched element. The
ClassName
parameter is a string for this method. - Method 2 removes multiple classes from a set of matched elements. The parameter
ClassNames
is an array for this method. - Method 3 removes one or more space-separated classes which are returned from the function. This method will receive the index of an element in the set, and the old class value will be as arguments.
- Method 4 removes one or more space-separated classes, which are returned from the function; the
className
can be the string or an array of classes. This method will also receive the index of an element in the set, and the old class value will be as arguments.
Let’s try examples for removeClass()
method.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).even().removeClass( "FontSize" );
</script>
</body>
</html>
The code above contains multiple paragraphs with more than one space-separated class, including FontSize
, UnderLine
, and Highlight
. The code will remove the FontSize
class from the even paragraphs.
See output:
To remove more than one space-separated class, see the example below.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).even().removeClass( "FontSize UnderLine Highlight" );
</script>
</body>
</html>
Similarly, we can remove multiple classes by giving space-separated class names in the removeClass
method. See output:
The code above removed the classes FontSize
, UnderLine
, and Highlight
from the even paragraphs.
Now let’s try to remove multiple classes using an array. See example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).even().removeClass( ["FontSize", "UnderLine", "Highlight"] );
</script>
</body>
</html>
The code above will have the same output as the example two because, in this example, the same multiple classes are given in an array to the removeclass
method. See output:
The removeClass
class without any parameters will remove all the classes from the given element. See example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).odd().removeClass( );
</script>
</body>
</html>
The code above will remove all the classes from odd paragraphs. See output:
Finally, the removeClass
example with function
as a parameter. See example:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).odd().removeClass(function(){
return $(this).attr( "class" );
});
</script>
</body>
</html>
The code above will remove all the classes from odd paragraphs. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook