How to Get Class in jQuery
- Understanding jQuery and Class Retrieval
- Using the .attr() Method
- Using the .prop() Method
- Using the .class() Method
- Conclusion
- FAQ
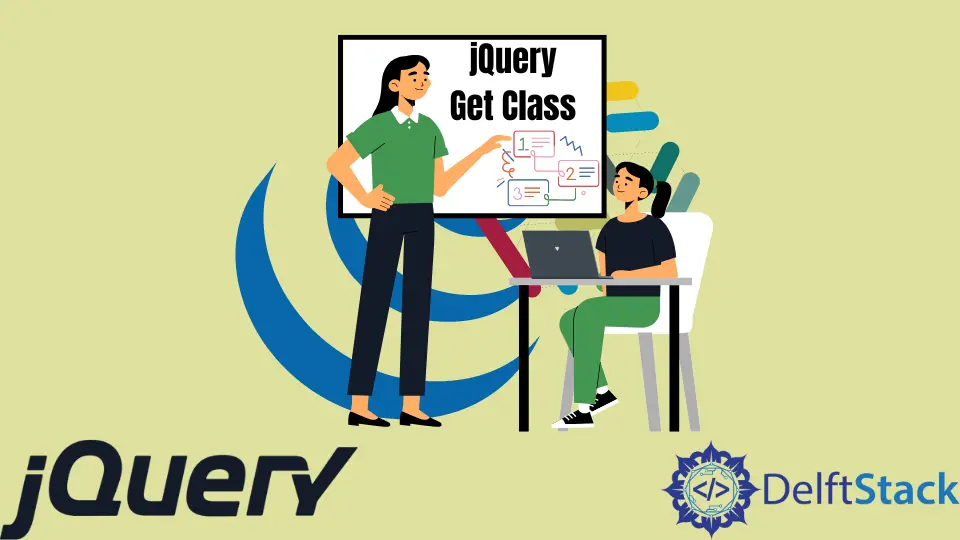
When working with jQuery, one of the most common tasks you might encounter is retrieving class names from elements. Whether you’re trying to manipulate styles, add interactivity, or simply gather information about elements on your page, understanding how to get class names is essential.
In this tutorial, we will explore various methods to achieve this using jQuery. We will guide you through the process step-by-step, ensuring that you can confidently retrieve class names from any HTML element. By the end of this article, you will have a solid understanding of how to use jQuery for this purpose, enhancing your web development skills.
Understanding jQuery and Class Retrieval
jQuery is a powerful JavaScript library that simplifies HTML document manipulation, event handling, and animation. One of its key features is the ability to easily access and modify the properties of DOM elements. When it comes to class names, jQuery offers several methods to retrieve them, making it a versatile tool for developers.
To get class names in jQuery, you can use methods like .attr()
, .prop()
, and .class()
. Each of these methods serves a different purpose and can be used in various scenarios. Let’s dive into the methods you can use to retrieve class names effectively.
Using the .attr() Method
The .attr()
method is one of the most straightforward ways to get the class attribute of an element. This method retrieves the value of the specified attribute for the first element in the set of matched elements. Here’s how you can use it:
$(document).ready(function() {
var className = $('#myElement').attr('class');
console.log(className);
});
Output:
example-class-name
In this example, we use jQuery to select an element with the ID myElement
. The .attr('class')
method retrieves the class attribute of that element. This is particularly useful when you want to check which classes are applied to a specific element. The retrieved class name can then be logged to the console or used in further operations. Keep in mind that if the element has multiple classes, they will be returned as a space-separated string.
Using the .prop() Method
Another way to get class names in jQuery is by using the .prop()
method. This method is similar to .attr()
, but it is more suitable for properties rather than attributes. Here’s how to use it to retrieve class names:
$(document).ready(function() {
var className = $('#myElement').prop('className');
console.log(className);
});
Output:
example-class-name
In this code snippet, we again select the element with the ID myElement
, but this time we use .prop('className')
. The className
property returns the element’s class attribute as a string, just like the .attr()
method. However, using .prop()
is generally preferred for properties because it ensures you’re getting the current state of the property rather than the initial value set in the HTML.
Using the .class() Method
While jQuery does not have a direct .class()
method, you can achieve similar results using the .hasClass()
method to check if an element has a specific class. However, if you want to retrieve all class names, you would typically combine it with the methods mentioned above. Here’s an example of how to use .hasClass()
:
$(document).ready(function() {
if ($('#myElement').hasClass('example-class-name')) {
console.log('Element has the class');
} else {
console.log('Element does not have the class');
}
});
Output:
Element has the class
In this example, we check if myElement
has a specific class called example-class-name
. The .hasClass()
method returns true
or false
, allowing you to conditionally execute code based on the presence of that class. This method is particularly useful for adding or removing classes based on certain conditions or user interactions.
Conclusion
Retrieving class names in jQuery is a fundamental skill for web developers. Whether you’re using the .attr()
, .prop()
, or .hasClass()
methods, each approach provides you with the flexibility to manipulate and interact with your HTML elements effectively. By mastering these techniques, you can enhance your web applications, making them more dynamic and user-friendly. Remember to experiment with these methods to find the best fit for your projects.
FAQ
-
How can I get multiple class names using jQuery?
You can use the.attr()
or.prop()
methods to retrieve all class names as a space-separated string, then split the string into an array if needed. -
Can I add a class to an element while retrieving its class names?
Yes, you can use the.addClass()
method to add a class to an element and then retrieve its class names using.attr()
or.prop()
. -
What is the difference between .attr() and .prop() in jQuery?
The.attr()
method retrieves the initial value of an attribute, while.prop()
retrieves the current state of a property.
-
Is jQuery necessary for modern web development?
While jQuery simplifies many tasks, modern JavaScript (ES6+) offers many built-in methods that can achieve similar results without jQuery. -
How can I check if an element has a specific class?
You can use the.hasClass()
method in jQuery to check if an element has a specific class.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook