How to Replace Class in jQuery
-
Use
removeClass()
andaddClass()
to Replace a Class in jQuery -
Use
attr()
to Replace a Class in jQuery
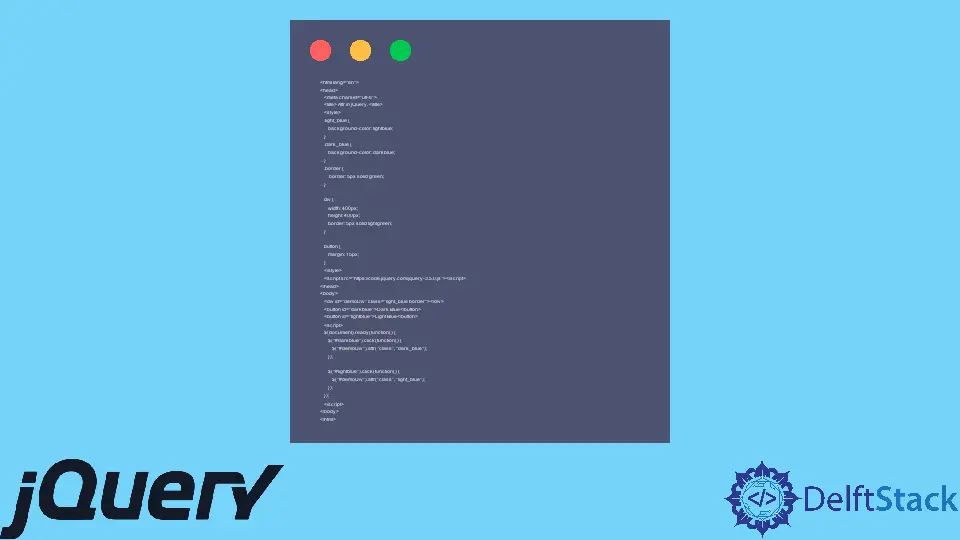
This tutorial demonstrates how to replace a class in jQuery.
jQuery doesn’t provide a built-in method to replace the classes of HTML elements, but it provides some other methods which can be used to achieve the class replacement functionality. This tutorial demonstrates different methods to replace a class with another class using jQuery.
Use removeClass()
and addClass()
to Replace a Class in jQuery
The removeClass()
function is used to remove a class from the element in jQuery and the addClass()
method will add a class to the HTML element. These classes can be implemented together to create a replaceClass
method.
Syntax for removeClass()
method:
$(Selector).removeClass(Class);
Syntax for addClass()
method:
$(Selector).addClass(Class);
Above, the class
is the class name that will be removed or added. Using both methods, we can create a function replaceClass
; let’s try an example.
<html lang="en">
<head>
<meta charset="utf-8">
<title> Remove and Add in jQuery. </title>
<style>
.light_blue {
background-color: lightblue;
}
.dark_blue {
background-color: darkblue;
}
div {
width: 400px;
height: 400px;
border: 5px solid lightgreen;
}
button {
margin: 15px;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="demoDiv" class="light_blue"></div>
<button id="darkblue">Dark Blue</button>
<button id="lightblue">Light Blue</button>
<script>
function replaceClass(Element_Id, Class1, Class2) {
var HTML_Element = $(`#${Element_Id}`);
if (HTML_Element.hasClass(Class1)) {
HTML_Element.removeClass(Class1);
}
HTML_Element.addClass(Class2);
}
$(document).ready(function() {
$("#darkblue").click(function() {
replaceClass("demoDiv", "light_blue", "dark_blue");
});
$("#lightblue").click(function() {
replaceClass("demoDiv", "dark_blue", "light_blue");
});
});
</script>
</body>
</html>
The code above creates function replaceClass
which uses the removeClass
and addClass
methods to replace a class of an HTML element.
The replaceClass()
function takes three parameters, first is the id of the element, second is the class to be replaced, and third is the class that will replace the given class. See the output:
Similarly, if we want to replace multiple classes, we can put spaces in the removeClass
and addClass
methods and then put the names of other classes. Let’s see an example.
<html lang="en">
<head>
<meta charset="utf-8">
<title> Remove and Add in jQuery. </title>
<style>
.light_blue {
background-color: lightblue;
}
.border1 {
border: 5px solid black;
}
.dark_blue {
border: 5px solid green;
}
.border2 {
background-color: darkblue;
}
div {
width: 400px;
height: 400px;
border: 5px solid lightgreen;
}
button {
margin: 15px;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="demoDiv" class="light_blue"></div>
<button id="darkblue">Dark Blue</button>
<button id="lightblue">Light Blue</button>
<script>
$(document).ready(function() {
$("#darkblue").click(function() {
$("#demoDiv").removeClass("light_blue border1")
$("#demoDiv").addClass("dark_blue border2")
});
$("#lightblue").click(function() {
$("#demoDiv").removeClass("dark_blue border2")
$("#demoDiv").addClass("light_blue border1")
});
});
</script>
</body>
</html>
As we can see, we put multiple class names in the methods removeClass
and addClass
, making sure the names are in one string divided by spaces.
The above code will change the background color and border of the div
by replacing two different classes. See the output:
Use attr()
to Replace a Class in jQuery
The attr
method in jQuery is used to access and change any attribute of an HTML element; it takes two parameters one is the attribute, and the second is the attribute name or properties with which the attribute will be replaced.
This will replace all the classes of the given element with a new class; the syntax for this method is below.
$(selector).attr('class', 'newClassName');
Above, the class
is the attribute, and the newClassName
will be the class name with which previous classes will be replaced. Let’s try an example.
<html lang="en">
<head>
<meta charset="utf-8">
<title> Attr in jQuery. </title>
<style>
.light_blue {
background-color: lightblue;
}
.dark_blue {
background-color: darkblue;
}
.border {
border: 5px solid green;
}
div {
width: 400px;
height: 400px;
border: 5px solid lightgreen;
}
button {
margin: 15px;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<div id="demoDiv" class="light_blue border"></div>
<button id="darkblue">Dark Blue</button>
<button id="lightblue">Light Blue</button>
<script>
$(document).ready(function() {
$("#darkblue").click(function() {
$("#demoDiv").attr("class", "dark_blue");
});
$("#lightblue").click(function() {
$("#demoDiv").attr("class", "light_blue");
});
});
</script>
</body>
</html>
The code above uses the attr
method to replace all the classes with a new class for an HTML element. See the output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook