How to Select Multiple Classes in jQuery
- Select Multiple Classes in jQuery: The Class Intersection Approach
-
Select Multiple Classes in jQuery: Use the
filter()
Function
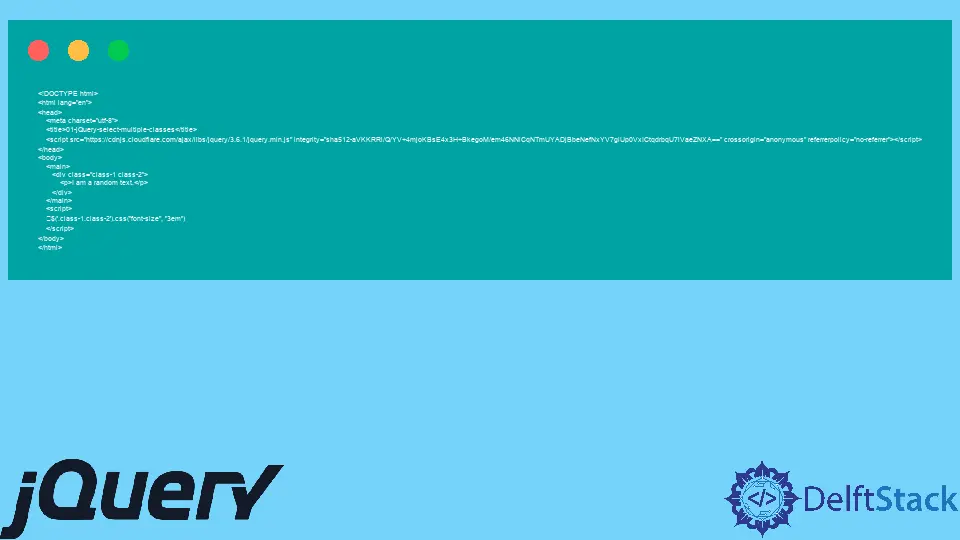
This article teaches you two methods that will select multiple classes in jQuery. Both methods will match elements that have the classes, but they do it differently.
Besides, when you include performance, one is faster than the other.
Select Multiple Classes in jQuery: The Class Intersection Approach
You can select multiple classes in jQuery using a class intersection approach. This is where you use the jQuery selector to grab the classes without spaces between them.
What’s more, the order that you write the classes does not matter. In the following code, we have an HTML element with two classes; class-1
and class-2
.
Afterward, we grab both classes using the class intersection approach. You can do what you want; we have changed the font size here.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-select-multiple-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<div class="class-1 class-2">
<p>I am a random text.</p>
</div>
</main>
<script>
$('.class-1.class-2').css("font-size", "3em")
</script>
</body>
</html>
Output:
Select Multiple Classes in jQuery: Use the filter()
Function
If an element has two classes, for example, class-a
and class-b
, you can use the filter()
function to select it. First, you’ll grab class-a
using the jQuery selector, then use the filter()
function to select class-b
.
This way, jQuery will select all elements with class-a
and remove all except those with class-b
.
The result is elements with class-a
and class-b
; that’s what we’ve done in the following code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-select-multiple-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
</head>
<body>
<main>
<div class="class-a class-b">
<p>Hello World</p>
</div>
</main>
<script>
$('.class-a.class-b').css("padding", "3em")
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn