jQuery 删除类
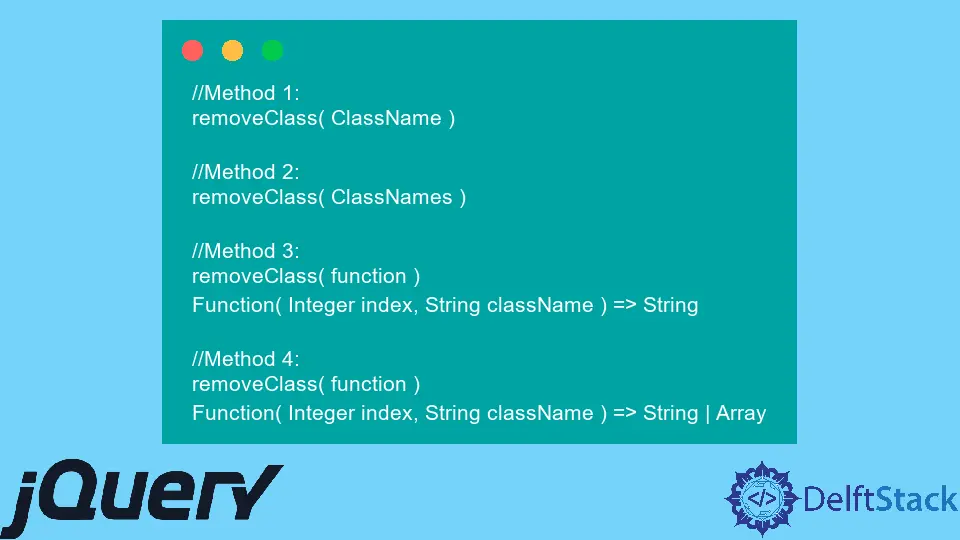
jQuery 中的 removeclass()
方法删除单个或多个类名。本教程演示了如何在 jQuery 中使用 removeclass()
方法。
jQuery 删除类
removeclass()
方法从一组元素或一组匹配元素中的每个 HTML 元素中删除单个类或多个类。该方法可以根据类的数量以多种方式使用。
请参阅下面的语法。
// Method 1:
removeClass(ClassName)
// Method 2:
removeClass(ClassNames)
// Method 3:
removeClass(function)
Function(Integer index, String className) => String
// Method 4:
removeClass(function)
Function(Integer index, String className) => String | Array
- 方法 1 删除一个或多个空格分隔的类,每个匹配元素的类属性。
ClassName
参数是此方法的字符串。 - 方法 2 从一组匹配的元素中删除多个类。参数
ClassNames
是此方法的数组。 - 方法 3 删除从函数返回的一个或多个空格分隔的类。此方法将接收集合中元素的索引,旧的类值将作为参数。
4、方法 4 删除一个或多个空格分隔的类,这些类是函数返回的;className
可以是字符串或类数组。此方法还将接收集合中元素的索引,并且旧的类值将作为参数。
让我们试试 removeClass()
方法的示例。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).even().removeClass( "FontSize" );
</script>
</body>
</html>
上面的代码包含多个段落,其中包含多个以空格分隔的类,包括 FontSize
、UnderLine
和 Highlight
。该代码将从偶数段落中删除 FontSize
类。
见输出:
要删除多个以空格分隔的类,请参见下面的示例。
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).even().removeClass( "FontSize UnderLine Highlight" );
</script>
</body>
</html>
类似地,我们可以通过在 removeClass
方法中提供以空格分隔的类名来删除多个类。见输出:
上面的代码从偶数段落中删除了类 FontSize
、UnderLine
和 Highlight
。
现在让我们尝试使用数组删除多个类。参见示例:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).even().removeClass( ["FontSize", "UnderLine", "Highlight"] );
</script>
</body>
</html>
上面的代码将具有与示例 2 相同的输出,因为在此示例中,相同的多个类在数组中提供给 removeclass
方法。见输出:
没有任何参数的 removeClass
类将从给定元素中删除所有类。参见示例:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).odd().removeClass( );
</script>
</body>
</html>
上面的代码将从奇数段中删除所有类。见输出:
最后,以 function
作为参数的 removeClass
示例。参见示例:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery removeClass</title>
<style>
p {
font-weight: bolder;
}
.FontSize {
font-size: 25px;
}
.UnderLine {
text-decoration: underline;
}
.Highlight {
background: lightgreen;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.0.js"></script>
</head>
<body>
<p class="FontSize UnderLine">Hello</p>
<p class="FontSize UnderLine Highlight">This</p>
<p class="FontSize UnderLine Highlight">is</p>
<p class="FontSize UnderLine">Delftstack</p>
<p class="FontSize UnderLine">Site</p>
<script>
$( "p" ).odd().removeClass(function(){
return $(this).attr( "class" );
});
</script>
</body>
</html>
上面的代码将从奇数段中删除所有类。见输出:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook