How to Loop Through a JavaScript Object
-
for-in
Loop to Loop Through JavaScript Object -
Use the
for-of
Loop to Loop Through JavaScript Object -
Use the
forEach
Method to Loop Through the JavaScript Object
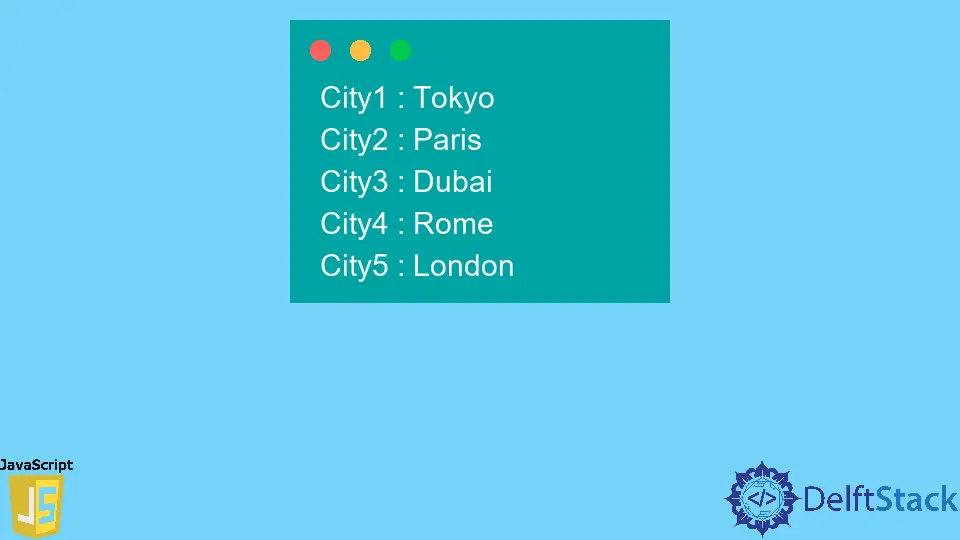
This tutorial article will introduce how we can iterate a JavaScript object.
There are several looping statements, and a method used to iterate through a JavaScript object is as follows.
for-in
loopfor-of
loopforEach
method
for-in
Loop to Loop Through JavaScript Object
The for-in
statement is a loop used to iterate the JavaScript object with key-value pairs. This loop iterates over all the elements of the JavaScript object.
The example code of using the for-in
loop to loop through the JavaScript object is as follows.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
} for (var city in Cities) {
console.log(city, ':', Cities[city]);
}
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London
While using the for-in
loop, we don’t have to use any special function to iterate or loop an object.
Use the for-of
Loop to Loop Through JavaScript Object
The for-of
statement is also a loop that iterates an iterable object that does not have key-value pairs. We can also use this loop to iterate the JavaScript object with key-value pairs but with some special functions as follows.
Object.getOwnPropertyNames
Object.entries
Object.keys
Reflect.ownKeys
Object.getOwnPropertyNames
This method returns an array of all the keys of the key-value pairs stored in the object. But to iterate all the pairs of key-value of the JavaScript object, we need to use this method with a for-of
loop.
The example code of using the for-of
with Object.getOwnPropertyNames()
to iterate the object is as follows.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
};
for (var city of Object.getOwnPropertyNames(Cities)) {
const CityName = Cities[city];
console.log(city, ':', CityName);
}
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London
Object.entries
This method returns the array of the key-value pair of the object. But to iterate a key and value, we need to use the for-of
loop with this method to loop through the JavaScript object.
The example code of using the for-of
with Object.entries()
to iterate the object is as follows.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
};
for (var [city, CityName] of Object.entries(Cities)) {
console.log(city, ':', CityName);
}
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London
Object.keys
This method returns the array of the keys of the object. But to iterate key-value pairs of an object, we need to use this function with the for-of
loop.
The example code of using the for-of
with Object.keys()
to iterate the object is as follows.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
};
for (var city of Object.keys(Cities)) {
console.log(city, ':', cities[city]);
}
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London
Reflect.ownKeys
This method also returns the keys of the object. But to iterate key-value pairs of the object, we need to use this function with the for-of
loop.
The example code of using the for-of
with Reflect.ownKeys
to iterate the object is as follows.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
};
for (var city of Reflect.ownKeys(Cities)) {
console.log(city, ':', cities[city]);
}
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London
Use the forEach
Method to Loop Through the JavaScript Object
The forEach
method works as an iterator to call the other method sequentially. But we can use this method to iterate the object by using several other functions that are as follows.
Object.keys
Reflect.ownKeys
Object.keys
Using this method with the forEach
method, we can iterate the key-value pairs of an object.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
};
Object.keys.forEach(city => {console.log(city, ':', Cities[city])})
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London
Reflect.ownKeys
Using this method with the forEach
method, we can iterate the key-value pairs of an object.
var Cities = {
City1: 'Tokyo',
City2: 'Paris',
City3: 'Dubai',
City3: 'Rome',
City4: 'London'
};
Reflect.ownKeys.forEach(city => {console.log(city, ':', Cities[city])})
Output:
City1 : Tokyo
City2 : Paris
City3 : Dubai
City4 : Rome
City5 : London