How to Update Object in JavaScript Array
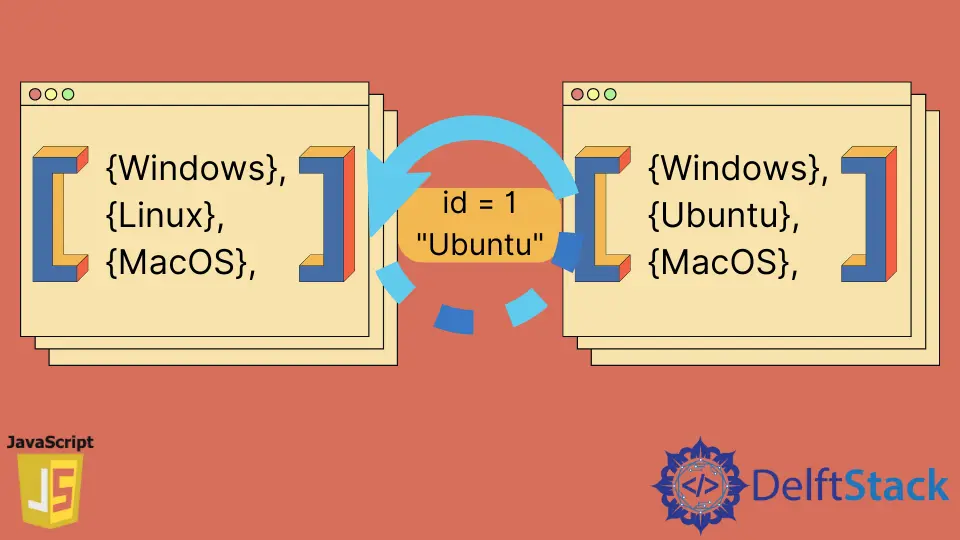
Arrays are objects in JavaScript with a fixed numeric key and dynamic values that can contain any amount of data in a single variable. An array can be one-dimensional or multi-dimensional. The JavaScript array can store values directly or store JavaScript objects. Unlike other languages, JS arrays can contain different data types at different indexes of the same array.
In today’s post, we will find out how to update the object of an array in JavaScript.
JavaScript offers two ways to update the object, using map()
and findIndex()
.
Update Object Using map()
in JavaScript
This built-in array method, provided by JavaScript, iterates over the original array and creates the new array, completing the elements based on the specified conditions. Instead of an arrow function, you can also pass the callback function. The only difference between map()
and forEach()
is that map()
will create the new array while forEach()
mutates the original array.
Syntax of map()
in JavaScript
Array.prototype.map(element => $updateCondition);
Array.prototype.map($callbackFn);
Parameter
$updateCondition
: This mandatory parameter specifies which action should be carried out for each element.
$callbackFn
: It is a mandatory parameter that specifies a function for each element of an array that is to be called. Each time the function is called, it returns the result to the new array.
Return Value
Returns the new array in which the elements are the result of the callback function.
Example code:
const osArray = [
{id: 0, name: 'Windows'},
{id: 1, name: 'Linux'},
{id: 2, name: 'MacOS'},
];
const updatedOSArray =
osArray.map(p => p.id === 1 ? {...p, name: 'Ubuntu'} : p);
console.log('After update: ', updatedOSArray);
Output:
After update: [
{id: 0, name: "Windows"},
{id: 1, name: "Ubuntu"},
{id: 2, name: "MacOS"}
]
Update Object Using findIndex()
in JavaScript
This built-in array method is provided by JavaScript, which finds the index of elements whose value matches the specified condition. Instead of an arrow function, you can also pass the callback function. There is a single difference between the two methods, findIndex()
and indexOf()
. findIndex()
is used for complex matching conditions or object data types while indexOf()
is used for simple conditions or primitive data types.
Syntax of findIndex()
in JavaScript
Array.prototype.findIndex(element => $condition);
Array.prototype.findIndex($callbackFn);
Parameter
$condition
: It is a mandatory parameter. The user can pass any condition related to the array element and find the first matching element that meets the condition.
Return Value
Returns the numeric index that fulfills the specified condition. If no element fulfills the specified condition, -1
is returned.
Example code:
const osArray = [
{id: 0, name: 'Windows'},
{id: 1, name: 'Linux'},
{id: 2, name: 'MacOS'},
];
elementIndex = osArray.findIndex((obj => obj.id == 1));
console.log('Before update: ', osArray[elementIndex]);
osArray[elementIndex].name = 'Ubuntu';
console.log('After update: ', osArray[elementIndex]);
Output:
Before update: {id: 1, name: 'Linux'}
After update: {id: 1, name: 'Ubuntu'}
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn