How to Check if a String Contains Substring in JavaScript
- Use Regular Expression to Check if a String Contains a Substring in JavaScript
-
Use
String.includes()
to Check if a String Contains Substring in JavaScript -
Use
String.indexOf()
to Check if a String Contains Substring in JavaScript
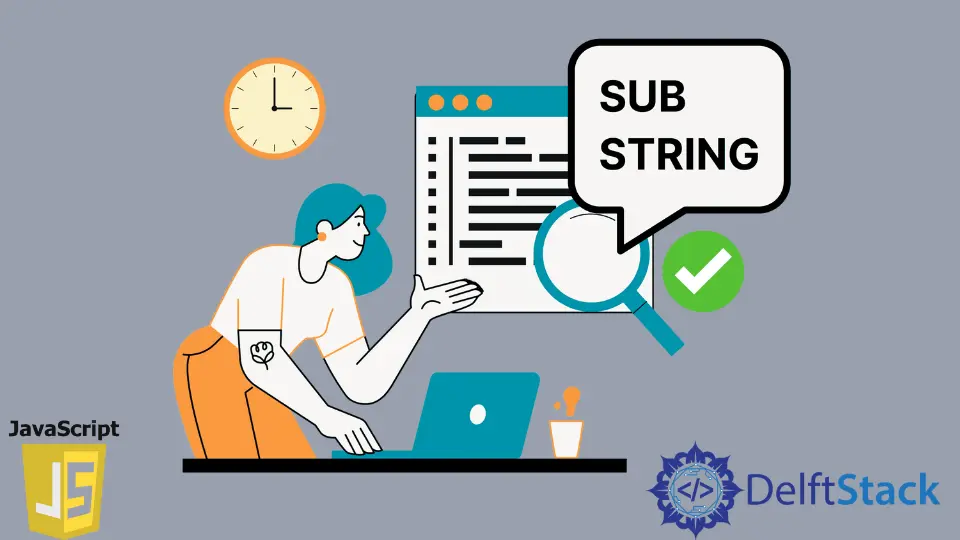
This tutorial teaches how to check if a string contains a substring in JavaScript.
It is a simple and common task, but different languages handle it in different ways. We will see the built-in methods in the JavaScript and custom-written methods to achieve the above goal.
Use Regular Expression to Check if a String Contains a Substring in JavaScript
It is one of the most powerful and traditional methods to check the presence of a substring. It gives us a lot of flexibility compared to built-in methods because with the JavaScript built-in methods, we can only check a constant string and can’t change the case-sensitivity of their behavior. We will be using the RegExp.test()
method to check for substrings in a string.
let str = 'delftstack';
console.log(/stack/.test(str));
Output:
true
Here we use the .test()
function to see if the substring stack
is present inside the string delftstack
and get true
as a result.
But the beauty of regex lies in the fact that we can do case insensitive searches, which is not possible with built-in JavaScript methods, and we can even look for patterns like a numeric substring of fixed length without even knowing what it is.
let str = 'delftstack';
console.log(stack / i.test(str));
Output:
true
We append i
to the expression /stack/
, and we can do the case insensitive search.
Use String.includes()
to Check if a String Contains Substring in JavaScript
ES6 introduced the .includes()
function that helps us to check if a given substring is present inside the string. It returns a boolean value telling presence by true
or false
. It is a case-sensitive method, and hence if substring S
is matched against s
, it will return false
.
This method takes 2 arguments: the first is the substring, and the second is the index from which we want to begin the search inside the string. If the second argument is out of the left bound i.e. it is less than 0
, then JavaScript starts the search from index 0
. If it is greater than the length of the string, it directly returns false.
const str = 'delftstack';
const substr = 'stack';
console.log(str.includes(substr));
Output:
true
In the above code, we pass the substring to the function, and it returns true
as stack
is present inside the string delftstack
.
const str = 'delftstack';
const substr = 'stack';
console.log(str.includes(substr, 4));
Output:
true
This code starts the searching of the word stack
from the 5th
index.
Use String.indexOf()
to Check if a String Contains Substring in JavaScript
Before ES6 introduced the String.includes()
method, the only built-in method was .indexOf()
. It is pretty similar to the String.includes()
method. Instead of returning a boolean value, this method returns the index of the first character of the substring if the substring is present inside the given string and -1
if the substring is not present.
const str = 'delftstack';
const substr = 'stack';
console.log(str.indexOf(substr) != -1);
Output:
true
It might be old but this is more precise as it can be used like .includes()
just by adding a check at the function’s result to see if it is not equal to -1
. Unlike .includes()
, it returns to us the exact index of the beginning of substring and can be of much more practical importance.
All the above methods except String.includes()
are supported by all the major browsers. String.includes()
was introduced by ES6 and is still not supported by Internet Explorer. One more method to do this will be to use the KMP (Knuth-Morris-Pratt) algorithm, an O(n)
algorithm with extensive applications. It is a huge topic in itself, and we will cover that in a future post.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn