How to Store Key-Value Array in JavaScript
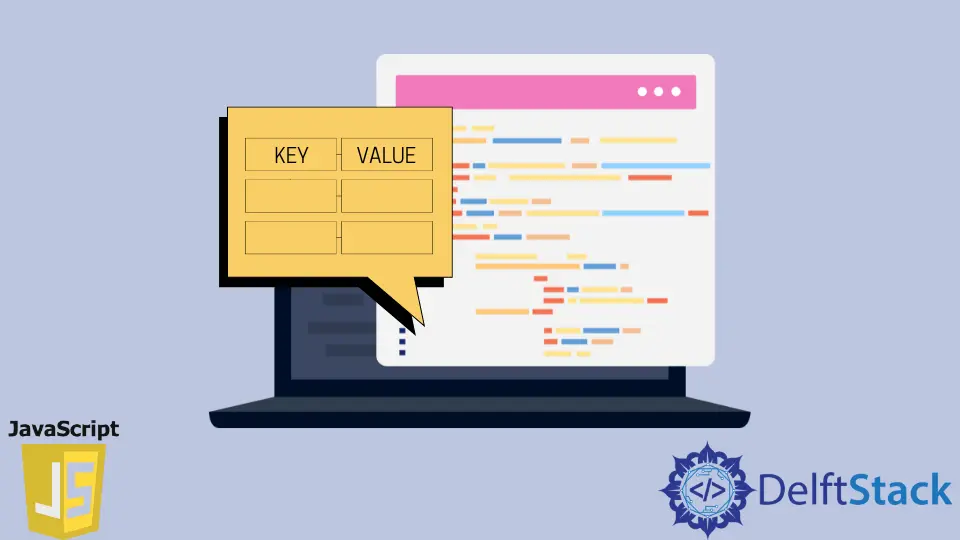
Storing key-value pairs in JavaScript is a fundamental aspect of programming in this versatile language. Whether you are dealing with configurations, user data, or any other type of information, understanding how to efficiently manage key-value arrays can enhance your coding skills significantly.
In this article, we will explore various methods to store key-value arrays in JavaScript, focusing on practical examples and clear explanations. By the end, you will have a solid grasp of how to effectively use key-value pairs in your JavaScript projects, making your code cleaner and more efficient.
Using Objects
One of the simplest ways to store key-value pairs in JavaScript is by using objects. Objects are collections of properties, where each property is defined as a key-value pair. This method is straightforward and widely used due to its simplicity and ease of access.
Here’s an example of how to create and use an object to store key-value pairs:
const user = {
name: "Alice",
age: 30,
email: "alice@example.com"
};
console.log(user.name);
console.log(user['age']);
Output:
Alice
30
In this example, we create an object named user
that contains three key-value pairs: name
, age
, and email
. You can access the values using either dot notation (e.g., user.name
) or bracket notation (e.g., user['age']
). Objects are dynamic, meaning you can add or remove properties at any time, making them highly flexible for various applications.
Using Arrays of Objects
Another effective method for storing key-value pairs is to utilize arrays of objects. This approach is particularly useful when you need to manage a collection of related items, such as a list of users or products. Each object in the array can represent a single item with its associated key-value pairs.
Here’s an example:
const users = [
{ name: "Alice", age: 30, email: "alice@example.com" },
{ name: "Bob", age: 25, email: "bob@example.com" },
{ name: "Charlie", age: 35, email: "charlie@example.com" }
];
users.forEach(user => {
console.log(user.name);
});
Output:
Alice
Bob
Charlie
In this case, we create an array named users
, which contains three objects. Each object represents a user with their respective properties. By using the forEach
method, we can easily iterate through the array and access each user’s name. This method is particularly useful for handling multiple entries and allows for efficient data manipulation and retrieval.
Using Maps
If you need a more advanced structure for storing key-value pairs, JavaScript provides the Map
object. Maps offer several advantages over plain objects, including the ability to use any value (including functions and objects) as keys. Additionally, Maps maintain the order of insertion, making them ideal for certain applications.
Here’s how you can create and use a Map:
const userMap = new Map();
userMap.set('name', 'Alice');
userMap.set('age', 30);
userMap.set('email', 'alice@example.com');
console.log(userMap.get('name'));
console.log(userMap.has('age'));
Output:
Alice
true
In this example, we create a new Map
called userMap
. We use the set
method to add key-value pairs and the get
method to retrieve a value by its key. The has
method checks if a specific key exists in the map. Maps are particularly useful when you need to store dynamic data and require advanced features like iteration and size tracking.
Conclusion
Storing key-value arrays in JavaScript is essential for efficient data management. Whether you choose to use objects, arrays of objects, or Maps, each method has its unique advantages and use cases. By understanding these techniques, you can write cleaner, more maintainable code that effectively handles data in your applications. As you continue to explore JavaScript, consider how these storage methods can enhance your projects and streamline your development process.
FAQ
-
What is the difference between an object and a Map in JavaScript?
An object is a simple key-value store, while a Map can use any value as a key and maintains the order of insertion. Maps also provide built-in methods for various operations. -
Can I store functions in a Map?
Yes, Maps allow you to store functions as values, making them versatile for various applications. -
How do I remove a key-value pair from a Map?
You can use thedelete
method to remove a key-value pair from a Map by specifying the key. -
Are arrays of objects slower than using a single object?
Generally, arrays of objects can be slightly slower due to the overhead of managing multiple objects, but they offer greater flexibility for handling collections of data. -
Can I nest objects within objects in JavaScript?
Yes, you can nest objects within objects, allowing for complex data structures and hierarchies.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript