How to Map A Filter in JavaScript
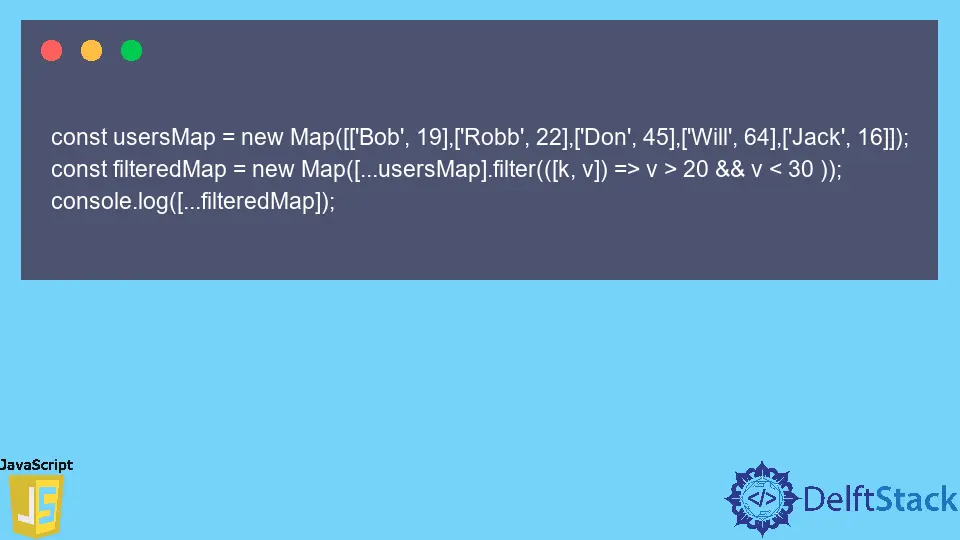
In web development, usually, the developers use HTML and CSS to build static websites/web pages. They use JavaScript (JS), a powerful scripting language with various useful features to make them interactive and dynamic.
JavaScript provides us with two features from its ocean of features: the map()
function and the filter()
function. The map()
function will create a new array with key-value pairs.
Through the filter()
function, we can build a new array with elements that have passed a test given by a process.
JavaScript Map Filter
Now, imagine that we should filter a map in JavaScript. Let’s see how to finish the task successfully.
First, let’s create a map to demonstrate this task, as shown below.
const usersMap = new Map(
[['Bob', 19], ['Robb', 22], ['Don', 45], ['Will', 64], ['Jack', 16]]);
In the above, we have created a map with some data and assigned it to a variable called usersMap
as our new map class. The data consists of the names of five users and their ages.
Let’s say we need to filter out the users whose age is less than 30 years. We can utilize the filter()
function to perform that.
See the given code block.
const filteredMap = new Map([...usersMap].filter(([k, v]) => v < 30));
As mentioned above, inside the filter()
function, we define the criteria we want to test over the map we created. We have used […usersMap]
to indicate that we are filtering the earlier-made map with the user’s information.
Then we assigned the above process to a newly created variable called filteredMap
. Then we printed out the filtered map using the console.log()
function as below.
console.log([...filteredMap]);
Now, if we execute this code, the following result will appear.
Output:
As you can see, we get the information of the users whose age is less than 30 years.
Now let’s think we need the user information whose age is between 20 and 30. Let’s implement our code by modifying the criteria in the filter()
function.
See the below code snippet.
const usersMap = new Map(
[['Bob', 19], ['Robb', 22], ['Don', 45], ['Will', 64], ['Jack', 16]]);
const filteredMap = new Map([...usersMap].filter(([k, v]) => v > 20 && v < 30 ));
console.log([...filteredMap]);
As shown above, we have changed our criteria and now let’s try running this code.
Output:
In the above output, we get only one user since our map has only one user aged between 20 and 30.
Up to now, we have filtered only the values. Now let’s try filtering the keys in the map.
For this example, we will filter the users without 'Will'
as their name. Below is the code to achieve this task.
const usersMap = new Map(
[['Bob', 19], ['Robb', 22], ['Don', 45], ['Will', 64], ['Jack', 16]]);
const filteredMap = new Map([...usersMap].filter(([k, v]) => k != 'Will'));
console.log([...filteredMap]);
Output:
Above is the result we get after running the code. As you can see, we get the result including the users whose name is not equal to 'Will'
.
Conclusion
This write-up discusses how we can filter a map using JavaScript’s filter()
function. Firstly, we created a map and then used the filter()
method to filter the information under different criteria.
Other than the technique mentioned above, there are more methods to filter maps in JavaScript, but we can quickly achieve our goal through this method.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.