How to Sort an ES6 Map in JavaScript
- An Overview of ES6 Map in JavaScript
-
Use the
.sort()
Method to Sort an ES6 Map in JavaScript - Sort an ES6 Map by String Keys in JavaScript
- Sort an ES6 Map by Number Keys in JavaScript
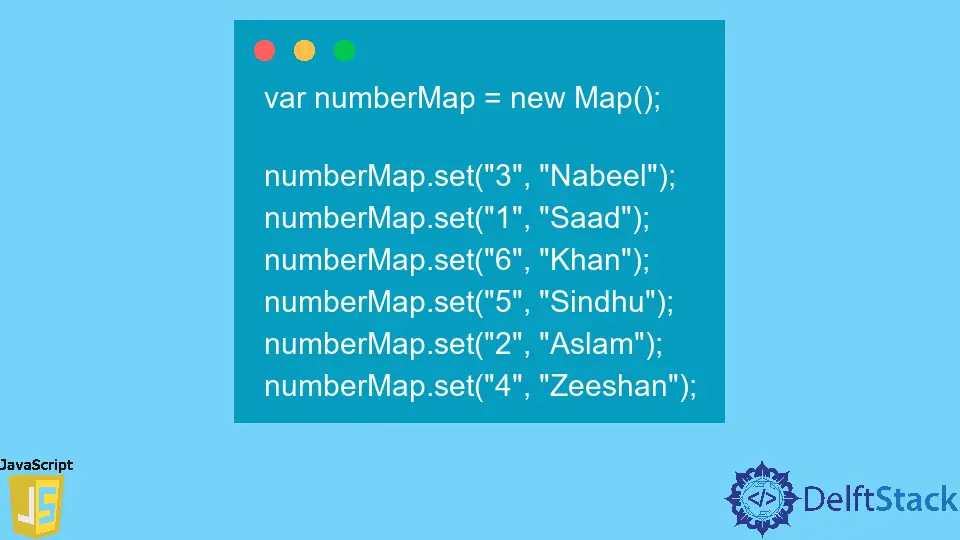
This article details the essential steps for sorting an ES6 map using JavaScript and demonstrates those procedures.
An Overview of ES6 Map in JavaScript
Using an object as a map has several drawbacks addressed by the new collection type named Map, which was introduced in ES6.
Map object stores key-value pairs by definition and values of any type may be used for either the keys or the values of the pairs it stores. In addition, a Map object will remember the original sequence in which the keys were inserted into the Map.
Use the .sort()
Method to Sort an ES6 Map in JavaScript
If you want to sort the keys in a Map, you may use the .sort()
method to do so; for example, var waqarMap = new Map([...mapA].sort())
. Using the Spread operator (...),
we can get all of the Map’s entries in an array, which we will then sort using the sort technique.
To sort the members in the array, the sort()
function makes use of the following logic:
- If the comparison function’s return value is larger than 0, the array should be sorted with
b
coming beforea
. - If the return value of the comparison function is smaller than 0, then
a
should come beforeb
in the sorting order. - If the comparison function’s return result equals 0, the order of
a
andb
should remain the same as before.
We will arrange the Map in two ways: first, based on the keys being strings, and second, based on the keys being numbers.
Let’s get started with the string key components first.
Sort an ES6 Map by String Keys in JavaScript
Make a new variable called waqarMap
and initialize it by producing an object of the Map()
class.
var waqarMap = new Map();
Now, we will add some values to the Map using the .set()
function, which takes two parameters. The first parameter is the key used to perform the sorting, and the second parameter is the value of the key that will be printed in the console next to the key.
At this point, we have our Map in hand, and it is prepared to be sorted.
waqarMap.set('c', 'three');
waqarMap.set('e', 'five');
waqarMap.set('d', 'four');
waqarMap.set('b', 'two');
waqarMap.set('f', 'six');
waqarMap.set('a', 'one');
Simply using the sort()
function is all required to sort a Map whose keys are string values.
var sortedMap = new Map([...waqarMap].sort());
Now that the Map has been sorted, we need to print it out on the console.
console.log(sortedMap);
Source Code to Sort an ES6 Map by String Keys in Ascending Order
var waqarMap = new Map();
waqarMap.set('c', 'three');
waqarMap.set('e', 'five');
waqarMap.set('d', 'four');
waqarMap.set('b', 'two');
waqarMap.set('f', 'six');
waqarMap.set('a', 'one');
var sortedMap = new Map([...waqarMap].sort());
console.log(sortedMap);
Output in Ascending Order:
Map {
'a' => 'one',
'b' => 'two',
'c' => 'three',
'd' => 'four',
'e' => 'five',
'f' => 'six' }
When we apply the method .sort()
, another function has to be used here, termed .reverse().
This is necessary to sort the Map in descending order.
The rest of the steps are the same except for this one.
var sortedMap = new Map([...waqarMap].sort().reverse());
Output in Descending Order:
Map {
'f' => 'six',
'e' => 'five',
'd' => 'four',
'c' => 'three',
'b' => 'two',
'a' => 'one' }
Sort an ES6 Map by Number Keys in JavaScript
Make a new variable called numberMap,
and then initialize it by producing an object of the Map()
class. Add the values to the map using the .set()
function.
var numberMap = new Map();
numberMap.set('3', 'Nabeel');
numberMap.set('1', 'Saad');
numberMap.set('6', 'Khan');
numberMap.set('5', 'Sindhu');
numberMap.set('2', 'Aslam');
numberMap.set('4', 'Zeeshan');
Here, the Map’s keys are numbers, then the sort()
method requires that you supply it a function as an argument. A function determines the sort order sent in as an argument to the procedure.
var sortedMap = new Map([...numberMap.entries()].sort());
console.log(sortedMap);
Source Code to Sort an ES6 Map by Number Keys in Ascending Order
var numberMap = new Map();
numberMap.set('3', 'Nabeel');
numberMap.set('1', 'Saad');
numberMap.set('6', 'Khan');
numberMap.set('5', 'Sindhu');
numberMap.set('2', 'Aslam');
numberMap.set('4', 'Zeeshan');
var sortedMap = new Map([...numberMap.entries()].sort());
console.log(sortedMap);
Output in Ascending Order:
Map {
'1' => 'Saad',
'2' => 'Aslam',
'3' => 'Nabeel',
'4' => 'Zeeshan',
'5' => 'Sindhu',
'6' => 'Khan' }
We need to use another function called .reverse()
if we want to sort our data in the opposite order of how it was sorted. Because this step is required to sort the Map in descending order and the rest are identical, this is the only difference between the two procedures.
var sortedMap = new Map([...numberMap.entries()].sort().reverse());
Output in Descending Order:
Map {
'6' => 'Khan',
'5' => 'Sindhu',
'4' => 'Zeeshan',
'3' => 'Nabeel',
'2' => 'Aslam',
'1' => 'Saad' }
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn