JavaScript for...in VS for...of Loop
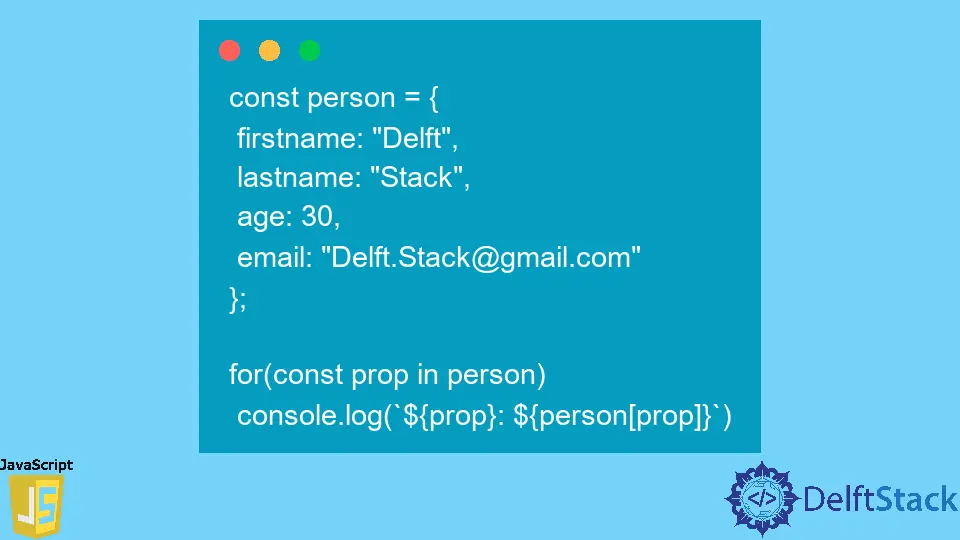
This article compares the for..in
and for..of
loop in JavaScript. It identifies the difference between them and shows where we can use them.
for..in
loops over the properties of an object. It gives back a key on each iteration which can be used to get the value of that particular key. The for..of
creates loop over the values of an iterable object including built-in Map
, Array
, Set
, String
, and TypedArray
.
Both have a variable
and an iterable
. For both, the variable
can be const
, let
, or var
. In for..of
, the iterable
is an object whose iterable properties are iterated while the iterable
of for..in
is an object itself.
Using the for..in
Loop in JavaScript
const person = {
firstname: 'Delft',
lastname: 'Stack',
age: 30,
email: 'Delft.Stack@gmail.com'
};
for (const prop in person) console.log(`${prop}: ${person[prop]}`)
Output:
"firstname: Delft"
"lastname: Stack"
"age: 30"
"email: Delft.Stack@gmail.com"
In the code given above, for..in
is looping over the properties of an object named person
. Let’s practice using the for..in
by iterating on its properties.
See the example code below.
const person = {
firstname: 'Delft',
lastname: 'Stack',
age: 30,
email: 'Delft.Stack@gmail.com'
};
function email() {
this.email = 'maofficial@gmail.com';
}
email.prototype = person;
var obj = new email();
for (const prop in obj) {
if (obj.hasOwnProperty(prop)) {
console.log(`obj.${prop} = ${obj[prop]}`);
}
}
Output:
obj.email = maofficial@gmail.com
The above code uses the for..in
loop to check whether the object has a particular property as its own or not using .hasOwnProperty()
. The .hasOwnProperty()
returns true
if the specified property is really its own property.
Using the for..of
Loop in JavaScript
Let’s start practicing the for..of
loop with an array.
const array = ['apple', 'banana', 'orange'];
for (const value of array) {
console.log(value);
}
Output:
apple
banana
orange
In the following code, the iterable
is a string.
const name = 'Delft';
for (const value of name) {
console.log(value);
}
Output:
M
e
h
v
i
s
h
The following code instance presents the use of the for..of
with Map
.
const personMap =
new Map([['firstname', 'Delft'], ['lastname', 'Stack'], ['age', 30]]);
for (const entry of personMap) {
console.log(entry);
}
Output:
[ 'firstname', 'Delft' ]
[ 'lastname', 'Stack' ]
[ 'age', 30 ]
Let’s practice the for..of
using an object that explicitly implements the iterable protocol.
const userDefinedfunctions = {
[Symbol.iterator]() {
return {
num: 0,
next() {
if (this.num < 10) {
return {value: this.num++, done: false};
}
return {value: undefined, done: true};
}
};
}
};
for (const value of userDefinedfunctions) console.log(value);
Output:
0
1
2
3
4
5
6
7
8
9
Symbol.iterator
is used as the default iterator for the object. This symbol is used by the for-of
loop where we have the iterable
whose iterable properties are iterated.