JavaScript for...in VS for...of 迴圈
Mehvish Ashiq
2023年10月12日
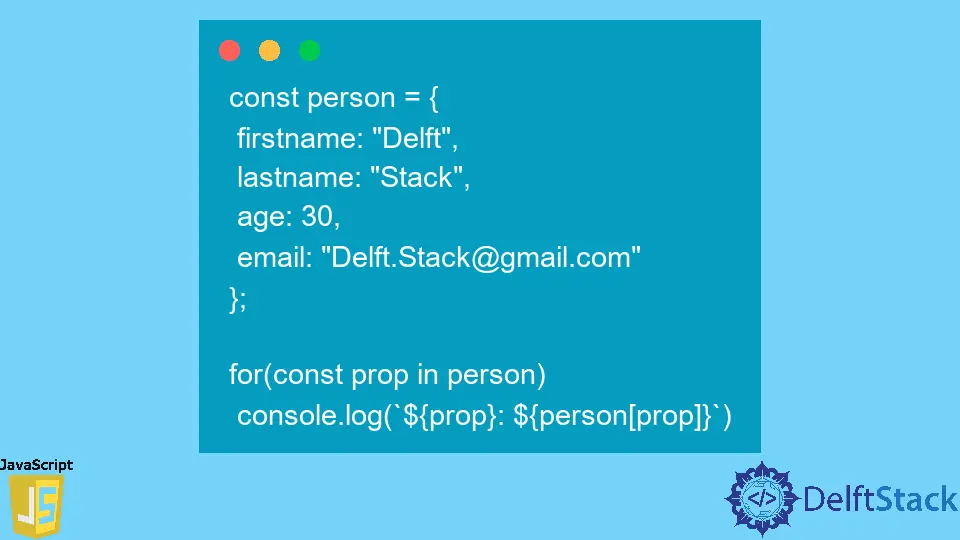
本文比較了 JavaScript 中的 for..in
和 for..of
迴圈。它識別了它們之間的區別並顯示了我們可以在哪裡使用它們。
for..in
迴圈物件的屬性。它在每次迭代時返回一個鍵,該鍵可用於獲取該特定鍵的值。for..of
在可迭代物件的值上建立迴圈,包括內建的 Map
、Array
、Set
、String
和 TypedArray
。
兩者都有一個 variable
和一個 iterable
。對於這兩種情況,variable
可以是 const
、let
或 var
。在 for..of
中,iterable
是一個物件,其可迭代屬性被迭代,而 for..in
的 iterable
是一個物件本身。
在 JavaScript 中使用 for..in
迴圈
const person = {
firstname: 'Delft',
lastname: 'Stack',
age: 30,
email: 'Delft.Stack@gmail.com'
};
for (const prop in person) console.log(`${prop}: ${person[prop]}`)
輸出:
"firstname: Delft"
"lastname: Stack"
"age: 30"
"email: Delft.Stack@gmail.com"
在上面給出的程式碼中,for..in
正在迴圈一個名為 person
的物件的屬性。讓我們通過迭代其屬性來練習使用 for..in
。
請參閱下面的示例程式碼。
const person = {
firstname: 'Delft',
lastname: 'Stack',
age: 30,
email: 'Delft.Stack@gmail.com'
};
function email() {
this.email = 'maofficial@gmail.com';
}
email.prototype = person;
var obj = new email();
for (const prop in obj) {
if (obj.hasOwnProperty(prop)) {
console.log(`obj.${prop} = ${obj[prop]}`);
}
}
輸出:
obj.email = maofficial@gmail.com
上面的程式碼使用 for..in
迴圈來檢查物件是否使用 .hasOwnProperty()
來檢查物件是否具有特定的屬性。如果指定的屬性確實是它自己的屬性,則 .hasOwnProperty()
返回 true
。
在 JavaScript 中使用 for..of
迴圈
讓我們開始用陣列練習 for..of
迴圈。
const array = ['apple', 'banana', 'orange'];
for (const value of array) {
console.log(value);
}
輸出:
apple
banana
orange
在下面的程式碼中,iterable
是一個字串。
const name = 'Delft';
for (const value of name) {
console.log(value);
}
輸出:
M
e
h
v
i
s
h
下面的程式碼例項展示了 for..of
與 Map
的用法。
const personMap =
new Map([['firstname', 'Delft'], ['lastname', 'Stack'], ['age', 30]]);
for (const entry of personMap) {
console.log(entry);
}
輸出:
[ 'firstname', 'Delft' ]
[ 'lastname', 'Stack' ]
[ 'age', 30 ]
讓我們使用顯式實現 iterable 協議的物件來練習 for..of
。
const userDefinedfunctions = {
[Symbol.iterator]() {
return {
num: 0,
next() {
if (this.num < 10) {
return {value: this.num++, done: false};
}
return {value: undefined, done: true};
}
};
}
};
for (const value of userDefinedfunctions) console.log(value);
輸出:
0
1
2
3
4
5
6
7
8
9
Symbol.iterator
用作物件的預設迭代器。這個符號由 for-of
迴圈使用,其中我們有 iterable
的可迭代屬性被迭代。
作者: Mehvish Ashiq