How to Create A Infinite Loop in JavaScript
-
Infinite Loop Using
while
Loop in JavaScript -
Infinite Loop Using
for
Loop in JavaScript -
Infinite Loop Using
setInterval
in JavaScript
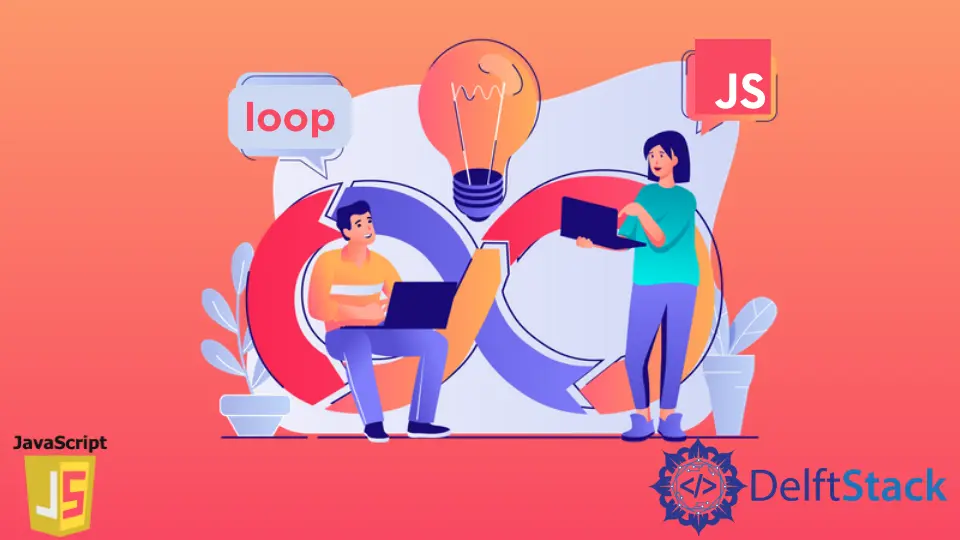
There are various loops available that serve a specific purpose and are used when the requirements match. We can use any of them to make it run infinite times.
In today’s post, we’ll learn about different types of loops and what is an infinite loop in JavaScript.
Infinite Loop Using while
Loop in JavaScript
The while
statement generates a loop that executes a specific statement as long as the test condition is true
. The condition is always evaluated before the statement inside it is executed.
Syntax:
while (condition) statement
To understand the while
loop, let’s look at the example below.
Example:
let i = 0
while (i < 3) {
console.log(i);
i += 1;
}
In the above example, i < 3
is the condition
. This is an expression that is evaluated before each step through the loop.
If this condition evaluates to true
, the statement written inside it is executed. So until the value of i
is less than 3, it will print the current value of i
.
Once the condition evaluates to false
, the while
loop execution stops and continues with the statement after the while
loop.
The console.log(i); i += 1
is the statement
that is an optional statement executed whenever the condition is true
. In our example, console.log
is the statement executed until the value of i
is less than 3.
When the above code is run in any browser, you will get the following output.
Output:
0
1
2
To make this loop work infinitely, specify the condition as true
, and it will run infinitely. The only drawback here is that JavaScript is single-threaded.
So it will block the current thread, and your window will freeze.
Infinite Loop Using for
Loop in JavaScript
The for
statement is an in-built loop provided by JavaScript. It creates a loop that takes three optional expressions, enclosed in parentheses.
These expressions are separated by semicolons, followed by a block statement that needs to be executed in the loop.
Syntax:
for ([initialization];[condition];[final - expression]) statement
To understand the for
loop, let’s look at the example below.
Example:
for (let i = 0; i < 3; i++) {
console.log(i)
}
// for(;;) {
// console.log("hello world!")
// }
In the above example, let i = 0
is the Initialization
of for
loop. It is an expression or variable declaration evaluated once before the loop begins.
This expression can declare new variables with the keywords var
or let
optionally. Variables declared with the var
keyword aren’t local to the loop; they are in the same scope as the for
loop.
Our variable i
is declared with let
, which is local to the statement.
The i < 3
is the condition
of for
loop. It is an expression evaluated before each iteration of the loop.
If this expression is true
, the statement is executed. In our example, until the value of i
is less than 3, it will print the current value of i
.
This conditioning test is an optional parameter. If omitted, the condition always evaluates to true
.
If the expression evaluates to false
, execution jumps to the first expression after the for
construct.
The i++
is the final-expression
. It is an expression evaluated at the end of each loop iteration.
This happens before the next evaluation of the condition. It updated the value of i
each time.
The console.log(i)
is the statement
. It is a statement executed whenever the condition evaluates to true
.
Find more information in the documentation of for()
loop.
When the above code is run in any browser, you will get the following output.
Output:
0
1
2
To make this loop work infinitely, do not specify any condition (as shown in commented code above), and it will run infinitely. The only drawback here is that JavaScript is single-threaded.
So it will block the current thread, and your window will freeze.
Infinite Loop Using setInterval
in JavaScript
The setInterval()
method is provided in JavaScript by default. This method, exposed in the Windows
and Worker
interfaces, repeatedly calls a function or executes a piece of code with a fixed time delay between each call.
The setInterval()
method returns an interval ID that uniquely identifies the interval so that you can later remove it by calling clearInterval()
.
Syntax:
const intervalID = setInterval(code, [delay]);
To understand the setInterval
, let’s look at the example below.
Example:
setInterval(() => {console.log('Hello world')}, 1);
console.log('Hello world')
is the code in the above example. An optional syntax that allows you to include a string instead of a function, compiled and executed every delay milliseconds.
1
is delay
, an optional parameter that specifies the time; in milliseconds, the timer should delay between executions of the specified function or code. The default value is 0 if not specified.
This function returns the interval ID
, a non-zero numeric value that identifies the timer created by the setInterval()
call. To cancel the interval, we can pass this value to clearInterval()
.
Find more information in the documentation for setInterval()
.
This function’s advantage is that it will not block the JavaScript execution, and it will return infinite times until the interval is cleared. To clear the interval call the clearInterval()
function.
Output:
'Hello world'
'Hello world'
'Hello world'
'Hello world'
'Hello world'
'Hello world'
'Hello world'
...
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn