How to Use forEach Index in JavaScript
-
JavaScript
forEach()
Index -
Arguments and Parameters of the
forEach()
Callback Function -
Print Odd and Even Values in a Given Array With
forEach()
-
Break/Continue or Terminate the Loop Using
forEach()
- Conclusion
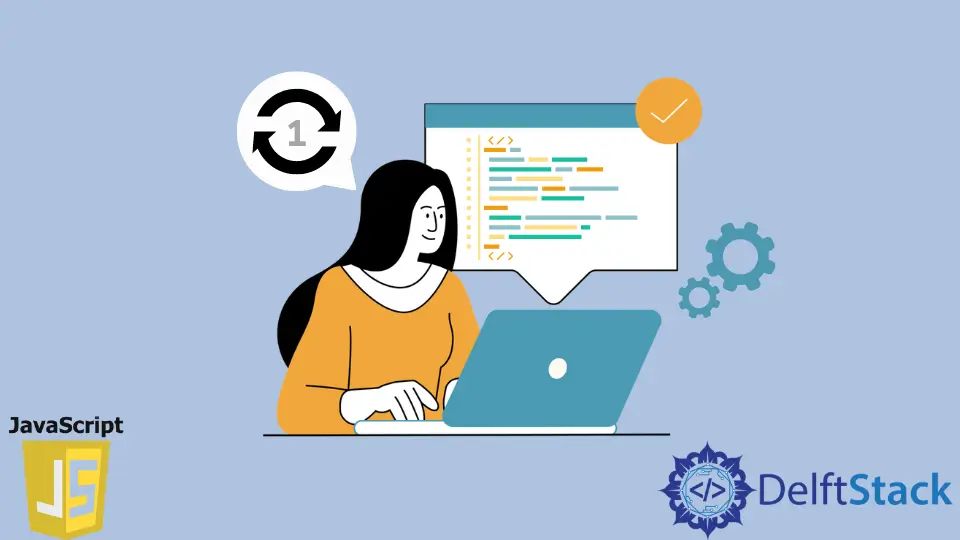
Out of the methods in JavaScript used for iterations in an array, forEach()
is the most commonly used.
JavaScript forEach()
Index
An array is an ordered list of values stored under an object specified by an index. forEach()
is looping through the indexed values in the array, starting from index[0]
to index[1]
, index[2]
, etc.
The forEach()
calls the function for each element in an array and returns undefined instead of returning a new array as it is not chainable. But this is not executed for empty elements and indexes that have been deleted or uninitialized.
Despite not being a mutator method with a callback function, forEach()
mutates the original array. When the forEach()
method traverses an array one element at a time in ascending index order, it is known as a callback function.
How forEach()
and Callback Function Works
At first, before calling the callback function, forEach()
processes the range of the elements of the array. Then, the callback function visits other indexes apart from already called upon indexes or those that are out of range.
If an existing element is changed or deleted at looping, the value passed before to the forEach()
function will be the default value. But the callback function will skip the elements removed or modified during the iteration.
Arguments and Parameters of the forEach()
Callback Function
The standardized method of the callback function of forEach()
consists of two arguments:
array.forEach(function(currentValue, index, array), thisArg)
The function
here is the function we use to call on values. As we execute every element, we use the callback function as the function
.
The callback function includes three parameters defined below:
currentValue
– The current element in the array (at loop time).index
– The index carries the index of thecurrentValue
.array
– The array of the current processing element belongs to.
The thisArg
is simply the value passed to this. Also, it refers to this value used in callback
.
The index
, array
, and thisArg
can be used in this standardized method if desired. Only function
and currentValue
are required.
Let’s clarify this through an example. Here, we have used an array of named letters by assigning some random letters in the alphabet.
var letters = ['b', 'e', 'f', 'j', 'u'];
Use the currentValue
Parameter
Code:
letters.forEach(function callback(letters) {
console.log(letters);
});
Output:
If we want to represent all the letters in the array individually, we can use forEach()
along with currentValue
. Here, we have used the letters
array as the currentValue
.
Using at least one parameter is a must when executing the function as it performs every single element within the array. To simplify the above code, we can use the arrow function representation as below.
Code:
letters.forEach(letters => console.log(letters))
Output:
Use the index
Parameter
To get the index of each element in the array, we can expand the code by adding the parameter index
shown below.
Code:
letters.forEach((letters, index) => {
console.log('Index of ' + letters + ' is ' + index);
});
Output:
Here the code displays the value of the element along with its index one by one.
Use the array
Parameter
Another optional parameter we use with the callback function is the array
. Using this parameter array
, we can print the arrays separately as below.
Code:
letters.forEach((letters, index, array) => {console.log(array)})
Output:
This code chunk prints the original array of similar types as the iterations and indexes it includes. By adapting to the above processes, we can implement the forEach()
function along with the parameters index
and array
depending on the use case.
So far, we have explained the arguments and parameters of the callback function separately. Below is another example using the forEach()
callback function.
Print Odd and Even Values in a Given Array With forEach()
Code:
var numbers = [15, 10, 9, 11, 16, 5];
numbers.forEach(function(currentValue, index, array) {
if (currentValue % 2) {
console.log(' ' + currentValue + ' is an odd number.');
} else {
console.log(' ' + currentValue + ' is an even number.');
}
});
Output:
Here, the numbers array consists of some random numbers we use in daily life. We have used the forEach()
function along with the parameters: currentValue
, index
, array
, and an if
conditional statement to print the number whether it is odd or not.
After each iteration, this will print the value as the output according to the if
conditional statement.
Break/Continue or Terminate the Loop Using forEach()
While looping, using break
or continue
is one method to terminate the array once the conditions are satisfied. As per other loops, let’s apply the break
and continue
to forEach()
.
Using break
:
Code:
var numbers = [15, 10, 9, 11, 16, 5];
numbers.forEach((numbers) => {
if (numbers == 9) break;
Output:
Using continue
:
Code:
var numbers = [15, 10, 9, 11, 16, 5];
numbers.forEach((numbers) => {
if (numbers == 9) continue;
console.log(numbers);
});
Output:
As in the output of both code snippets, it seems that using the forEach()
method makes the loop inability to terminate or use break
or continue
for the loop using forEach()
without throwing an exception inside the callback function. So forEach()
is not supported with the break
or continue
keywords.
Conclusion
The forEach()
function becomes an alternative to the for
loop by considering the code’s reusability and readability.
Code:
var letters = ['b', 'e', 'f', 'j', 'u'];
//using for() loop
Console.log("From For Loop")
for(var i=0; i< letters.length; i++){
console.log(letters [i]);
}
//using forEach() function
console.log("From ForEach() function")
letters.forEach(function(currentValue){
console.log(currentValue);
});
Output:
Overall, among the other features in ES5, forEach()
is one of the best features compatibility at its maximum.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.