How to Terminate a forEach Loop Using Exceptions in JavaScript
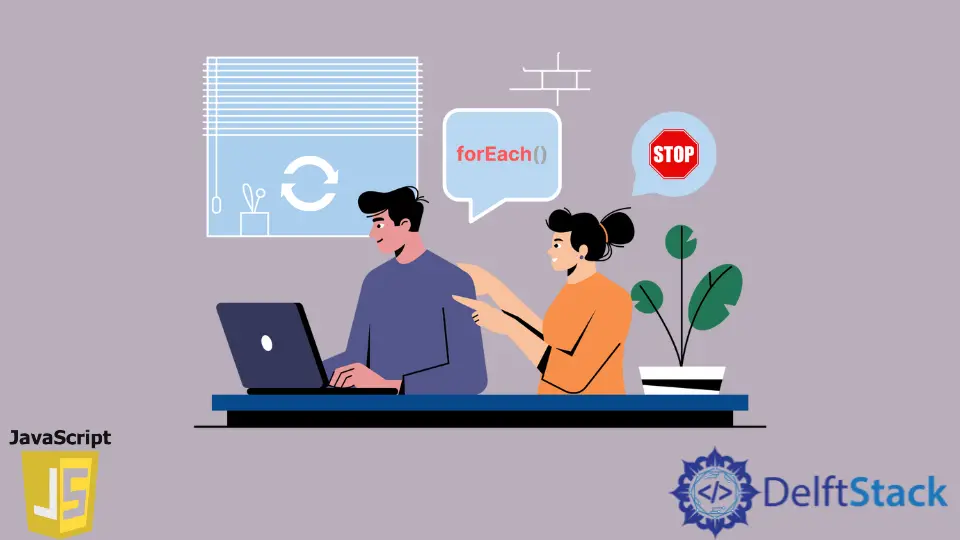
The JavaScript programming language comes with various variations of for
loop, unlike other programming languages, we only get to see the traditional for
loop, which consists of 3 things - initialization, condition, and increment or decrement. We have to follow these 3 elements to make a traditional for
loop work. It can seem quite confusing, especially if you are new to programming.
JavaScript has provided us with a new way of writing the for
loop, and that is the forEach
loop. The forEach
loop is used to iterate over an array. Many developers prefer using the forEach
loop over the traditional for
loop to iterate over an array because it is much easier to write and is much more readable.
Any loop, either a for
loop or a while
loop, can be terminated with the help of a break
statement. The only drawback with using the forEach
loop to iterate over an array is that it can’t be terminated using the break
keyword. There are times where we want to terminate a forEach
loop when some particular condition holds (either true or false) during the execution of the program. So, for that, we can use exception handling.
Terminate a forEach
Loop Using the try...catch
Block in JavaScript
To achieve the functionality provided by the break
statement inside the array.forEach
loop, we can use the concept of exception handling, which is available in JavaScript. The exception handling is nothing but handling abnormal conditions if some error occurs while running a program and avoiding unnecessary program crashes. This is done with the help of a try...catch
block.
The try
block is where you will be writing all of the code. And the catch
block will contain the code which will be used to handle the exception.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<p id="errorMsg"></p>
<script>
var arr = [1, 2, 3, 4, 5]
try {
arr.forEach((element)=> {
if (element === 4) throw "number is equal to four";
console.log(element);
});
}
catch (e) {
errorMsg = document.getElementById('errorMsg');
errorMsg.innerHTML = "Error: " + e + ".";
}
</script>
</body>
</html>
Output:
// The below output will be printed on the screen.
Error: number is equal to four.
// The below output will be printed inside the web browser console
1
2
3
This is our HTML 5 document. Inside the <body>
tag. We have one paragraph tag and the id
of which is errorMsg
. This paragraph tag will be used to show the error message which will be thrown by our JavaScript program.
Inside the <script>
tag, we have our actual program. Here, first, we have declared an array called arr
, which contains elements from 1 to 5. The main goal here is to iterate over this array using the forEach
loop and then break the loop when it reaches the element 4
inside the arr
array.
In the try
block, we use a forEach
loop to iterate over this array. Inside this loop, we have passed in an anonymous function (also known as arrow function). Inside that function, we have an if
statement to check if the element at a particular position in an array is 4
or not. If it is not 4
, it will print that element in the web browser’s console; otherwise, it will throw an exception, saying that the number is equal to four
.
This exception thrown by the try
block will be caught by the catch
block and stored inside the variable e
. Now we can show the error message present inside the variable e
inside the p
tag with the help of an HTML property called innerHTML
. This is an entire process of implementing a break
functionality inside the forEach
loop while iterating over an array in JavaScript.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn