JavaScript Counting
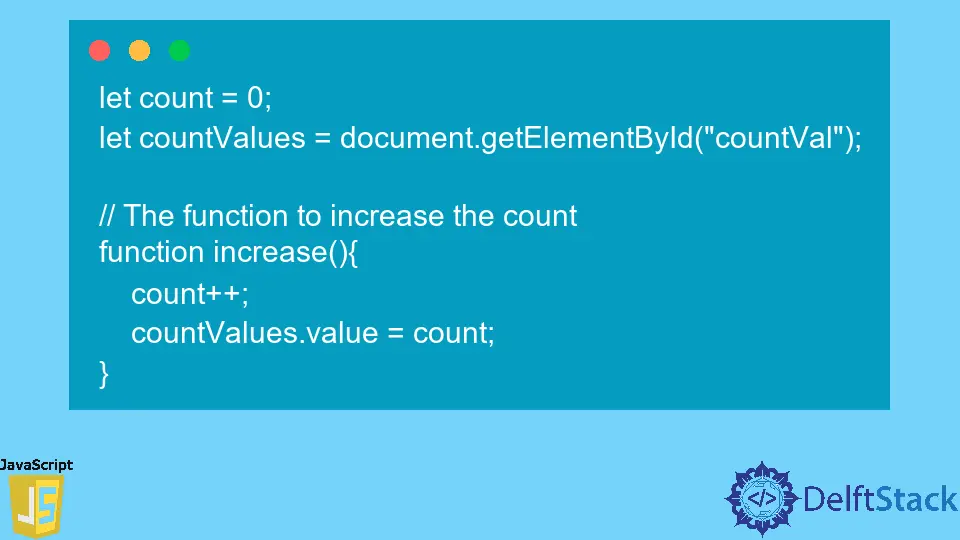
JavaScript has become very popular recently as a scripting language for web development. We can do numerous things or projects using JavaScript, and it provides lots of features to achieve them.
JavaScript Counting
Counting is a very essential for us in day-to-day life. Using programming languages, we can count various things to simplify things.
JavaScript also provides us with the functionalities to perform counting for various activities. Therefore, lots of implementations are easier to build with this.
Let’s try a simple code where we count numbers from 0 to 10. We will use a for
loop to achieve the goal.
for (let count = 0; count <= 10; count++) {
console.log(count);
}
The above code counts from 0 to 10, including 0. We will get the output shown below if we run the code.
Output:
As you can see, the code will print the numbers from 1 to 10, including both starting and ending numbers.
We can change the above code if we want to have even numbers. Refer to the below-modified code.
for (count = 0; count <= 10; count += 2) {
console.log(count);
}
Now, let’s try running this code to see the result.
Output:
As shown in the above output, we get all the even numbers from 0 to 10, including both 0 and 10. If we need all the odd numbers, we can change the starting value to 1 and run the code as below.
for (count = 1; count <= 10; count += 2) {
console.log(count);
}
It will give us all the even numbers within limits (1 to 10), including 1.
Output:
So, those are the primary applications of counting in JavaScript. Now, let’s try a different example.
Imagine, we need a counter that can be increased using a button. We can quickly achieve this goal with the counting concept using JavaScript with the help of HTML.
Firstly, we should build a button and a text box using the <input>
tag. The text box is to display the number, and the button increases the number upon the mouse click.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Counting</title>
</head>
<body>
<div>
<!-- Creating the text box -->
<input type="text" value="0" id="countVal" readonly>
<!-- Creating the increase button -->
<input type="button" value="+" onclick="increase()">
</div>
<script src="main.js"></script>
</body>
</html>
In the above code, we have to build a text box with countVal
as the ID and 0 as the starting value. Also, we made it uneditable using the read-only
attribute.
Then, we created a button to increase the value through the increase()
function upon the mouse click.
The above code displays the below result.
Output:
Now, we can implement the JavaScript code in a separate file to make the button interactive.
let count = 0;
let countValues = document.getElementById('countVal');
// The function to increase the count
function increase() {
count++;
countValues.value = count;
}
Firstly, we have declared a variable called count
to help the counting process. Then, we used the getElementById()
method to pass the values to the text box from the script file.
Afterward, the getElementById()
method has assigned to a variable called countValues
.
To make the button behave as we expected, we created an increase()
function. Every time a user clicks on the button, the value of the count is increased by one, and then assign that value to the value in the text box.
Now, by executing the code, we can have the below output.
Output:
The value of the count
variable increases, as in above, every time we click the button.
Let’s modify our work by adding another button to decrease the value and implementing a separate function.
<input type="button" value="-" onclick="decrease()">
We can add the above code inside the <div>
tag, and here is the complete HTML code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Counting</title>
</head>
<body>
<div>
<!-- Creating the text box -->
<input type="text" value="0" id="countVal" readonly>
<!-- Creating the increase button -->
<input type="button" value="+" onclick="increase()">
<!-- Creating the decrease button -->
<input type="button" value="-" onclick="decrease()">
</div>
<script src="main.js"></script>
</body>
</html>
Then, we can implement the JavaScript function to that button as follows.
function decrease() {
count--;
countValues.value = count;
}
Full JavaScript Code:
let count = 0;
let countValues = document.getElementById('countVal');
// The function to increase the count
function increase() {
count++;
countValues.value = count;
}
// The function to decrease the count
function decrease() {
count--;
countValues.value = count;
}
If we run the code, we will get our expected output below.
Output:
As in the above output, every time we click the decrease button, it decreases the number by one.
Conclusion
In this write-up, we discussed using JavaScript to apply the counting concept for several use cases. We used a for
loop to achieve them and got the expected outputs.
Also, we looked at a different use case where we used a text box and two buttons to increase and decrease the value upon the mouse clicks. We used HTML to build them and implemented a JavaScript code with two functions to make the buttons interactive.
We can apply the counting concept for various activities in many more methods.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.