JavaScript Async forEach
- Understanding JavaScript forEach
- Method 1: Using a Traditional for Loop
- Method 2: Using Promise.all with forEach
- Method 3: Using for…of with Async/Await
- Conclusion
- FAQ
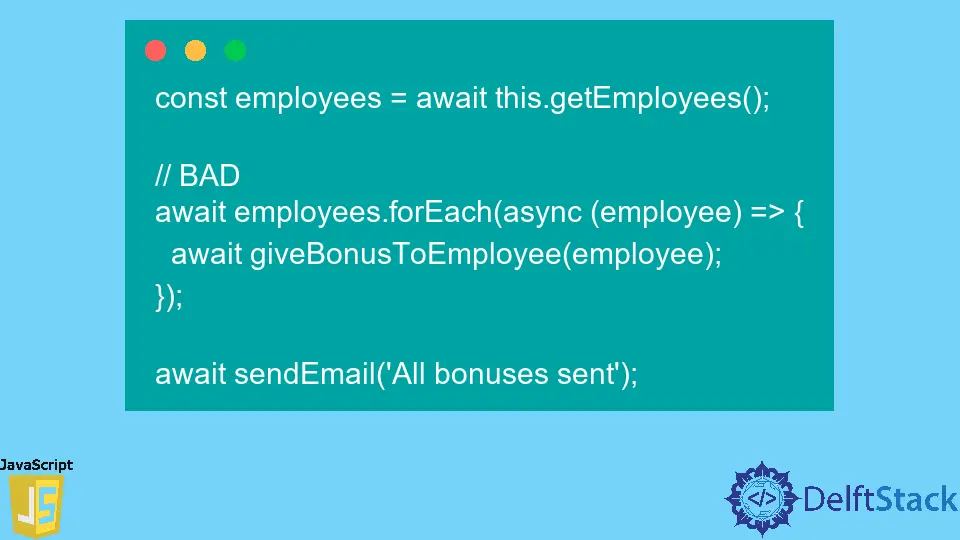
In today’s fast-paced web development landscape, handling asynchronous operations efficiently is crucial. JavaScript’s forEach
method is a staple for iterating over arrays, but it doesn’t natively support asynchronous functions. This limitation can be frustrating when you want to perform asynchronous tasks within a loop.
In this article, we’ll explore how to effectively use async
functions with forEach
, enabling you to manage asynchronous operations seamlessly. Whether you’re fetching data, performing calculations, or manipulating DOM elements, mastering this technique will elevate your JavaScript skills. Let’s dive into the world of asynchronous iteration and discover how to make the most of JavaScript’s capabilities.
Understanding JavaScript forEach
The forEach
method in JavaScript is a powerful tool for iterating over arrays. It executes a provided function once for each array element. However, when you introduce asynchronous functions into the mix, you might encounter unexpected behavior. The forEach
method does not wait for asynchronous operations to complete before moving on to the next iteration. This can lead to issues, especially when order of execution matters.
To illustrate, consider the following example where we want to fetch data from an API for each item in an array. Using forEach
with an async function won’t yield the expected results. Let’s take a look at how we can work around this limitation.
Method 1: Using a Traditional for Loop
One of the simplest ways to handle asynchronous operations in a loop is to use a traditional for
loop. Unlike forEach
, a for
loop will wait for each iteration to complete before moving on to the next one. Here’s how you can implement it:
async function fetchData(urls) {
for (let i = 0; i < urls.length; i++) {
const response = await fetch(urls[i]);
const data = await response.json();
console.log(data);
}
}
const urls = ['https://api.example1.com', 'https://api.example2.com'];
fetchData(urls);
In this example, we define an async function fetchData
that takes an array of URLs. By using a traditional for
loop, we await the response for each URL before proceeding to the next one. This ensures that the API requests are made in sequence.
Output:
{...data from api.example1.com...}
{...data from api.example2.com...}
This method is straightforward and effective for scenarios where the order of operations is critical. However, it may not be the most efficient approach when dealing with a large number of asynchronous tasks, as it processes them sequentially.
Method 2: Using Promise.all with forEach
If you want to execute multiple asynchronous operations concurrently, you can leverage Promise.all
in combination with map
instead of forEach
. This allows you to initiate all requests simultaneously and wait for all of them to complete. Here’s how you can do it:
async function fetchData(urls) {
const promises = urls.map(url => fetch(url).then(response => response.json()));
const results = await Promise.all(promises);
results.forEach(data => console.log(data));
}
const urls = ['https://api.example1.com', 'https://api.example2.com'];
fetchData(urls);
In this code, we use map
to create an array of promises, each representing an API request. Then we pass this array to Promise.all
, which resolves when all the promises are fulfilled. Finally, we iterate over the results and log the data.
Output:
{...data from api.example1.com...}
{...data from api.example2.com...}
This approach is more efficient for handling multiple asynchronous tasks, as it allows them to run concurrently. However, keep in mind that if any of the promises reject, Promise.all
will reject as well, so you may want to implement error handling depending on your use case.
Method 3: Using for…of with Async/Await
Another elegant solution is to use the for...of
loop, which works well with async/await. This method allows you to maintain the readability of your code while ensuring that each asynchronous operation completes before moving on to the next. Here’s an example:
async function fetchData(urls) {
for (const url of urls) {
const response = await fetch(url);
const data = await response.json();
console.log(data);
}
}
const urls = ['https://api.example1.com', 'https://api.example2.com'];
fetchData(urls);
In this example, we use for...of
to iterate over the array of URLs. Each fetch call is awaited, ensuring that the data is logged in the order the URLs were provided.
Output:
{...data from api.example1.com...}
{...data from api.example2.com...}
This method combines the benefits of sequential execution with the clarity of async/await syntax. It’s particularly useful when you need to perform operations in a specific order while still taking advantage of asynchronous programming.
Conclusion
Understanding how to effectively use async functions with JavaScript’s forEach
is essential for modern web development. By employing methods such as traditional loops, Promise.all
, and for...of
, you can manage asynchronous operations efficiently. Each approach has its own strengths and can be chosen based on the specific requirements of your project. With these techniques in your toolkit, you’ll be well-equipped to handle asynchronous tasks in your JavaScript applications, ensuring a smoother user experience and more robust code.
FAQ
-
What is the main limitation of using async functions with forEach?
The main limitation is that forEach does not wait for async operations to complete, leading to potential issues with the order of execution. -
How can I execute multiple async operations concurrently in JavaScript?
You can use Promise.all in combination with map to initiate all async operations at once and wait for their completion. -
Is it better to use a for loop or forEach for async operations?
A for loop is better for sequential execution, while forEach is more suitable for non-async tasks. For async tasks, consider using for…of or Promise.all. -
Can I use async/await with forEach?
No, forEach does not work with async/await as it does not return a promise. Instead, use for…of or Promise.all. -
What happens if one of the promises in Promise.all rejects?
If any promise in Promise.all rejects, the entire Promise.all will reject, and you should implement error handling to manage this scenario.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn