How to Wait for Promises to Get Resolved in JavaScript
- What Are Promises in JavaScript
- Stages of Promise in JavaScript
-
Implementing Promises Using
async
andawait
Keywords in JavaScript
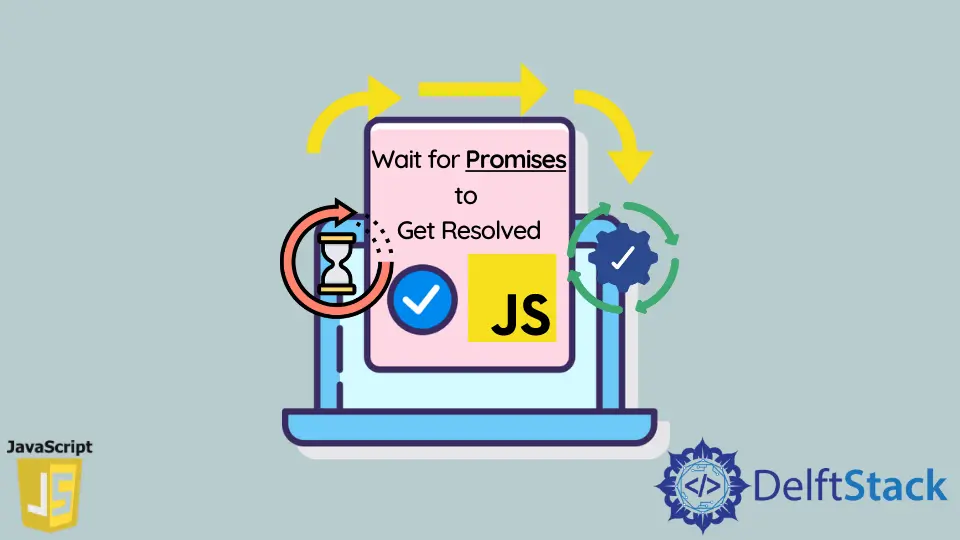
In the ECMA Script (ES6) version, most of the modern features of the JavaScript programming language were introduced. The features of this language, one of the most prominent and widely used features of JavaScript, called promises, was also introduced in this version.
As we know, the JavaScript language is a single-threaded language meaning only one task can be performed and in a specific order. In some cases, this can create a problem.
For example, if you have used a setTimeout()
function, executed after three seconds, the JavaScript compiler will not wait for 3 seconds. As shown below, it will just start executing the next part of the code.
function abc() {
console.log('a');
setTimeout(() => {
console.log('b');
}, 3000)
console.log('c');
}
abc();
Output:
a
c
b
Here, b
must have been printed before c
, but that’s not the case. So, to overcome this, the promises were introduced in JavaScript, which helps us perform an asynchronous task.
Let’s see how promises work and wait until promises are resolved in the background. Also, most of the concepts we will be learning in this article are supported by the JavaScript ES6 version and above, and it may not work in older browsers like IE.
What Are Promises in JavaScript
Whenever we perform any request like a database request or an API request, it may take a while to get the data back from the server. We can’t say how much time such operations can take in production where many requests are performed simultaneously.
Promises in JavaScript allow us to wait for such operations or tasks to complete their execution, and based on whether the task is fulfilled, we can take further actions.
We must create a Promise
class object using the new
keyword to use promises. Promise takes two arguments, resolve and reject.
The resolve()
method returns a promise object that is resolved with a value. If there is an error while executing a promise, the reject method will return an error.
Promises use async
and await
keywords. These keywords are important and necessary while implementing promises.
We will learn more about them later in this article.
Stages of Promise in JavaScript
An analogy to Promises could be, say you and your friend promises to each other that you both will go to watch a movie together on Sunday. And assume that its Friday and the Promise is made today.
Since Sunday has not come yet, we can technically say that the Promise is pending. If you meet your friend on Sunday, the Promise is fulfilled, and if you break your Promise by not going to watch the movie, then the Promise is rejected.
Promises in JavaScript are performed in 3 stages, and they are as follows.
- Pending: When a promise is being executed, it is said to be in the pending stage.
- Fulfilled: When a promise completes its execution successfully, it gets resolved and returns the value of the operation performed. This is where the Promise is said to be in fulfilled stage.
- Rejected: If the Promise fails during its execution, it gets rejected and returns an error message. This is where the Promise is said to be in rejected stage.
Implementing Promises Using async
and await
Keywords in JavaScript
The async
and await
keywords go hand in hand while implementing promises.
The async
keyword can be used with a function that you think can take time to execute. This makes them that function asynchronous.
This keyword tells the JavaScript engine that some part of the code within that function could take some time to execute, and you need to wait till it completes its execution.
We must specify which part of the code within that function could take some time to execute. This is where we use the await
keyword.
So, wherever you use an await
keyword within that function, the JavaScript engine will wait until that part is completely executed. If the execution is successful, the fulfilled Promise will be returned, or the Promise will be rejected.
The below example illustrates this.
Here, we have a function called myPromise
. We have created a Promise
inside this function, either resolved or rejected.
The job of this function is to fetch the JSON data from the API. Since we are requesting data from the API, the Promise
could take some time to execute, so we have used await
to wait for the Promise to resolve.
As we use await
before returning a Promise
, we must make the myPromise
function as async
.
The API we have used is a free API from the JSONPlaceholder website. Using this API, we will fetch the posts data.
We can use the fetch()
function to fetch the data from this API. After this function fetches the data, we must specify what we want to do next using the then()
function.
We simply return the data from the fetch()
function to the then()
function as a parameter. The parameter’s name could be anything, and we have given response
as the parameter name.
Finally, we will return the resolve the Promise by converting the result into JSON format using the response.json()
function.
async function myPromise() {
return await new Promise((resolve, reject) => {
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => {
resolve(response.json());
})
.catch(error => {
reject(error);
});
})
}
If we face some error while fetching the data from the API, that will be handled by the catch()
function. This function will return the error with the help of the reject()
function.
After the Promise is resolved, you can do whatever you want next with the data, which it returns with the help of the then()
function. You can have multiple then()
functions, which will be executed in a sequence one after the other.
And if you want to catch errors, you can use the catch()
function.
myPromise().then(result => {
result.forEach(element => {
var heading = document.createElement('h2');
heading.innerHTML = element.title;
document.body.appendChild(heading);
});
});
Output:
After the myPromise()
function returns a resolved promise, we use the then()
function to get the posts’ title and show it on the HTML document.
Here, we have created an h2
heading tag, and we are appending the titles present inside the result data we received from the posts API to the body
of the HTML document.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn