How to Decode HTML Entities Using JavaScript
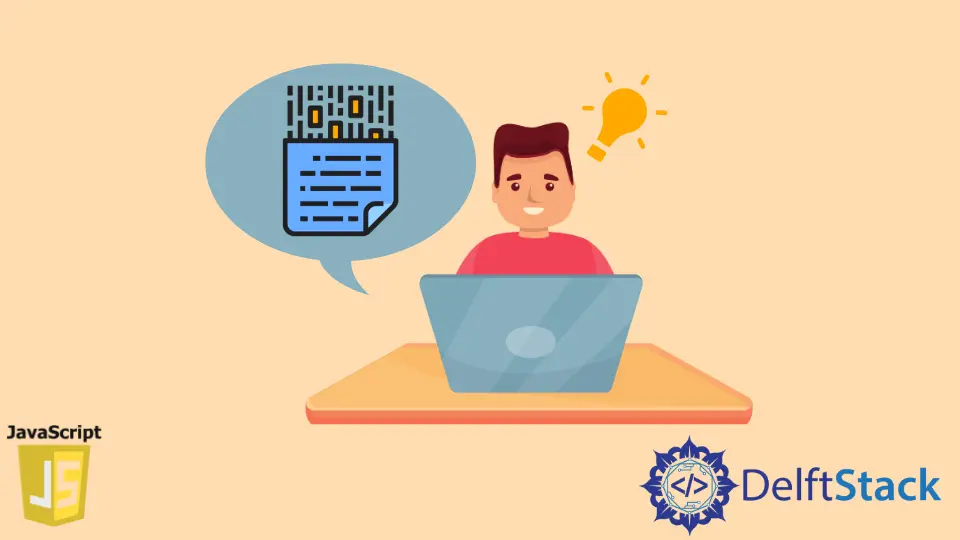
HTML entities are a set of character codes used to represent characters reserved by HTML. It starts with ampersand &
and ends with a semicolon ;
. This image provides you with a list of HTML character codes with their respective entity name.
This article will look at how to decode the below string using JavaScript. This string contains various characters like a
, b
, c
, and HTML character entities like &nbps;
, <
, '
, etc.
var str = 'Give us some'<h2>time</h2>' & space <br> Please';
The entire string is stored inside the str
variable. There are two ways to decode HTML entities. One way is by using vanilla JavaScript, and the other is by using an external library.
The external library we will be using is the he
library. This is one of the most commonly used libraries for encoding and decoding HTML entities.
Decode HTML Entities Using Vanilla JavaScript
One of the simplest ways of decoding HTML entities is by using vanilla JavaScript. The tag used to achieve this is the textarea
.
First, we will create a function called decodeEntity()
, which takes a string as an input. We will pass the str
variable to this function as an argument.
Inside this function, we will first create a textarea
element using document.createElement()
and then storing the reference of this variable inside the textarea
variable.
function decodeEntity(inputStr) {
var textarea = document.createElement('textarea');
textarea.innerHTML = inputStr;
return textarea.value;
}
console.log(decodeEntity(str));
Output:
var str = "Give us some'<h2>time</h2>' & space <br> Please";
var he = require('he');
console.log(he.decode(str));
We will store the string str
inside the textarea
using the innerHTML
property. The browser automatically converts the str
back to its proper HTML format at this step. All the HTML entities will be decoded, and all the HTML tags will be retained.
Finally, using the textarea.value
, we will return the decoded string value.
Decode HTML Entities Using he
Library
The he
is an external library to encode and decode HTML entities written in JavaScript. This library can easily be downloaded by running the below command. The node.js
(any version) must be installed on your system for the below command to execute successfully.
To learn more about the he
library, visit this link.
npm install he
After we have installed the he
library, we can then import that library inside our JavaScript file using the require
function and pass the library’s name, he
, as a string to this function.
Then we will store the reference of that inside a variable called he
. You can use any name to represent this variable.
var str = 'Give us some'<h2>time</h2>' & space <br> Please';
var he = require('he');
he.decode(str);
The he
library has a function called decode()
which takes two parameters html
and options
.
The html
parameter is a string that consists of encoded HTML entities and is mandatory to pass. The options
parameter is optional and it takes two values isAttributeValue
and strict
. We don’t need this second parameter, so we will not use it.
We will pass the str
variable mentioned above to the he.decode()
function, and the resulting output will be as follows.
Give us some '<h2>time</h2>'&space<br>Please
It is easy to decode HTML entities using an external JavaScript library.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedInRelated Article - JavaScript Promises
- How to Wait for Promises to Get Resolved in JavaScript
- How to Wait for a Function to Finish in JavaScript