How to Decode URL in JavaScript
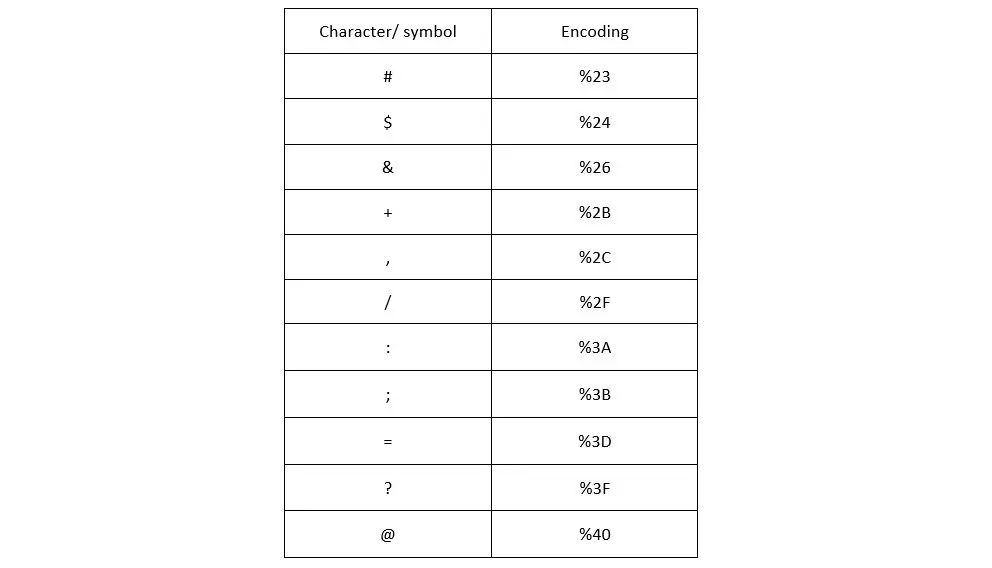
This article looks at URL decoding and how to decode an encoded URL using JavaScript.
Need of URL Encoding and Decoding
There are certain characters that an URL should have. An URL can include US-ASCII
characters like numbers from 0 to 9 and characters from a-z, and some special characters.
But, some URLs can have characters or special characters that are not in US-ASCII. That is when we need URL encoding.
URL encoding enables us to have an URL in a proper format, in other words, acceptable form, even if it has special characters outside the US-ASCII table.
Refer to the below example.
http://example.us/url encoding
In the above URL, there is a gap/ space between the URL and encoding, which is not acceptable for a URL format. When encoding the URL, the gap will be replaced by some special characters, and the URL will be in the proper format.
We can use URL decoding to decode an encoded URL. This process is the reverse of URL encoding.
When we decode an URL, we can have the URL in a more readable way.
URL Decoding in JavaScript
In JavaScript, there are three methods to decode an encoded URL.
unescape()
methoddecodeURI()
methoddecodeURIComponent()
method
Use the unescaped()
Method to Decode an Encoded URL
This method is deprecated in JavaScript, which means that function is not used in JavaScript. Instead of using this function, we can use the other two options to decode an encoded URL.
Use the decodeURI()
Method to Decode an Encoded URL
In JavaScript, we have the encodeURI()
method to encode an URL and decodeURI()
to decode an encoded URL. The encodeURI()
method encodes the URL address without the domain-related parts of the address (HTTPS, domain name, etc.).
decodeURI()
is the reverse method of encodeURI()
, which decodes the encoded URL with regular characters. Below are the syntaxes for the decodeURI()
and encodeURI()
methods.
Syntax:
// Syntax for encoding
encodeURI(content to encode)
// Syntax for decoding
decodeURI(content to decode)
To see the decoding process, we need to have an encoded URL. Let’s take a sample URL.
http://example.us /url encoding
As the first step, we should assign the URL to a variable as a string below.
let exURL = ' http://example.us /url encoding';
Then we encode it using the encodeURI()
method and print using the console.log()
method.
let encodedURL = encodeURI(exURL);
console.log(encodedURL);
In the above statement, we have assigned the encodeURI()
method to a variable called encodedURL
. In another way, we can directly use the encodeURI()
function inside the console.log()
function as below.
console.log(encodeURI(exURL));
Both ways give us the same result so let’s move with the first path. Now our complete JavaScript code should look like this.
Full Code:
let exURL = 'http://example.us /url encoding';
let encodedURL = encodeURI(exURL);
console.log(encodedURL);
Output:
Now let’s decode it using the decodeURI()
method. Firstly, we can assign the decodeURI()
process to another variable, as below.
let decodedURL = decodeURI(encodedURL);
console.log(decodedURL);
Output:
As you can see, we will get the decoded URL from the above process.
Use the decodeURIComponent()
Method to Decode an Encoded URL
The decodeURIComponent()
method is the reverse method of the encodeURIComponent()
method. The encodeURIComponent()
method encodes the URL address with the domain-related parts of it.
Also, it encodes a set of special characters, which the encodeURI()
method does not. Here is the unique character list.
The decodeURIComponent()
approach decodes the encoded URL with the relevant characters and symbols.
Syntax:
// Syntax for encoding
encodeURIComponent(content to encode)
// Syntax for decoding
decodeURIComponent(content to decode)
Let’s take an example URL.
https://stackoverflow.com/url+encoded string+in javascript
In the above example, there are spaces
, /
,:
, and +
marks within the URL. Let’s encode the URL using the encodeURIComponent()
method.
let exURL = 'https://stackoverflow.com/url+encoded string+in javascript';
let encoded = encodeURIComponent(exURL);
console.log(encoded);
Output:
Now let’s try to decode it using the decodeURIComponent()
method as below.
let decoded = decodeURIComponent(encoded);
console.log(decoded);
As in above, we have assigned the decodeURIComponent()
method to a variable. Print it using the console.log()
method.
Output:
As you can see, the URL we encoded has been given as the decoded URL.
Full code:
let exURL = 'https://stackoverflow.com/url+encoded string+in javascript';
let encoded = encodeURIComponent(exURL);
console.log(encoded);
let decoded = decodeURIComponent(encoded);
console.log(decoded);
Conclusion
This article briefly introduced JavaScript and taught what URL encoding and decoding are. Then we mainly discussed two methods: decodeURI()
and decodeURIComponent()
, along with some examples.
Both methods work in two ways to use the suitable method according to the requirement. The unescape()
method has been deprecated recently, and instead of it, we can use the other two methods as solutions.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.