How to Filter Object in JavaScript
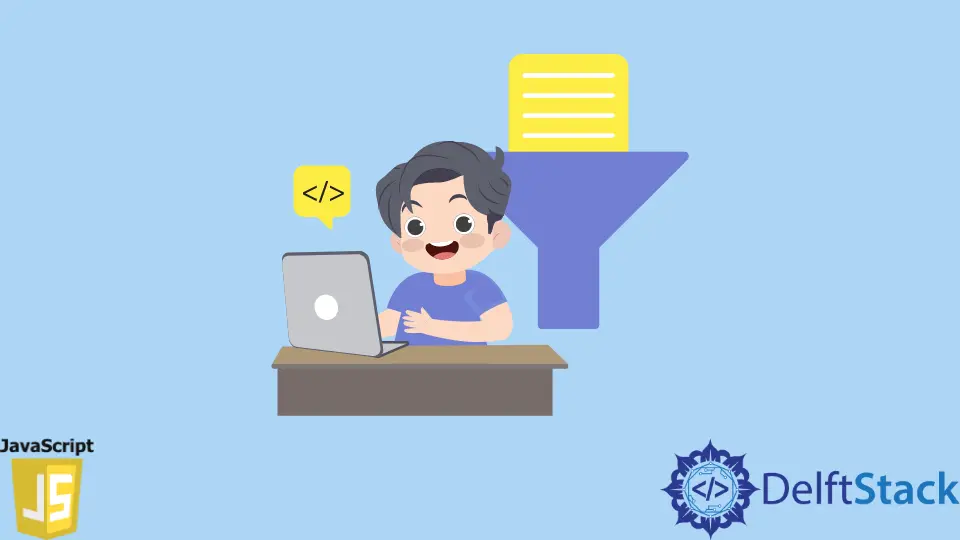
This tutorial will introduce how to filter an object in JavaScript. We will learn how to implement a similar filter
method as in the array data type to the Object
in JavaScript.
Use reduce
to Filter Object in JavaScript
Let’s now implement the function to filter objects using the reduce
function.
Suppose we have the following object:
var grades = {linearAlgebra: 90, chemistry: 95
biology: 90
languages : 96
}
The above object represents a student’s grades; we need to filter that object and only get the subjects with a grade of 95 or more.
var grades = {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96};
Object.filter =
function(mainObject, filterFunction) {
return Object.keys(mainObject)
.filter(function(ObjectKey) {
return filterFunction(mainObject[ObjectKey])
})
.reduce(function(result, ObjectKey) {
result[ObjectKey] = mainObject[ObjectKey];
return result;
}, {});
}
console.log('The grades are ', grades);
var targetSubjects = Object.filter(grades, function(grade) {
return grade >= 95;
});
console.log('Target Subjects are ', targetSubjects);
Output:
"The grades are ", {
biology: 90,
chemistry: 95,
languages: 96,
linearAlgebra: 90
}
"Target Subjects are ", {
chemistry: 95,
languages: 96
}
We implement the Object.filter
function as Object.filter = function(mainObject, filterFunction)
in the above example. We can simplify that example code as below.
Object.filter = function(mainObject, filterFunction) {
return Object.keys(mainObject)
.filter(innerFilterFunction)
.reduce(reduceFunction, {});
}
It has 3 main steps to implement that Object.filter
function:
Object.keys()
returns an array of keys from thekey-value
pairs in the object. It islinearAlgebra, chemistry, biology, languages
in our example.- The result of the
Object.keys()
is sent to the callback functioninnerFilterFunction
, which is also an argument of the filter function, and the output of thatinnerFilterFunction
function will be the filtered keys. The output ischemistry, languages
in our example. - The result of the filter is now passed to our
reduce()
function, which adds each value to its key, and returns a new object containing all of those pairs. So the result in our example is{chemistry: 95, languages: 96}
.
If we want to recreate the above example with the arrow
syntax of ES6, we could rewrite it as below.
var grades = {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96};
Object.filter = (mainObject, filterFunction) =>
Object.keys(mainObject)
.filter((ObjectKey) => filterFunction(mainObject[ObjectKey]))
.reduce(
(result, ObjectKey) =>
(result[ObjectKey] = mainObject[ObjectKey], result),
{});
console.log('The grades are ', grades);
var targetSubjects = Object.filter(grades, (grade) => grade >= 95);
console.log('Target Subjects are ', targetSubjects);
Output:
The grades are {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96}
Target Subjects are {chemistry: 95, languages: 96}
It uses the comma
operator to return the result
object, and it can also be replaced with Object.assign
, which returns the result as well. So the code can be rewritten as follows.
Object.filter = (mainObject, filterFunction) =>
Object.keys(mainObject)
.filter((ObjectKey) => filterFunction(mainObject[ObjectKey]))
.reduce(
(result, ObjectKey) =>
Object.assign(result, {[ObjectKey]: mainObject[ObjectKey]}),
{});
Use the map
Function to Filter JavaScript Objects
As we use the reduce
function in the above solution, we can also use the map
function to filter JavaScript objects.
Object.filter = function(mainObject, filterFunction) {
return Object.assign(...Object.keys(mainObject)
.filter(function(ObjectKey) {
return filterFunction(mainObject[ObjectKey])
})
.map(function(ObjectKey) {
return {[ObjectKey]: mainObject[ObjectKey]};
}));
}
We replaced the reduce
function with the map
function as shown above, and also used the Object.assign
and sent to it using the spread
syntax all the operations starting from Object.keys()
to .map()
, so the full Implementation should now be like the following:
var grades = {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96};
Object.filter =
function(mainObject, filterFunction) {
return Object.assign(...Object.keys(mainObject)
.filter(function(ObjectKey) {
return filterFunction(mainObject[ObjectKey])
})
.map(function(ObjectKey) {
return {[ObjectKey]: mainObject[ObjectKey]};
}));
}
console.log('The grades are ', grades);
var targetSubjects = Object.filter(grades, (grade) => grade >= 95);
console.log('Target Subjects are ', targetSubjects);
Output:
The grades are {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96}
Target Subjects are {chemistry: 95, languages: 96}