JavaScript의 필터 개체
Moataz Farid
2023년10월12일
JavaScript
JavaScript Filter
JavaScript Object
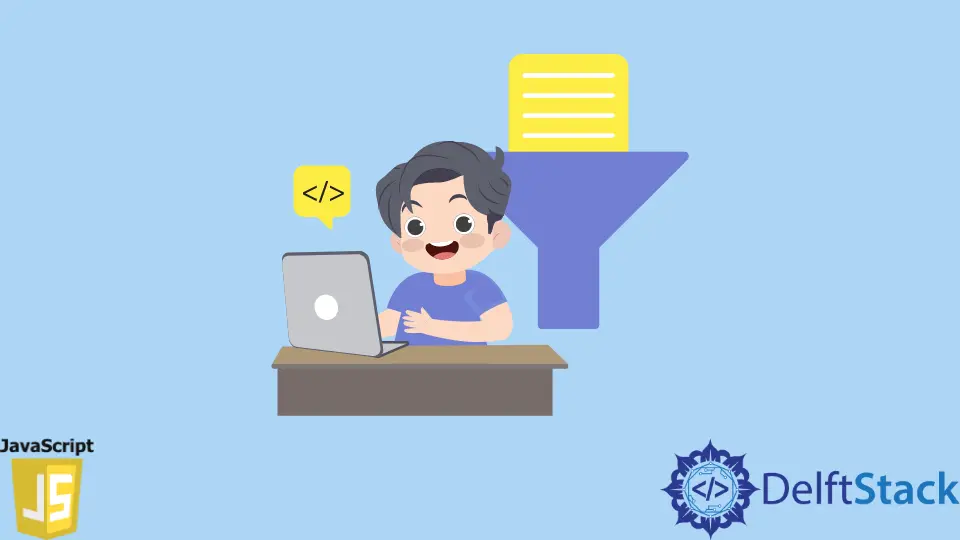
이 튜토리얼은 JavaScript에서 객체를 필터링하는 방법을 소개합니다. JavaScript의Object
에 배열 데이터 유형에서와 유사한filter
메소드를 구현하는 방법을 배웁니다.
reduce
를 사용하여 JavaScript에서 개체 필터링
이제reduce
함수를 사용하여 객체를 필터링하는 함수를 구현해 보겠습니다.
다음 객체가 있다고 가정합니다.
var grades = {linearAlgebra: 90, chemistry: 95
biology: 90
languages : 96
}
위의 개체는 학생의 성적을 나타냅니다. 우리는 그 개체를 필터링하고 95 이상의 등급을 가진 과목 만 가져와야합니다.
var grades = {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96};
Object.filter =
function(mainObject, filterFunction) {
return Object.keys(mainObject)
.filter(function(ObjectKey) {
return filterFunction(mainObject[ObjectKey])
})
.reduce(function(result, ObjectKey) {
result[ObjectKey] = mainObject[ObjectKey];
return result;
}, {});
}
console.log('The grades are ', grades);
var targetSubjects = Object.filter(grades, function(grade) {
return grade >= 95;
});
console.log('Target Subjects are ', targetSubjects);
출력:
"The grades are ", {
biology: 90,
chemistry: 95,
languages: 96,
linearAlgebra: 90
}
"Target Subjects are ", {
chemistry: 95,
languages: 96
}
위 예제에서는Object.filter
함수를Object.filter = function(mainObject, filterFunction)
로 구현합니다. 이 예제 코드를 아래와 같이 단순화 할 수 있습니다.
Object.filter = function(mainObject, filterFunction) {
return Object.keys(mainObject)
.filter(innerFilterFunction)
.reduce(reduceFunction, {});
}
Object.filter
함수를 구현하는 3 가지 주요 단계가 있습니다.
Object.keys()
는 객체의key-value
쌍에서 키 배열을 반환합니다. 우리 예에서는linearAlgebra, chemistry, biology, languages
입니다.Object.keys()
의 결과는 필터 함수의 인수이기도 한 콜백 함수innerFilterFunction
으로 전송되며,innerFilterFunction
함수의 출력은 필터링 된 키가됩니다. 출력은이 예에서chemistry, languages
입니다.- 이제 필터의 결과가
reduce()
함수에 전달되어 각 값을 키에 추가하고 이러한 모든 쌍을 포함하는 새 객체를 반환합니다. 따라서 예제의 결과는{chemistry: 95, languages: 96}
입니다.
ES6의arrow
구문으로 위 예제를 다시 만들고 싶다면 아래와 같이 다시 작성할 수 있습니다.
var grades = {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96};
Object.filter = (mainObject, filterFunction) =>
Object.keys(mainObject)
.filter((ObjectKey) => filterFunction(mainObject[ObjectKey]))
.reduce(
(result, ObjectKey) =>
(result[ObjectKey] = mainObject[ObjectKey], result),
{});
console.log('The grades are ', grades);
var targetSubjects = Object.filter(grades, (grade) => grade >= 95);
console.log('Target Subjects are ', targetSubjects);
출력:
The grades are {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96}
Target Subjects are {chemistry: 95, languages: 96}
comma
연산자를 사용하여result
객체를 반환하고 결과를 반환하는Object.assign
으로 대체 할 수도 있습니다. 따라서 코드는 다음과 같이 다시 작성할 수 있습니다.
Object.filter = (mainObject, filterFunction) =>
Object.keys(mainObject)
.filter((ObjectKey) => filterFunction(mainObject[ObjectKey]))
.reduce(
(result, ObjectKey) =>
Object.assign(result, {[ObjectKey]: mainObject[ObjectKey]}),
{});
map
함수를 사용하여 JavaScript 객체 필터링
위의 솔루션에서reduce
함수를 사용하므로map
함수를 사용하여 JavaScript 객체를 필터링 할 수도 있습니다.
Object.filter = function(mainObject, filterFunction) {
return Object.assign(...Object.keys(mainObject)
.filter(function(ObjectKey) {
return filterFunction(mainObject[ObjectKey])
})
.map(function(ObjectKey) {
return {[ObjectKey]: mainObject[ObjectKey]};
}));
}
위와 같이reduce
함수를map
함수로 대체하고Object.assign
도 사용하고Object.keys()
부터까지 모든 작업을spread
구문을 사용하여 전송했습니다. map
이므로 전체 구현은 이제 다음과 같아야합니다.
var grades = {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96};
Object.filter =
function(mainObject, filterFunction) {
return Object.assign(...Object.keys(mainObject)
.filter(function(ObjectKey) {
return filterFunction(mainObject[ObjectKey])
})
.map(function(ObjectKey) {
return {[ObjectKey]: mainObject[ObjectKey]};
}));
}
console.log('The grades are ', grades);
var targetSubjects = Object.filter(grades, (grade) => grade >= 95);
console.log('Target Subjects are ', targetSubjects);
출력:
The grades are {linearAlgebra: 90, chemistry: 95, biology: 90, languages: 96}
Target Subjects are {chemistry: 95, languages: 96}
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다