How to Filter Multiple Conditions in JavaScript
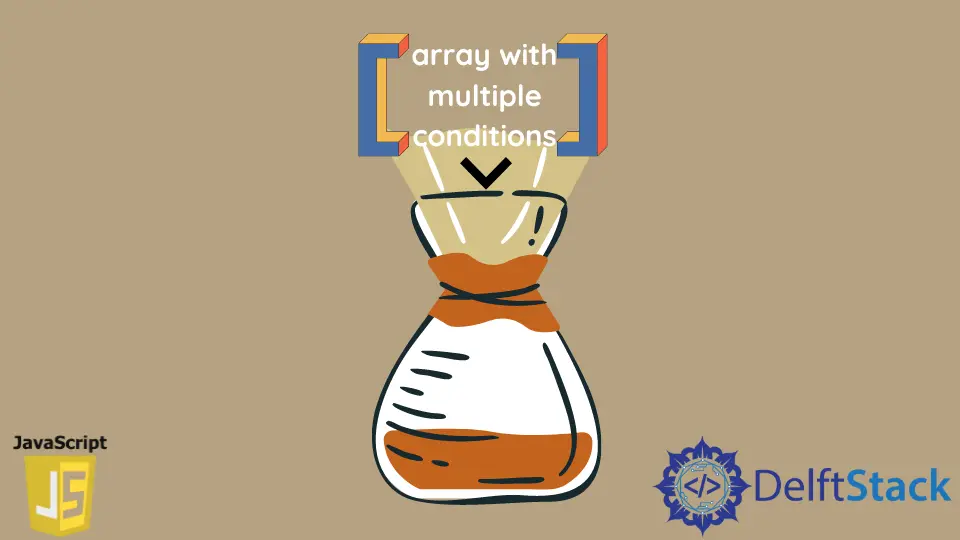
Today’s post teaches us to filter an array with multiple conditions using the traditional and filter
methods in JavaScript.
Filter Multiple Conditions in JavaScript
Arrays are a type of JavaScript object with a fixed numeric key and dynamic values. JavaScript provides several built-in methods to access and manipulate these array elements.
The filter()
method generates a new array from the original array with all elements that pass the condition/test implemented by the provided function.
Syntax:
filter(callbackFn)
The filter
method accepts callbackFn
as a parameter. This function is a predicate to test each element of the array.
Returns a new array that forces the element to remain true
or false
otherwise.
The callback function is called with the three arguments.
- An
element
is the current element processed in the array. - The
index
is the current element’s index being processed in the array. - The
array
on whichfilter()
was called.
A new array is returned, containing the elements that pass the test. An empty array will be returned if no elements pass the test.
filter()
calls a provided callbackFn
function once for each element of an array and constructs a new array of all values for which callbackFn
returns a value that enforces true.
callbackFn
is only called for array indexes having assigned values. It isn’t called for indexes that have been dropped or have never been assigned.
Array elements that fail the callbackFn
test are skipped and not included in the new array. Find more information in the documentation for the filter()
.
Use Traditional Method
const filter = {
address: 'India',
name: 'Aleena'
};
const users = [
{name: 'John Doe', email: 'johndoe@mail.com', age: 25, address: 'USA'},
{name: 'Aleena', email: 'aleena@mail.com', age: 35, address: 'India'},
{name: 'Mark Smith', email: 'marksmith@mail.com', age: 28, address: 'England'}
];
const filteredUsers = users.filter((item) => {
for (var key in filter) {
if (item[key] === undefined || item[key] != filter[key]) return false;
}
return true;
});
console.log(filteredUsers);
We have described a filter
object with the desired properties within the above example. We have defined the callback function in the filter
method to check if the desired properties are present in the specified array.
If the desired properties are not present, false
is returned; otherwise, true
is returned.
This approach is useful when the filter
object is dynamically generated. If the filter
object is static, you can filter the objects using the &&
condition below.
Attempt to run the above code snippet in any browser that supports JavaScript; it will display the result below.
Use the Filter
Method
const filteredUsers = users.filter(
obj => obj.name == filter.name && obj.address == filter.address);
console.log(filteredUsers);
Output:
[{
address: "India",
age: 35,
email: "aleena@mail.com",
name: "Aleena"
}]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn