How to Download a File Using JavaScript
-
Use
download
Attribute in HTML to Download Files - Using a Custom-Written Function to Create and Download Text Files in JavaScript
-
Use
Axios
Library to Download Files
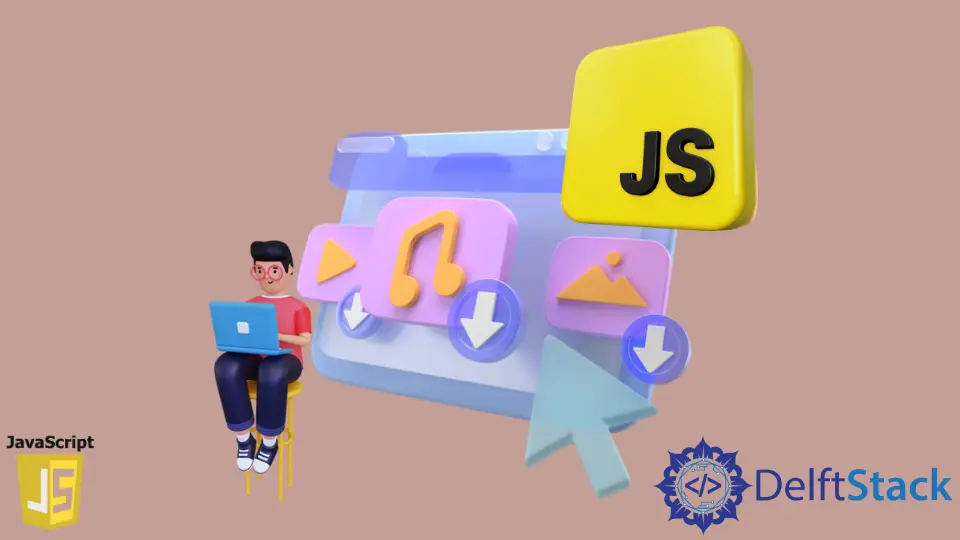
In this article, we will learn how to download files using JavaScript. Automatic downloading files help us retrieve files directly from the URL with a JavaScript function without contacting any servers. We will achieve this using our custom written functions and using the download attribute of HTML 5.
Use download
Attribute in HTML to Download Files
The download
attribute in HTML 5 is used to download files when users click on the hyperlink. It is used with anchor tags - <a>
and <area>
. We are required to set the href
attribute specifying the source of the file. The value of the download
attribute determines the name of the downloaded file. If this value is removed, then the downloaded filename will be the same as the original file name.
<!DOCTYPE html>
<html>
<head>
<title>How to Download files Using JavaScript </title>
</head>
<body>
<a href="apple.png" download="apple">
<button type="button">Download</button>
</a>
</body>
</html>
In the above code, we download an image apple.png
using the download
attribute. We first create the anchor tag containing the image’s address and add the download
attribute to it. Then we also created a download button to facilitate downloading files.
Using a Custom-Written Function to Create and Download Text Files in JavaScript
This approach will create text data on the fly and then use JavaScript to create a text file and then download it.
Download Algorithm
-
Create a text area to enter the text data.
-
Create an anchor tag
<a>
using thecreateElement
property and assigndownload
andhref
attributes to it. -
Use the
encodeURIComponent
to encode the text and append it to URI as its component. This will help us to replace certain special characters with a combination of escape sequences. -
Set the date type to
text/plain
and encoding toUTF-8
using thedata:text/plain; charset = utf-8
as the attribute value ofhref
. -
Append this created element to the body of the document(HTML page).
-
Use
element.click()
to simulate a mouse click. -
Remove the element from the body of the document(HTML page).
Attach an event listener looking for a click to a download button. Call the download function with text from the text area and the text file’s filename as parameters.
<!DOCTYPE html>
<html>
<head>
<title>
How to Download files Using JavaScript
</title>
</head>
<body>
<textarea id="text">DelftStack</textarea>
<br />
<input type="button" id="btn" value="Download" />
<script>
function download(filename, textInput) {
var element = document.createElement('a');
element.setAttribute('href','data:text/plain;charset=utf-8, ' + encodeURIComponent(textInput));
element.setAttribute('download', filename);
document.body.appendChild(element);
element.click();
//document.body.removeChild(element);
}
document.getElementById("btn")
.addEventListener("click", function () {
var text = document.getElementById("text").value;
var filename = "output.txt";
download(filename, text);
}, false);
</script>
</body>
</html>
Use Axios
Library to Download Files
In this approach, we will use the Axios
library to download files. Before proceeding with the approach’s details, let us understand what Blob
is, the data type used to download files using Axios.
Blob
Blob
stands for Binary Large Object
and is a data type that can store binary data. It represents data like programs, code snippets, multimedia objects, and other things that don’t support JavaScript native format.
Download Files
-
Create an Axios
get
request with URL as the source of the file and theresponseType
as ablob
. -
Resolve the promise returned by the Axios request by performing the following steps:
- Create a DOMString that contains the URL representing the Blob object.
- Create an anchor tag
<a>
using thecreateElement
property and assigndownload
andhref
attributes to it. - Set
href
as the URL created in the first step anddownload
attribute as the downloaded file’s name. - Attach this link to the document and simulate a click using the
.click()
method. - Remove this link from the document.
<!DOCTYPE html>
<html>
<head>
<title>How to download files using JavaScript</title>
</head>
<body>
<button onclick="download()">
Download Image
</button>
<script src="https://cdnjs.cloudflare.com/ajax/libs/axios/0.19.2/axios.min.js">
</script>
<script>
function download() {
axios({
url: 'https://source.unsplash.com/random/500x500',
method: 'GET',
responseType: 'blob'
})
.then((response) => {
const url = window.URL
.createObjectURL(new Blob([response.data]));
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', 'image.jpg');
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
})
}
</script>
</body>
</html>
Here we get random images from a site, use Axios
to request those images in the form of blobs, and then download them using the anchor tag’s download
attribute. This method is not restricted to the plain text entered by the user like the previous method. We can request any sort of data from an API and then use this approach to save data inside our computer.
All the major browsers support all the above methods except the method using the Axios
library. Internet Explorer still does not supports the native ES6 promises, and Axios depends heavily on them.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn