使用 JavaScript 下载文件
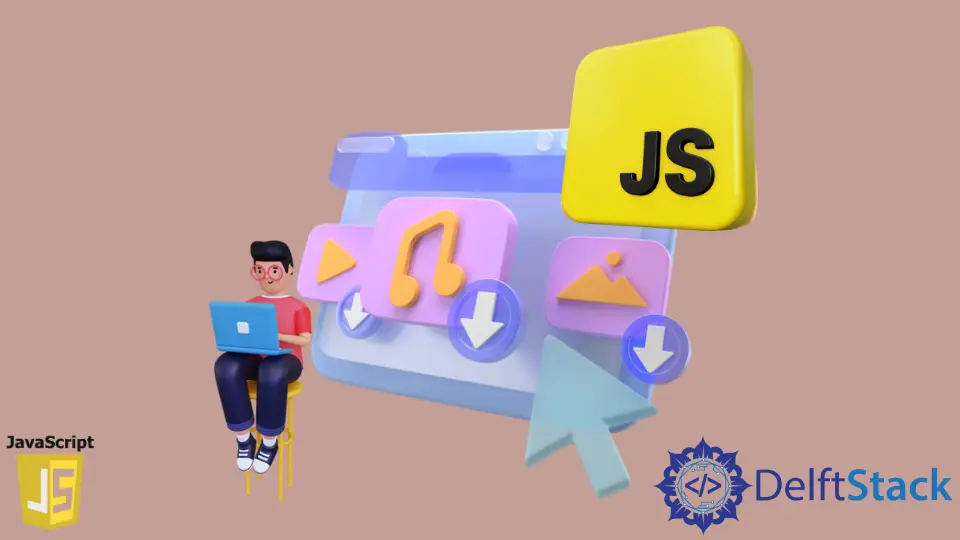
在本文中,我们将学习如何使用 JavaScript 下载文件。自动下载文件可帮助我们使用 JavaScript 函数直接从 URL 检索文件,而无需联系任何服务器。我们将使用自定义的编写函数并使用 HTML 5 的下载属性来实现此目的。
使用 HTML 中的 download
属性下载文件
当用户单击超链接时,HTML 5 中的 download
属性用于下载文件。它与锚标签-<a>
和 <area>
一起使用。我们需要设置 href
属性,以指定文件的来源。download
属性的值确定下载文件的名称。如果删除此值,则下载的文件名将与原始文件名相同。
<!DOCTYPE html>
<html>
<head>
<title>How to Download files Using JavaScript </title>
</head>
<body>
<a href="apple.png" download="apple">
<button type="button">Download</button>
</a>
</body>
</html>
在上面的代码中,我们使用 download
属性下载图像 apple.png
。我们首先创建包含图像地址的锚标签,然后向其添加 download
属性。然后,我们还创建了一个下载按钮,以方便下载文件。
在 JavaScript 中使用自定义函数创建和下载文本文件
这种方法将动态创建文本数据,然后使用 JavaScript 创建文本文件然后下载。
下载算法
-
创建一个文本区域以输入文本数据。
-
使用
createElement
属性创建锚标签<a>
,并为其分配download
和href
属性。 -
使用
encodeURIComponent
编码文本并将其附加到 URI 作为其组成部分。这将帮助我们用转义序列的组合替换某些特殊字符。 -
将日期类型设置为
text/plain
,并使用data:text/plain; charset = utf-8
作为href
的属性值。 -
将此创建的元素追加到文档(HTML 页面)的正文中。
-
使用
element.click()
模拟鼠标单击。 -
从文档正文(HTML 页面)中删除元素。
附加事件侦听器,以查找单击下载按钮的过程。使用文本区域中的文本和文本文件的文件名作为参数来调用下载功能。
<!DOCTYPE html>
<html>
<head>
<title>
How to Download files Using JavaScript
</title>
</head>
<body>
<textarea id="text">DelftStack</textarea>
<br />
<input type="button" id="btn" value="Download" />
<script>
function download(filename, textInput) {
var element = document.createElement('a');
element.setAttribute('href','data:text/plain;charset=utf-8, ' + encodeURIComponent(textInput));
element.setAttribute('download', filename);
document.body.appendChild(element);
element.click();
//document.body.removeChild(element);
}
document.getElementById("btn")
.addEventListener("click", function () {
var text = document.getElementById("text").value;
var filename = "output.txt";
download(filename, text);
}, false);
</script>
</body>
</html>
使用 Axios
库下载文件
在这种方法中,我们将使用 Axios
库下载文件。在继续介绍该方法的详细信息之前,让我们了解什么是 Blob
,这是用于使用 Axios 下载文件的数据类型。
Blob
Blob
代表 Binary Large Object
,是一种可以存储二进制数据的数据类型。它表示程序、代码片段、多媒体对象之类的数据,以及不支持 JavaScript 本机格式的其他内容。
下载文件
-
创建一个 Axios
get
请求,其中 URL 为文件源,而responseType
为blob
。 -
通过执行以下步骤来解决 Axios 请求返回的承诺:
- 创建一个包含代表 Blob 对象的 URL 的 DOMString。
- 使用
createElement
属性创建锚标签<a>
,并为其分配download
和href
属性。 - 将
href
设置为第一步中创建的 URL,将download
属性设置为下载文件的名称。 - 将此链接附加到文档,并使用
.click()
方法模拟点击。 - 从文档中删除此链接。
<!DOCTYPE html>
<html>
<head>
<title>How to download files using JavaScript</title>
</head>
<body>
<button onclick="download()">
Download Image
</button>
<script src="https://cdnjs.cloudflare.com/ajax/libs/axios/0.19.2/axios.min.js">
</script>
<script>
function download() {
axios({
url: 'https://source.unsplash.com/random/500x500',
method: 'GET',
responseType: 'blob'
})
.then((response) => {
const url = window.URL
.createObjectURL(new Blob([response.data]));
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', 'image.jpg');
document.body.appendChild(link);
link.click();
document.body.removeChild(link);
})
}
</script>
</body>
</html>
在这里,我们从一个网站获得随机的图片,使用 Axios
来请求这些图片的 blob 形式,然后使用锚标签的 download
属性下载它们。与以前的方法一样,此方法不限于用户输入的纯文本。我们可以从 API 请求任何种类的数据,然后使用这种方法将数据保存在计算机内部。
除使用 Axios
库的方法外,所有主流浏览器均支持上述所有方法。Internet Explorer 仍然不支持本机 ES6 承诺,而 Axios 严重依赖它们。
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn