How to Auto Click in JavaScript
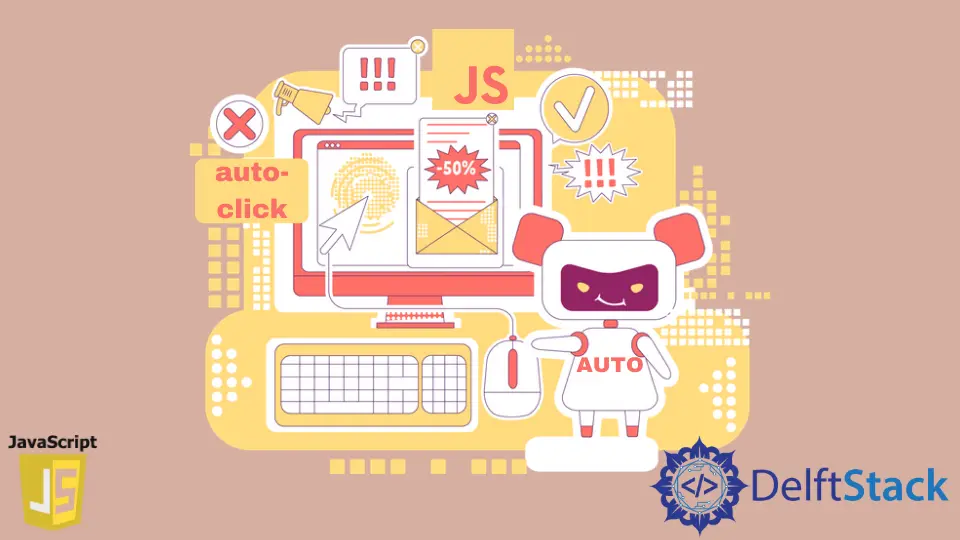
The window
object is one of the most important objects for communicating with the browser; it represents the browser window. All functions and global variables become members of the window
object.
The WindowLocation
object is used to get the URL of the current page and also change the URL of the redirect.
This article teaches how to automatically trigger a click event of anchor tag in JavaScript. This can be achieved via the location
attribute or the open
method of the window
object.
Use window.open()
to Auto Click in JavaScript
The window.open()
is a JavaScript-provided window interface method that loads the specified URL/resource into a new tab or existing browser with the specified name. This method creates a new window used to open a specific URL.
Every time the window.open()
method returns, it contains about:blank
. Once the current script block has been executed, the actual URL will be loaded.
Syntax:
window.open(url, windowName, windowFeatures);
This method takes url
as an input parameter which is a required parameter that accepts valid image paths, URLs, or other browser-supported resources. If you pass an empty string, a new tab will open with an empty URL.
The windowName
is an optional parameter that specifies the name of the browser context. This does not determine the window title, and also, the name of this window cannot contain spaces.
The windowFeatures
is an optional parameter. This parameter accepts comma-separated window properties of a new tab in the form name=value
or name
if the property is Boolean.
Some of the options are the default position and size of the object window.
You can find more information in the documentation for the method Window.location
.
<a class="myLink" id="gle-lnk" href="https://google.com">Open Google</a>
const link = document.getElementById('gle-lnk');
window.open(link.href);
In the above code, we use the open
method of the window
object, which will open the requested URL in the new tab.
Use window.location
to Auto Click in JavaScript
This is a read-only property of Window.location
. This returns the Location
object.
All the information related to the document’s current location is stored in this object. This location object also contains href
, protocol
, host
, hostname
, port
, etc.
You can also access the window.location
properties directly using location
since the widget always stays at the top of the scope chain. Users can use the href
property or the Location
object’s assign
method to load/open another URL/resource.
Syntax:
window.location = URL_PATH;
window.location.assign(URL_PATH);
window.location.href = URL_PATH;
URL_PATH
is a required parameter that accepts a valid URL to be opened. This URL can be any URL, absolute URL, anchor URL, relative URL, or new protocol.
<a class="myLink" id="gle-lnk" href="https://google.com">Open Google</a>
const link = document.getElementById('gle-lnk');
window.location = link.href;
window.location.href = link.href;
In the above code, we use the location
property of the window
object, which will change the requested URL (specified in the anchor tag) with the existing URL in the same tab.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn