How to Initialize JavaScript Date to a Particular Time Zone
-
Use
Intl.DateTimeFormat
andformat()
Methods to Initialize JavaScript Date to a Particular Time Zone -
Use the
toLocaleString()
Method to Initialize JavaScript Date to a Particular Time Zone
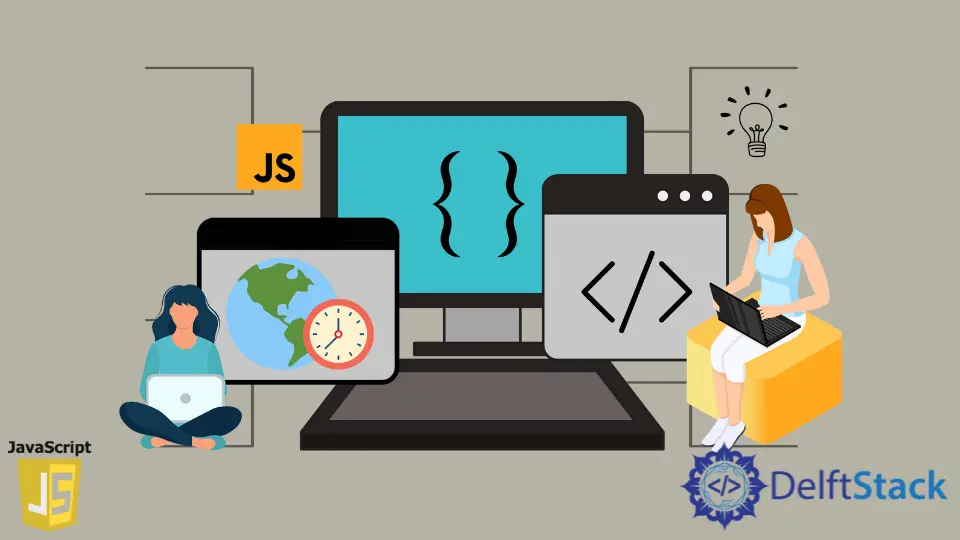
This tutorial teaches how to initialize JavaScript date to a particular time zone.
JavaScript’s date object works with UTC internally but displays output according to the local time of the operating computer. The date object doesn’t have any notion of time zones. There are no string objects declared. It is just a measurement of the number of milliseconds that have elapsed since 1970-01-01 00:00:00 UTC
. While outputting the time, it automatically takes into account the computer’s local time zone and applies to the internal representation. It doesn’t work with different time zones. So, we need the help of functions and external libraries to perform such operations.
Use Intl.DateTimeFormat
and format()
Methods to Initialize JavaScript Date to a Particular Time Zone
JavaScript Intl object is the aid for JavaScript Internationalization API. It provides us with many functions for the conversion of date/time, strings, and numbers. The Intl object uses the DateTimeFormat
to format date-time strings. This has a format
method that takes the date and converts it to different time zone using the locale and options provided as an argument to the formatter. This method will format the date to the required time zone and convert it into a string.
The parameter taken by the format()
function is the date. We require the following arguments for the formation of Intl.DateTimeFormat
object:
locales
: It is an array of strings that contain language and locale tags. Normally, it is an optional parameter but it is necessary for our purpose. To change the time zone we can just specify the BCP language code and the required time zone.options
: It is an object used to specify properties to perform comparisons. It is also an optional parameter that can be used to specify the style and display units. Some of the properties aresecond
,minute
,hour
,day
,month
andyear
, etc.
For example: We can pass an options object like { hour: 'numeric', hour12: false, minute: 'numeric', timeZoneName: 'short'}
function changeTimezone() {
let date = new Date(Date.UTC(2021, 5, 28, 3, 0, 0));
console.log('Date in India: ' + date);
let formatter =
new Intl.DateTimeFormat('en-US', {timeZone: 'America/Denver'});
let usDate = formatter.format(date);
console.log('Date in USA: ' + usDate);
}
Output:
Date in India: Mon Jun 28 2021 08:30:00 GMT+0530 (India Standard Time)
VM1504:7 Date in USA: 6/27/2021
In the above function, we first create a Date object using the Date()
constructor. Then we go and create a formatter using Intl.DateTimeFormat
specifying the locale i.e the BCP language tag
and the timeZone
to which we want to convert. We then use this formatter to convert the date to the required timezone.
Use the toLocaleString()
Method to Initialize JavaScript Date to a Particular Time Zone
The toLocaleString()
method is the most commonly used for changing time zone as it is easier to use and can be called directly on a Date. It works in the same sway as the Intl.DateTimeFormat
. It also takes the locale string and options as arguments and returns a string with dates formatted according to them. The advantage of this method is that unlike the above method converts the time according to the time zone of the country and returns that in the string.
function changeTimezone() {
let date = new Date(Date.UTC(2021, 5, 28, 3, 0, 0));
console.log('Date in India: ' + date);
let usDate = date.toLocaleString('en-US', {timeZone: 'America/New_York'});
console.log('Date in USA: ' + usDate);
}
Output:
Date in India: Mon Jun 28 2021 08:30:00 GMT+0530 (India Standard Time)
Date in USA: 6/27/2021, 11:00:00 PM
In the above function, we first create a Date object using the Date()
constructor. We call the toLocaleString
function on the date specifying the language tag
and the timeZone
and get the date/time converted to a different time zone.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn