How to Display DateTime in 12-Hour AM/PM Format in JavaScript
- Display DateTime in 12-Hour AM/PM Format By Creating Own Function Method in JavaScript
-
Display DateTime in 12-Hour AM/PM Format Using the
Date.prototype.toLocaleString
Method in JavaScript -
Display DateTime in 12-Hour AM/PM Format Using the
Date.prototype.toLocaleTimeString
Method in JavaScript -
Display DateTime in 12-Hour AM/PM Format Using the
moment.js
Method in JavaScript - Conclusion
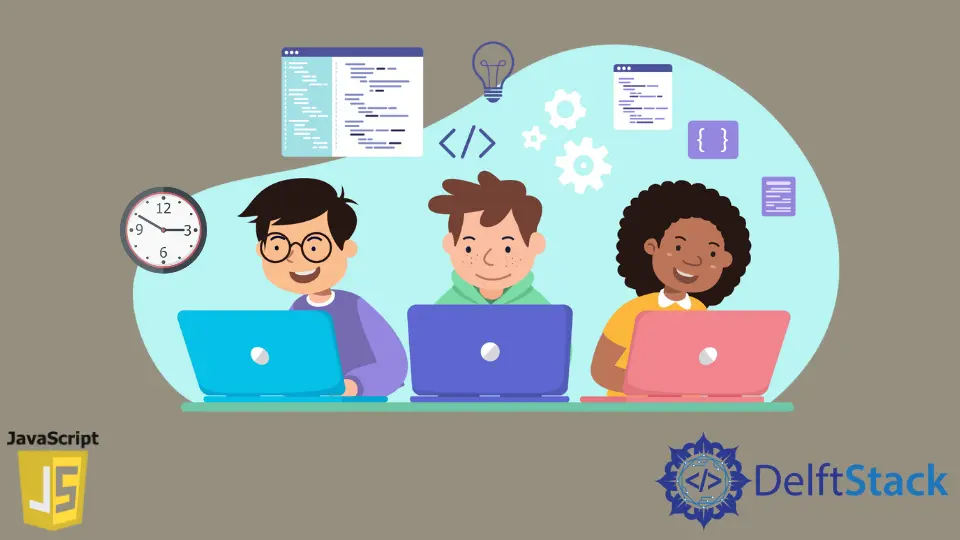
In JavaScript, the 24-hour format is used as the default for date-time. We can also display date-time in the 12-hour AM/PM format using several different approaches.
In this tutorial, we’ll explore these methods. The 12-hour time will be displayed in hours, minutes, and seconds (HH:MM:SS) format with AM/PM.
Display DateTime in 12-Hour AM/PM Format By Creating Own Function Method in JavaScript
The first method you can format a JavaScript date-time into a 12-hour AM/PM format is by creating your function.
Firstly, change the date-time format by only using native methods in this approach. To illustrate this in layman’s terms, use the following example: apply the modulo %
operator to find the date-time in 12-hour format and use the conditional ?
operator to apply AM or PM.
Example:
const formatAMPM =
(date) => {
let hours = date.getHours();
let minutes = date.getMinutes();
let ampm = hours >= 12 ? 'pm' : 'am';
hours = hours % 12;
hours = hours ? hours : 12;
minutes = minutes.toString().padStart(2, '0');
let strTime = hours + ':' + minutes + ' ' + ampm;
return strTime;
}
console.log(formatAMPM(new Date(2022, 1, 1)));
You have the formatAMPM
function, which will take a JavaScript date
object as a parameter. Call getHours
to get the hours in the 24-hour format in the function and minutes
to get the minutes.
Then, create the ampm
variable and set it to am
or pm
depending on the value of hours. Change the hours to a 12-hour format using the %
operator to get the remainder when divided by 12.
Next, use the toString()
function to convert minutes to a string, and then use the padStart
function to pad a string with 0 if it’s only one digit. Finally, use strTime
to tie everything together.
Output:
Display DateTime in 12-Hour AM/PM Format Using the Date.prototype.toLocaleString
Method in JavaScript
The second method you will use is the Date.prototype.toLocaleString
method. This method makes it easier for the user to format a date-time to AM/PM format.
In this method, you will utilize an inbuilt function known as the toLocaleString
function to change the format of the given date. toLocaleString():
will return a string representation of the date Object.
The two arguments, Locale
and options,
allow for customization of the method’s behavior.
Example:
const str = new Date(2022, 1, 1)
.toLocaleString(
'en-US', {hour: 'numeric', minute: 'numeric', hour12: true})
console.log(str);
Call the toLocaleString
function on your date object with the locale
, object
, and some options. hour
is set to numeric to show the hours in a numerical format.
This is the same with minute
. Then, hour12
displays the hours in 12-hour format.
Output:
Display DateTime in 12-Hour AM/PM Format Using the Date.prototype.toLocaleTimeString
Method in JavaScript
The third method you can use is the Date.prototype.toLocaleTimeString
method. This method will replace toLocaleString
with toLocaleTimeString
and will give you the same result as the toLocaleString
function.
Example:
const str = new Date(2022, 1, 1)
.toLocaleTimeString(
'en-US', {hour: 'numeric', minute: 'numeric', hour12: true})
console.log(str);
Output:
Display DateTime in 12-Hour AM/PM Format Using the moment.js
Method in JavaScript
The fourth method which you can use is the moment.js
method. This method formats a date object into a 12-hour AM/PM format.
To do this, you will first call the format
method.
Where:
a
adds the AM/PM;hh
is the formatting code for a two-digit hour;mm
is the formatting code for a two-digit minute.
Example:
const str = moment(new Date(2022, 1, 1)).format('hh:mm a')
console.log(str);
Output:
Conclusion
In this article, you learned various methods such as creating our function, Date.prototype.toLocaleString
, Date.prototype.toLocaleTimeString
, and moment.js
, which can display JavaScript date-time in the 12-hour AM/PM format. All these methods are equally applicable and can easily be used.