How to Get Current Time in JavaScript
-
Get Current Time With the
Date
Object in JavaScript - Get Current Time in UTC Format in JavaScript
- Get the Entire Current Time in the Local Format in JavaScript
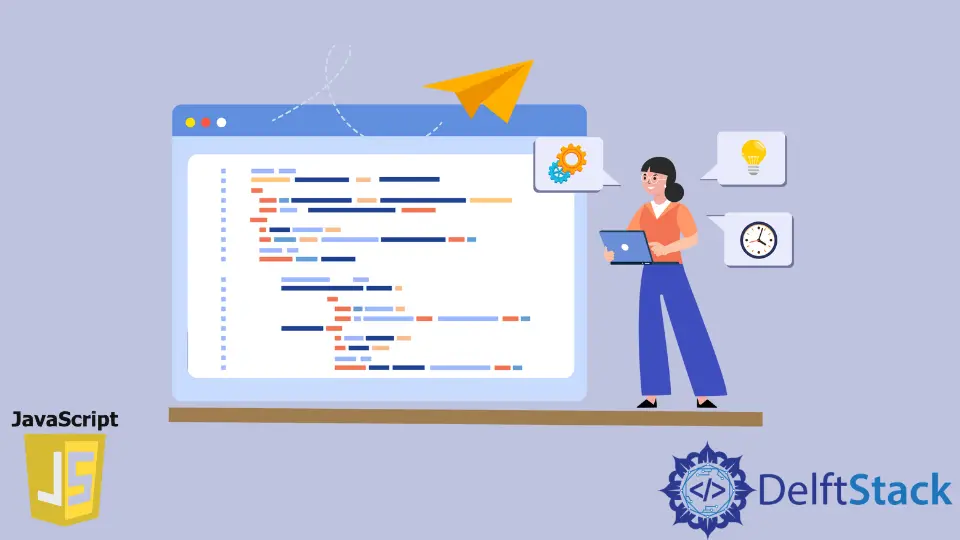
There are times when you might want to show the current date or time on your website, for example, when you create a web application like a digital clock where you want to show the current time to the user. It can easily be done with the help of the Date
object provided by the JavaScript language.
Get Current Time With the Date
Object in JavaScript
The Date
object in JavaScript allows us to work with date and time. Using this object, we can either set the time manually and then get it or get the data about the current time. Below are some of the methods which we can use to get the time in JavaScript.
Methods | Purpose |
---|---|
getHours() |
It returns the hours, according to universal time (0-23) |
getMinutes() |
It returns the minutes, according to universal time (0-59) |
getSeconds() |
It returns the seconds, according to universal time (0-59) |
Let’s try to understand this with the help of the below code example. The below program will display the current time on the browser. We all know that time consists of three things, hours, minutes, and seconds. To get each of these data, we have different methods available in JavaScript.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<p id="displayHours"></p>
<p id="displayMinutes"></p>
<p id="displaySeconds"></p>
<script>
var displayHours = document.getElementById('displayHours');
var displayMinutes = document.getElementById('displayMinutes');
var displaySeconds = document.getElementById('displaySeconds');
var currentTime = new Date();
displayHours.innerHTML = currentTime.getHours() + " hrs";
displayMinutes.innerHTML = currentTime.getMinutes() + " min";
displaySeconds.innerHTML = currentTime.getSeconds() + " sec";
</script>
</body>
</html>
Output:
22 hrs
56 min
19 sec
For displaying these 3 things on the webpage, we will use 3 paragraph tags. Each of these tags has an id
associated with it. Using this id
we will be able to access these HTML elements inside our JavaScript program using the document.getElementById()
method. After that, we will store each of these HTML tags inside the variables with the names displayHours
, displayMinutes
, and displaySeconds
.
To get the current time, we have to call the Date
object as a constructor (notice we have a new
keyword there). This Date
object will return a number that we will store inside the currentTime
variable. The currentTime
variable now contains the entire time (hours, minutes, and seconds).
We have the current time and the 3 paragraph tags, with the help of which we will be displaying the time on our browser. To get the individual fields i.e hours, minutes and seconds we will use getHours()
, getMinutes()
and getSeconds()
methods respectively on the currentTime
variable. Using the innerHTML
property, we can assign these individual fields to each of the paragraph tags. If you execute the above code on the browser as an HTML document, you will get the current time as an output on the browser window.
Since this program will display the current local time based on our computer, the above output may vary depending upon the time you are executing the above code.
If you want the entire time with the time format (GMT, UTC, IST, etc) and the date, you can directly assign the currentTime
variable to the paragraph tag using the innerHTML
property as shown below.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<p id="dateAndTime"></p>
<script>
var dateAndTime = document.getElementById('dateAndTime');
var currentTime = new Date();
dateAndTime.innerHTML = currentTime;
</script>
</body>
</html>
Output:
Wed Apr 14 2021 23:30:12 GMT+0530 (India Standard Time)
Get Current Time in UTC Format in JavaScript
There are different methods with the help of which you can get the time in Universal Time Coordinate (UTC) format. These methods work almost the same way as that of the above methods.
Below is a list of methods that will give you the time in UTC format.
Methods | Purpose |
---|---|
getUTCHours() |
It returns hour value in universal time format (0-23) |
getUTCMinutes() |
It returns minutes value in universal time format (0-59) |
getUTCSeconds() |
It returns seconds value in universal time format(0-59) |
Get the Entire Current Time in the Local Format in JavaScript
The methods we have seen only return a particular part of the time, either only hours, minutes, or seconds and not the entire time itself. So, to get the whole current time in the local format, we can use a method named as toLocaleTimeString()
.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<p id="dateAndTime"></p>
<script>
var dateAndTime = document.getElementById('dateAndTime');
var currentTime = new Date();
dateAndTime.innerHTML = currentTime.toLocaleTimeString();
</script>
</body>
</html>
Output:
11:43:06 PM
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn