JavaScript Date.getTime()
-
Date
Object in JavaScript -
Use the
getTime()
Method in JavaScriptDate
Objects -
Similar Method to the
getTime()
Approach - Conclusion
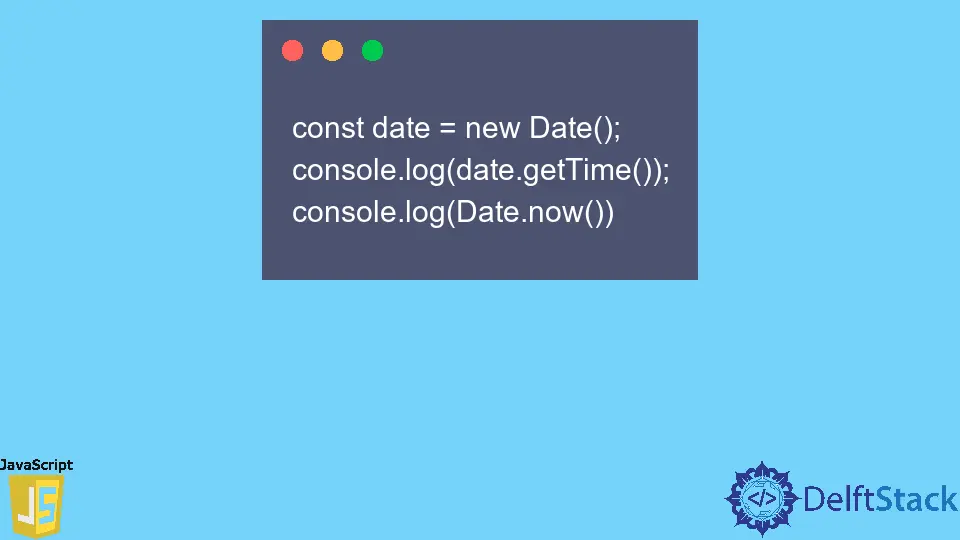
In web development, we use some essential components and languages often. JS, also known as JavaScript, is one of those languages we use to make static web pages dynamic and interactive.
JavaScript provides us with many features in various ways, and the Date
object is another significant feature we can use on many occasions.
Date
Object in JavaScript
Date
objects in JavaScript provide us with time-related information from 1 January 1970 up to the current. In JavaScript, we can derive the current date and time using the below code snippet.
console.log(Date());
And it returns our expected output as below.
Output:
Use the getTime()
Method in JavaScript Date
Objects
Date
objects provide some methods to help us complete different tasks. Some of them are getDate()
, getDay()
, getMonth()
, getMinutes()
, getTime()
, etc.
The getTime()
method is crucial among those methods. The main task of the getTime()
process is to return the time from 1 January 1970 to now.
The time we get has the unit in milliseconds. Let’s see how we can output the time using the getTime()
method in JavaScript Date
objects.
We can follow the two simple steps below to get the time using the above method.
- Assign a new
Date
object to a variable for ease of use. - Use the
getTime()
method along with that variable.
Refer to the below code chunk.
const date = new Date();
console.log(date.getTime());
The first statement consists of our first step, assigning the Date
object to a new variable. Here, we have set it to a variable called date
.
In the second statement, we have used the getTime()
method and the newly created date
variable to have the time from 1 January 1970 up to now.
When we execute the code, the following output will be displayed.
Output:
As you can see, the time from 1 January 1970 to now has been provided to us in milliseconds.
Also, we can use the getTime()
method to derive the time from a given date and time string. Let’s try to do that.
Firstly, we can create a new Date
object, and while making it, we can assign a random date and time. Then, we can use the getTime()
method to derive the time from that Date
object.
Refer to the below code snippet.
var newDate = new Date('November 12, 2022 11:30:25');
console.log(newDate.getTime());
Here, we gave a random date to the newDate
variable and printed the output.
Below is the result we get.
If we give a date before 1 January 1970, we will get the time as a minus value because the start time of the JS is set from 1 January 1970. The time of a particular date before the starting date and time is outputted as a minus value.
See the below code.
var newDate = new Date('November 12, 1969 11:30:25');
console.log(newDate.getTime());
In the above code, the year is older than 1970, and the output is below.
Output:
As you can see, we get the time as a minus value.
Let’s try another example.
var newDate = new Date('November 45, 2022 11:30:25');
console.log(newDate.getTime());
In this example, we have given 45 as the value of the Day, which is greater than 31. After we execute the code, the following result will appear to us.
Output:
As you can see, it provides us with NaN
as the output, which refers to Not-a-Number, and the reason is a month cannot have days more than 31.
Similar Method to the getTime()
Approach
In JavaScript Date
objects, another method is provided to us, similar to the getTime()
process. That is the now()
method.
The now()
method also gives us the time from 1 January 1970 to the current time in milliseconds.
The difference between the getTime()
method and the now()
method is now()
method is 2x faster than the getTime()
method. When we use the getTime()
method, we should create a new Date
class and then use the getTime()
method.
It is slowing its performance when compared to the now()
method, but both the getTime()
and now()
processes provide us with the same output. The now()
method is the right choice if a developer wants faster performance.
Let’s try these methods to check whether they give us the same result. See the below code block we have implemented.
const date = new Date();
console.log(date.getTime());
console.log(Date.now())
This is the result that will appear after deploying the above-implemented code.
Output:
As you can see, we get the same time in milliseconds from both methods.
Conclusion
JavaScript provides us with the Date
module on many occasions. In this write-up, we looked at the methods that the Date
object gives us to ease our work.
Also, we learned about the getTime()
method in Date
objects and situations we might get unexpected outputs, along with the reasons. Then, we looked at the now()
method as a similar method to the getTime()
method, which is 2x times faster than the getTime()
method.
Overall, the getTime()
is a valuable method to achieve many goals when interacting with dates and times.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.