How to Get Name of Month in JavaScript
-
Using the
toLocaleString
Function in JavaScript -
Using the
Intl.DateTimeFormat
Object in JavaScript - Using Custom Code to Get Month Name From a Given Date in JavaScript
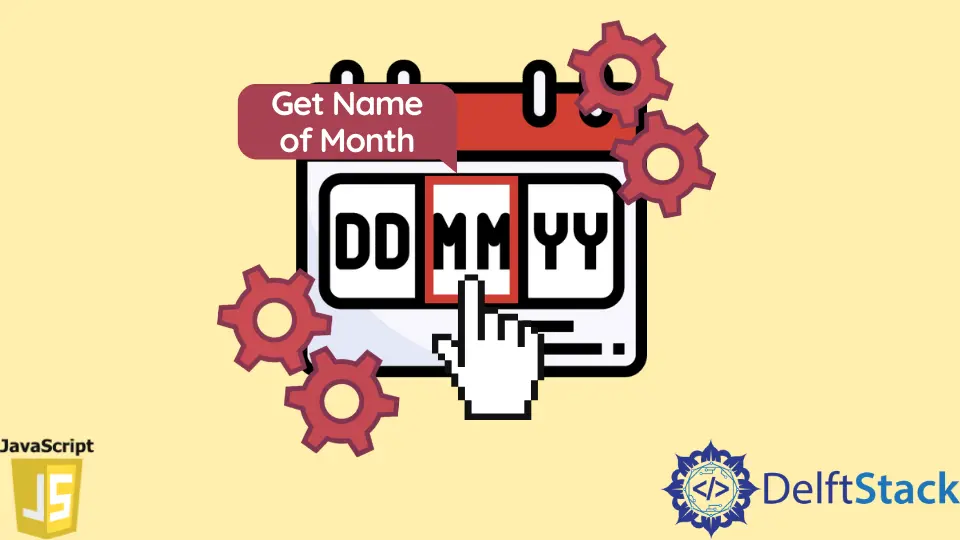
In JavaScript, we have a getMonth()
method of date object. It returns the index of a month starting from 0
. But most applications in real-world scenarios demand this month to be in alphabetical name to make it easier for the end-user to read through. It may be hard to write code to represent the month name for the given month index. Let us look at a few ways to get the name of a month in JavaScript.
Using the toLocaleString
Function in JavaScript
We can use the toLocaleString
function of javascript to get the month name from a date object. It offers options to return the month names in a given language supported by the toLocaleString
function.
Syntax of the toLocaleString
Function
toLocaleString()
toLocaleString(locales)
toLocaleString(locales, options)
Parameters:
The function usually is used without any parameters to get the date string. toLocaleString
accepts two optional parameters, the first one being the language key (for example, en-US
for English, ar-EG
for Arabic etc.), and the second one is a set of configurations passed as options
, which is an object.
Return Type:
toLocaleString
returns a string value representing the date in the format MM/DD/YYYY, hh:mm:ss a
. a
is for AM / PM
.
We can use the function to get both the long and shorter forms of the month names. The longer ones display the full month names, whereas the shorter ones list only the first three characters of the month name in the output. The usage is as follows.
const dateObj = new Date('1-1-2021');
const dateString = dateObj.toLocaleString();
const enDateString = dateObj.toLocaleString('en-US');
const monthNameLong = dateObj.toLocaleString('en-US', {month: 'long'});
const monthNameShort = dateObj.toLocaleString('en-US', {month: 'short'});
console.log(dateString);
console.log(enDateString);
console.log(monthNameLong);
console.log(monthNameShort);
Output:
1/1/2021, 12:00:00 AM
1/1/2021, 12:00:00 AM
January
Jan
We get longer month names with the { month: 'long' }
passed as the option
. For shorter month names, we need to set the month configuration to short
as in { month: 'short' }
.
Note
toLocaleString
is supported by all browsers, including the Internet Explorer browser.- Comparing the date string output to a date stored as a string in a variable may not give consistent results across browsers. For instance, the following code may run differently in Firefox and Internet Explorer and older versions of MS Edge browsers.
('1/5/2021, 6:00:00 PM' ===
new Date('2021-01-05T12:30:00Z').toLocaleString('en-US')) ?
true :
false;
Output in Chrome:
true
Output in Internet Explorer:
false
It happens because the Internet Explorer and older versions of edge insert control characters at the start and end of the date string. You can try checking the length of the string output by new Date("2021-01-05T12:30:00Z").toLocaleString("en-US")
in chrome and Internet Explorer to observe the difference. IE says the length as 32 whereas, chrome says it as 20. And also, the character at the zeroth position in IE consoles as ""
whereas in chrome, it gives "1"
.
Using the Intl.DateTimeFormat
Object in JavaScript
Intl.DateTimeFormat
returns a DateTimeFormat
object. It has a format
function that takes the target date as input and formats it based on the options
we set. It is similar to the toLocaleString
function. The difference is that the toLocaleString
is applied on an object with data type as a date. Whereas in the Intl.DateTimeFormat
, we pass the date as an argument to the format
function. The parameter names differ for various date format scenarios (Refer MDN docs for more details). Intl.DateTimeFormat
is supported in all browsers. We can get the longer and shorter names for the months using Intl.DateTimeFormat
as depicted in the following code.
new Intl.DateTimeFormat('en-US', {month: 'short'})
.format(new Date('1-1-2021')) new Intl
.DateTimeFormat('en-US', {month: 'long'})
.format(new Date('1-1-2021'))
Output:
Jan
January
Note
- The
Intl.DateTimeFormat
constructor is created for formatting dates.Intl.DateTimeFormat
has much more flexibility with the formatting of thedate
objects. We can use it to get the date string in a local language. It even supports an option for providing a fallback language in itsoptions
object. - It is supported in all web browsers, including the older versions of Internet Explorer.
Using Custom Code to Get Month Name From a Given Date in JavaScript
If we do not want to use the above two inbuilt JavaScript methods, we can write a custom code to get the month name for a corresponding date. We can write the code in various ways. We can use two arrays: one holding the long month names and the other with shorter month names. But following is the concise and efficient code that uses a single array of month names to return the full name and short name. Let us look at the following code.
const monthNames = [
'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August',
'September', 'October', 'November', 'December'
];
getLongMonthName =
function(date) {
return monthNames[date.getMonth()];
}
getShortMonthName =
function(date) {
return monthNames[date.getMonth()].substring(0, 3);
}
console.log(getLongMonthName(new Date('1-1-2021')));
console.log(getShortMonthName(new Date('1-1-2021')));
console.log(getLongMonthName(new Date('11-5-2021')));
console.log(getShortMonthName(new Date('11-5-2021')));
console.log(getLongMonthName(new Date('12-8-2021')));
console.log(getShortMonthName(new Date('12-8-2021')));
Output:
January
Jan
November
Nov
December
Dec
We use two functions in the above code snippet: one to get the full name and the other for shorter ones.
- We code the
getLongMonthName()
function that takes a date object as a parameter and returns the full month name corresponding to it. It uses the inbuilt function in javascript,getMonth()
. ThegetMonth()
function of the date object returns the month index. We use this index to get the month name from themonthNames
array that holds the full name of the months. - We have the
getShortMonthName
function that has an additional step to shorten the month name. It uses the same logic as thatgetLongMonthName
. We truncate the final result to three characters using thesubstring()
function of javascript. Hence, we get the short month name for the date passed as the parameter. - We may need to modify the
monthNames
array to support more than one language to allow localization and internationalization of the code. We can use different string arrays for storing month names of various languages. And it may not always be true that the shorter month names be just the first three characters. Each language has its representation for shorter month names. Hence, we may need to use two arrays for such languages: one containing the longer month names and the other containing the shorter month names. The logic ingetLongMonthName()
can still be used to get the month name corresponding to the date value passed as a parameter.