在 JavaScript 中获取月份名称
-
在 JavaScript 中使用
toLocaleString
函数 -
在 JavaScript 中使用
Intl.DateTimeFormat
对象 - 在 JavaScript 中使用自定义代码从一个给定的日期中获取月份名称
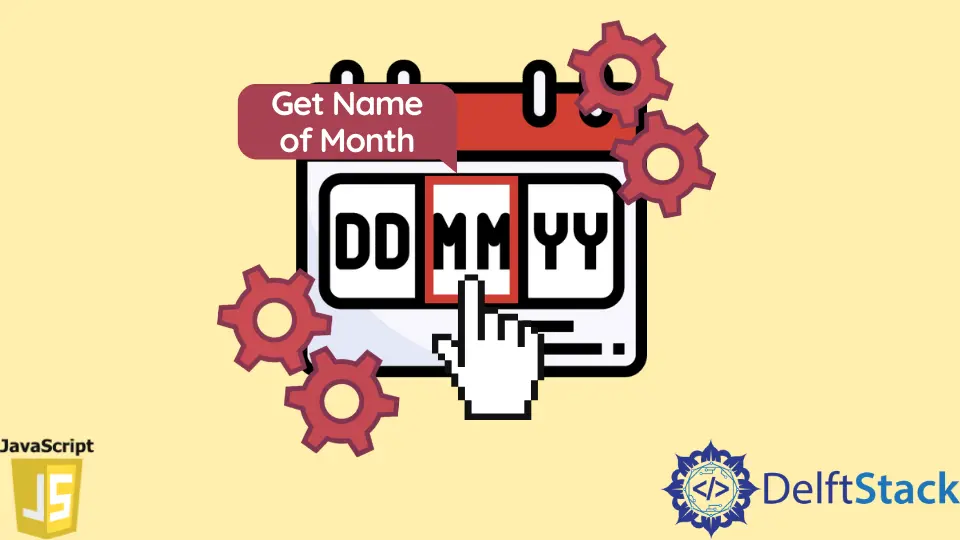
在 JavaScript 中,我们有一个日期对象的 getMonth()
方法。它返回从 0
开始的一个月的索引。但现实世界场景中的大多数应用程序都要求本月使用字母名称,以便最终用户更容易阅读。编写代码来表示给定月份索引的月份名称可能很困难。让我们看看在 JavaScript 中获取月份名称的几种方法。
在 JavaScript 中使用 toLocaleString
函数
我们可以使用 javascript 的 toLocaleString
函数从日期对象中获取月份名称。它提供了以 toLocaleString
函数支持的给定语言返回月份名称的选项。
toLocaleString
函数的语法
toLocaleString()
toLocaleString(locales)
toLocaleString(locales, options)
参数:
该函数通常在不带任何参数的情况下使用来获取日期字符串。toLocaleString
接受两个可选参数,第一个是语言键(例如,en-US
代表英语,ar-EG
代表阿拉伯语等),第二个是作为 options
传递的一组配置选项。options
是一个对象。
返回类型:
toLocaleString
以 MM/DD/YYYY, hh:mm:ss a
格式返回表示日期的字符串值。a
表示 AM / PM
我们可以使用该函数来获取月份名称的长短形式。较长的显示完整的月份名称,而较短的仅列出输出中月份名称的前三个字符。用法如下。
const dateObj = new Date('1-1-2021');
const dateString = dateObj.toLocaleString();
const enDateString = dateObj.toLocaleString('en-US');
const monthNameLong = dateObj.toLocaleString('en-US', {month: 'long'});
const monthNameShort = dateObj.toLocaleString('en-US', {month: 'short'});
console.log(dateString);
console.log(enDateString);
console.log(monthNameLong);
console.log(monthNameShort);
输出:
1/1/2021, 12:00:00 AM
1/1/2021, 12:00:00 AM
January
Jan
我们通过将 { month: 'long' }
作为 option
传递来获得更长的月份名称。对于较短的月份名称,我们需要将月份配置设置为 short
,如 { month: 'short' }
。
注意
- 所有浏览器都支持
toLocaleString
,包括 Internet Explorer 浏览器。 - 将日期字符串输出与在变量中存储为字符串的日期进行比较可能无法在浏览器中提供一致的结果。例如,以下代码在 Firefox 和 Internet Explorer 以及旧版本的 MS Edge 浏览器中的运行方式可能不同。
('1/5/2021, 6:00:00 PM' ===
new Date('2021-01-05T12:30:00Z').toLocaleString('en-US')) ?
true :
false;
Chrome 中的输出:
true
Internet Explorer 中的输出:
false
这是因为 Internet Explorer 和旧版本的 Edge 在日期字符串的开头和结尾插入了控制字符。你可以尝试在 chrome 和 Internet Explorer 中通过 new Date("2021-01-05T12:30:00Z").toLocaleString("en-US")
检查字符串输出的长度以观察差异。IE 将长度表示为 32,而 chrome 将其表示为 20。此外,IE 控制台中第零位置的字符为""
,而在 chrome 中,它给出了 "1"
。
在 JavaScript 中使用 Intl.DateTimeFormat
对象
Intl.DateTimeFormat
返回一个 DateTimeFormat
对象。它有一个 format
函数,它将目标日期作为输入,并根据我们设置的 options
对其进行格式化。它类似于 toLocaleString
函数。不同之处在于 toLocaleString
应用于数据类型为日期的对象。而在 Intl.DateTimeFormat
中,我们将日期作为参数传递给 format
函数。参数名称因各种日期格式方案而异(有关更多详细信息,请参阅 MDN 文档)。所有浏览器都支持 Intl.DateTimeFormat
。我们可以使用 Intl.DateTimeFormat
获取月份的长短名称,如下面的代码所示。
new Intl.DateTimeFormat('en-US', {month: 'short'})
.format(new Date('1-1-2021')) new Intl
.DateTimeFormat('en-US', {month: 'long'})
.format(new Date('1-1-2021'))
输出:
Jan
January
注意
Intl.DateTimeFormat
构造函数用于格式化日期。Intl.DateTimeFormat
在date
对象的格式方面具有更大的灵活性。我们可以使用它来获取本地语言的日期字符串。它甚至支持在其options
对象中提供后备语言的选项。- 所有网络浏览器都支持它,包括旧版本的 Internet Explorer。
在 JavaScript 中使用自定义代码从一个给定的日期中获取月份名称
如果我们不想使用上面两个内置的 JavaScript 方法,我们可以编写一个自定义代码来获取相应日期的月份名称。我们可以用各种方式编写代码。我们可以使用两个数组:一个保存长月份名称,另一个保存较短的月份名称。但以下是使用月份名称的单个数组来返回全名和短名称的简洁高效的代码。让我们看看下面的代码。
const monthNames = [
'January', 'February', 'March', 'April', 'May', 'June', 'July', 'August',
'September', 'October', 'November', 'December'
];
getLongMonthName =
function(date) {
return monthNames[date.getMonth()];
}
getShortMonthName =
function(date) {
return monthNames[date.getMonth()].substring(0, 3);
}
console.log(getLongMonthName(new Date('1-1-2021')));
console.log(getShortMonthName(new Date('1-1-2021')));
console.log(getLongMonthName(new Date('11-5-2021')));
console.log(getShortMonthName(new Date('11-5-2021')));
console.log(getLongMonthName(new Date('12-8-2021')));
console.log(getShortMonthName(new Date('12-8-2021')));
输出:
January
Jan
November
Nov
December
Dec
我们在上面的代码片段中使用了两个函数:一个用于获取全名,另一个用于获取较短的名称。
- 我们编写了
getLongMonthName()
函数,该函数将日期对象作为参数并返回与其对应的完整月份名称。它使用 javascript 中的内置函数getMonth()
。日期对象的getMonth()
函数返回月份索引。我们使用这个索引从保存月份全名的monthNames
数组中获取月份名称。 - 我们有
getShortMonthName
函数,它有一个额外的步骤来缩短月份名称。它使用与getLongMonthName
相同的逻辑。我们使用 javascript 的substring()
函数将最终结果截断为三个字符。因此,我们得到作为参数传递的日期的短月份名称。 - 我们可能需要修改
monthNames
数组以支持一种以上的语言,以允许代码的本地化和国际化。我们可以使用不同的字符串数组来存储各种语言的月份名称。较短的月份名称可能只是前三个字符,这可能并不总是正确的。每种语言都有其较短月份名称的表示形式。因此,我们可能需要为此类语言使用两个数组:一个包含较长的月份名称,另一个包含较短的月份名称。getLongMonthName()
中的逻辑仍可用于获取与作为参数传递的日期值对应的月份名称。