How to Convert Milliseconds to Date in JavaScript
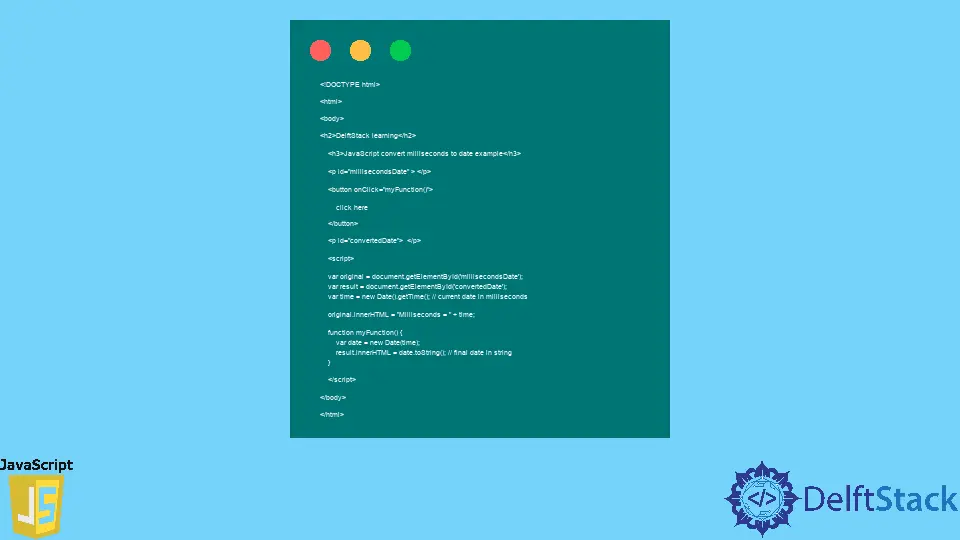
This article will discuss generating a date from milliseconds using JavaScript’s default date
object and methods.
Convert Milliseconds to Date in JavaScript
To generate a date from milliseconds in JavaScript, we can use the default JavaScript date
object and its methods. Firstly, we must be required to pass the millisecond value as a parameter to the date
object and convert a date into the desired date format.
There are multiple default methods to convert date format. Mostly, developers need to convert date content to string, as shown below in syntax.
Basic Syntax:
let date = new Date(milliseconds); // convert date from milliseconds
date.toString(); // change format
Generate String Date From Milliseconds in JavaScript
The following example will create a simple webpage in which we will generate the current date
object and convert it into milliseconds by the date.time()
method. Then, with that millisecond value, we will generate a string format of the current date at the click of a button.
Example code:
<!DOCTYPE html>
<html>
<body>
<h2>DelftStack learning</h2>
<h3>JavaScript convert milliseconds to date example</h3>
<p id="millisecondsDate" > </p>
<button onClick="myFunction()">
click here
</button>
<p id="convertedDate"> </p>
<script>
var original = document.getElementById('millisecondsDate');
var result = document.getElementById('convertedDate');
var time = new Date().getTime(); // current date in milliseconds
original.innerHTML = "Milliseconds = " + time;
function myFunction() {
var date = new Date(time);
result.innerHTML = date.toString(); // final date in string
}
</script>
</body>
</html>
In the above HTML source code, we have used the paragraph tag <p></p>
to display the converted date. In <script>
tags, we have generated the current date and get the milliseconds.
We declared myFunction()
and generated a new date by passing milliseconds as a parameter. Now, convert the date into string format using the toString()
method.
We assigned the final converted date to the paragraph tag using innerHTML
. Here, we called myFunction()
by clicking a button.
You can save the above source with the extension of HTML and open it in the browser to see the results.
Common Date Conversions From Milliseconds in JavaScript
Here are some common methods for date conversion from milliseconds to various date formats in JavaScript.
let originalDate = new Date(milliseconds);
originalDate.toLocaleString(); // output will be: "D/MM/YYYY, H:MM:SS PM/AM"
originalDate.toLocaleDateString(); //output will be: "D/MM/YYYY"
originalDate.toDateString(); // output will be: "Day Month DD YYYY"
originalDate.toTimeString(); // output will be: "HH:MM:SS GMT+0530"
originalDate.toString(); // output will be: "Day Month DD YYYY HH:MM:SS GMT+0500"
originalDate.toLocaleTimeString(); // output will be: "H:MM:SS AM/PM"