How to Validate a Date in JavaScript
-
Validate Date With the
moment.js
Library in JavaScript - Validate Date Using Regular Expressions in JavaScript
-
Validate Date With With
Date.parse()
Method in JavaScript
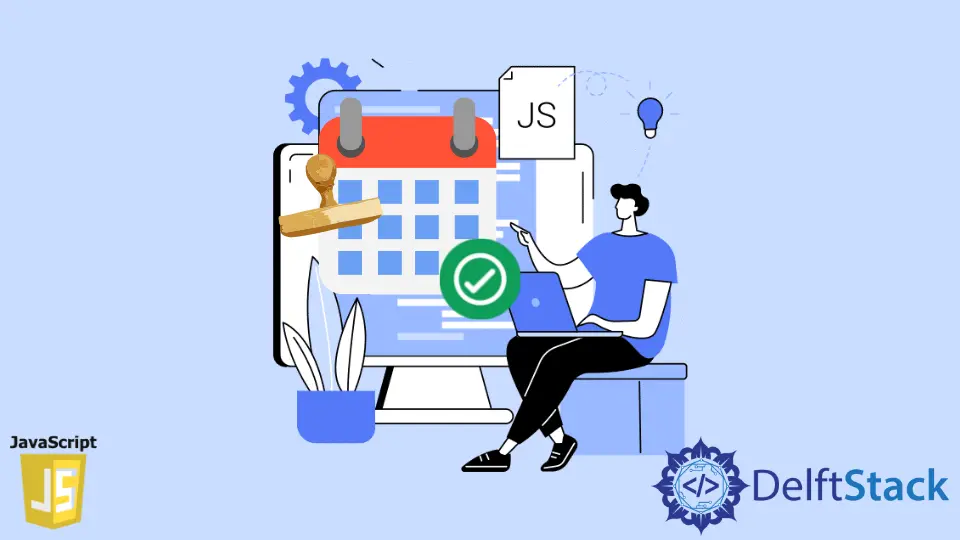
Validating a date becomes important to validate dates in JavaScript because various people at various locations follow different formats while entering dates. We have to follow a particular format while validating dates in our web application so that later it becomes easier for us to work with the date.
This article will introduce how to validate the date in mm/dd/yy
format in JavaScript.
Validate Date With the moment.js
Library in JavaScript
The moment.js
library is the best and easiest way of validating and formatting dates and times in JavaScript. We will use this library for validating and displaying dates in JavaScript. To use this library, we first have to download it using the below command.
npm install -g moment --save
It will install a moment in your system. Then we can directly use the moment()
method available in the moment.js
library. Below is the code to validate a date with moment.js
in JavaScript.
import * as moment from 'moment';
let result = moment('05/22/12', 'MM/DD/YY', true).isValid();
console.log(result)
Output:
true
The moment
function takes three parameters as an input; the first one is the input date that we want to validate, the second one is the format that we want our date to follow, and the last parameter is optional; if it is set to true
then it will use the strict parsing. The strict parsing requires that the format and input match exactly, including delimiters. At last, we use the isValid()
function to check whether the input date is valid or not and returns a boolean value of true
if the input date matches the format dd/mm/yy
and false
if the input date doesn’t match the format specified.
Validate Date Using Regular Expressions in JavaScript
Regular expression is a great way of validating date. The only issue that many people face when it comes to regular expressions is the difficulty in understanding them as they contain various symbols and numbers. Each symbol or expression in a regular expression has its own meaning. Using these expressions, we can validate the date in dd/mm/yy
format in JavaScript.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="message"></h1>
<script>
var date_regex = /^(0[1-9]|1[0-2])\/(0[1-9]|1\d|2\d|3[01])\/(0[1-9]|1[1-9]|2[1-9])$/;
var testDate = "03/17/21"
if (date_regex.test(testDate)) {
document.getElementById("message").innerHTML = "Date follows dd/mm/yy format";
}
else{
document.getElementById("message").innerHTML = "Invalid date format";
}
</script>
</body>
</html>
Output in Browser:
Date follows dd/mm/yy format
The regular expression /^(0[1-9]|1[0-2])\/(0[1-9]|1\d|2\d|3[01])\/(0[1-9]|1[1-9]|2[1-9])$/
has 3 groups. The first group represents month
, the second group represents day
, and the third group represents the last 2 digits of the year
. Wherever you see forward slash /
, it represents the starting of the group, and backslash \
represents the end of the group.
There are 12 months in a year. So that there are two possibilities when selecting a month, either it can start with 0
followed by a number or with 1
followed by the number 1
or 2
. The same process is similar for other groups. The \d
in group second represents any digit between 0 and 9. You can also create your regular expressions for validating dates.
We then store this regular expression in the date_regex
variable. We will use the date which is stored inside myDate
to check if the date follows the dd/mm/yy
format or not. If the date follows this format, we will print the Date follows dd/mm/yy format
inside the console; otherwise, we will print Invalid date format
.
Validate Date With With Date.parse()
Method in JavaScript
The Data.parse()
is a method that is already available in JavaScript. This method only takes a date as a single parameter which is a string.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="message"></h1>
<script>
let isValidDate = Date.parse('03/17/21');
if (isNaN(isValidDate)) {
document.getElementById('message').innerHTML = "This is not a valid date format.";
}
else{
document.getElementById('message').innerHTML = "This is a valid date format.";
}
</script>
</body>
</html>
Output in Browser:
This is a valid date format.
The Data.parse()
function will return a number in milliseconds based on the input date provided. If it does not recognize the input date, then it will return NaN (not a number) as an output. The result of this function is stored inside the isValidDate
variable.
If the value inside the isValidDate
variable is NaN
(not a number), it will return false
, and the message that will be printed on the screen is This is not a valid date format.
. If it returns true
, then it will print the message This is the valid date format.
on the screen as the output.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn