How to Convert JSON to Object in JavaScript
- Understanding JSON and Its Importance
- Using JSON.parse() Method
- Safely Parsing JSON with Error Handling
- Working with Nested JSON Objects
- Conclusion
- FAQ
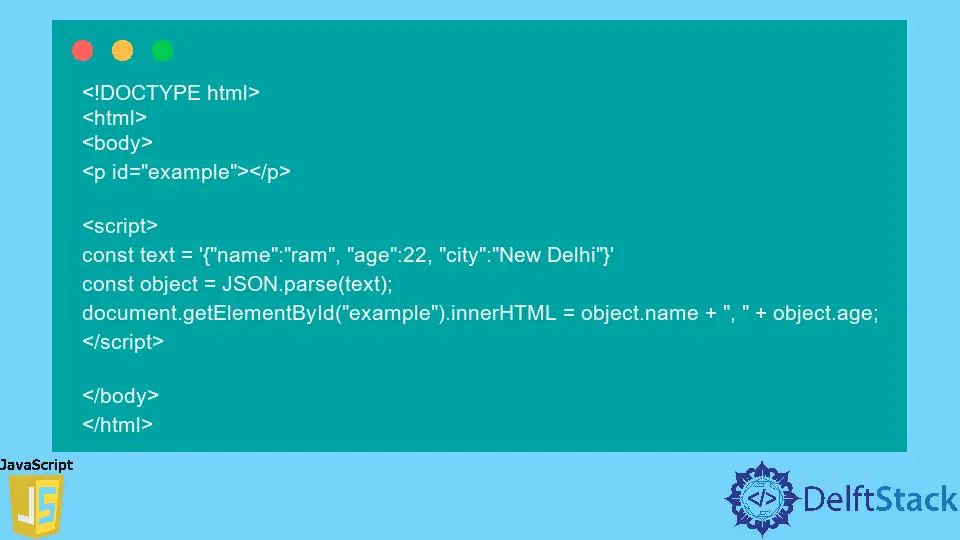
Converting JSON strings to JavaScript objects is a common task for developers, especially when working with APIs or handling data interchange formats. JSON, or JavaScript Object Notation, is a lightweight data format that’s easy for humans to read and write, and easy for machines to parse and generate.
In this tutorial, we will explore safe methods to transform JSON strings into JavaScript objects. We will walk through the process step-by-step, ensuring you understand each method thoroughly. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to handle JSON data effectively in your JavaScript applications.
Understanding JSON and Its Importance
Before diving into the conversion process, it’s essential to understand what JSON is and why it’s widely used. JSON is a text-based format that represents structured data based on JavaScript object syntax. Its simplicity and readability make it a popular choice for data interchange between a server and a client. In JavaScript, converting JSON to an object allows you to manipulate data easily, making it crucial for web development and API interactions.
Using JSON.parse() Method
One of the most straightforward ways to convert a JSON string into a JavaScript object is by using the built-in JSON.parse()
method. This method takes a JSON string as input and returns the corresponding JavaScript object. It’s important to ensure that the JSON string is properly formatted to avoid errors during parsing.
Here’s how you can do it:
const jsonString = '{"name":"John", "age":30, "city":"New York"}';
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject);
Output:
{ name: 'John', age: 30, city: 'New York' }
In this example, we have a JSON string representing a person with properties like name, age, and city. The JSON.parse()
method converts this string into a JavaScript object. Once converted, you can access the properties of the object using dot notation or bracket notation, just like any other JavaScript object.
However, it’s crucial to handle potential errors that might arise if the JSON string is malformed. You can achieve this by wrapping the JSON.parse()
call in a try-catch block to catch any exceptions that may occur during parsing.
Safely Parsing JSON with Error Handling
When working with JSON data, it’s essential to handle errors gracefully. Malformed JSON strings can lead to runtime exceptions, which can disrupt your application. To ensure robustness, you can implement error handling when parsing JSON.
Here’s an example of how to do this:
const jsonString = '{"name":"Jane", "age":25, "city":"Los Angeles"}';
try {
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject);
} catch (error) {
console.error("Invalid JSON string:", error);
}
Output:
{ name: 'Jane', age: 25, city: 'Los Angeles' }
In this code snippet, we attempt to parse a JSON string inside a try block. If the string is valid, it will successfully convert to a JavaScript object. If the string is invalid, the catch block will execute, logging an error message to the console. This approach ensures that your application can handle unexpected input without crashing, providing a better user experience.
Working with Nested JSON Objects
JSON can also represent complex data structures, including nested objects. When converting nested JSON strings to JavaScript objects, the process remains the same. You can still use JSON.parse()
to convert the string, and then access nested properties as needed.
Here’s an example:
const nestedJsonString = '{"person":{"name":"Alice", "age":28}, "city":"Chicago"}';
const nestedJsonObject = JSON.parse(nestedJsonString);
console.log(nestedJsonObject.person.name);
Output:
Alice
In this example, we have a JSON string containing a nested object under the “person” key. After parsing, we can access the name property of the nested object using dot notation. This feature is particularly useful when dealing with APIs that return complex data structures, allowing you to extract the information you need easily.
Conclusion
Converting JSON strings to JavaScript objects is a vital skill for any web developer. By using the JSON.parse()
method, you can quickly and safely transform JSON data into usable JavaScript objects. Remember to implement error handling to manage any potential issues that may arise from malformed JSON strings. With these techniques in your toolkit, you’ll be well-equipped to handle JSON data in your applications, paving the way for more dynamic and responsive web experiences.
FAQ
-
What is JSON?
JSON stands for JavaScript Object Notation, a lightweight format for data interchange. -
How do I handle errors when parsing JSON?
Use a try-catch block around theJSON.parse()
method to catch and manage exceptions.
-
Can I parse nested JSON objects?
Yes, you can parse nested JSON objects usingJSON.parse()
and access properties using dot notation. -
What happens if I try to parse an invalid JSON string?
An error will be thrown, which you can catch using a try-catch block to prevent your application from crashing. -
Is JSON only used in JavaScript?
No, JSON is language-independent and can be used in various programming languages, including Python, Java, and PHP.
Related Article - JavaScript JSON
- How to Format JSON in JavaScript
- How to Generate Formatted and Easy-To-Read JSON in JavaScript
- How to Convert XML to JSON in JavaScript
- How to Convert CSV to JSON in JavaScript
- How to POST a JSON Object Using Fetch API in JavaScript
- How to Convert JSON to XML in JavaScript