How to Get JSON Object From URL in PHP
-
Use the
file_get_contents()
Function to Get JSON Object From the URL in PHP -
Use
curl
to Get JSON Object From the URL in PHP
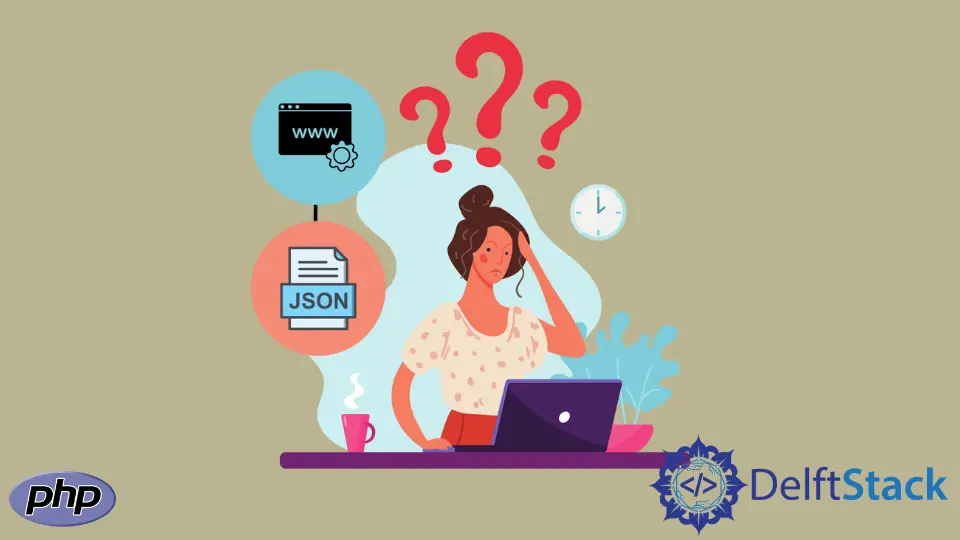
This tutorial introduces how to get the JSON object from a URL in PHP.
Use the file_get_contents()
Function to Get JSON Object From the URL in PHP
We can use file_get_contents()
along with json_decode()
to get the JSON object from a URL. The file_get_contents()
function reads the file in a string format. We should specify the path to the file in the function or we can even give the URL in the function as the first parameter. We should enable allow_url_fopen
to use the file_get_contents()
function. We can enable it by setting phpini_set("allow_url_fopen", 1)
in the php.ini
file. The json_decode()
function converts the JSON object into PHP object. Thus, we can access the objects in JSON URL as PHP objects.
For the demonstration, we will use a dummy JSON URL from jsonplaceholder. Create a variable $url
and store the URL in it. Use the URL https://jsonplaceholder.typicode.com/posts/1
. The JSON objects of the URL are shown below. Next, create a $json
variable and use the $url
as an argument to the file_get_contents()
function. Now, use the json_decode()
function to decode JSON string to PHP object. Store the object in the $jo
variable. Lastly, access the title
object with $jo
and print it out.
Thus, we accessed a URL containing a JSON object from the web and converted it into PHP. In this way, we can get JSON objects from a URL in PHP.
Example Code:
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
<?php
$url = 'https://jsonplaceholder.typicode.com/posts/1';
$json = file_get_contents($url);
$jo = json_decode($json);
echo $jo->title;
?>
Output:
sunt aut facere repellat provident occaecati excepturi optio reprehenderit
Use curl
to Get JSON Object From the URL in PHP
curl
is a command-line tool that is used to send and receive data and files. It uses supported protocols like HTTP, HTTPS, FTP, etc., and sends data from or to a server. In PHP, there is a curl
library that lets us make an HTTP request. We can use curl
to read file contents from the web. There are various curl
functions in PHP that facilitate us to send and receive data. We can use them to get JSON objects from a URL. The curl_init()
function initiates the curl. We can use the curl_setopt()
function to set several options like returning transfer and setting URLs. The curl_exec()
function executes the operation and curl_close()
closes the curl.
We can use the same URL as in the first method to demonstrate the use of curl
. Create a variable $curl
and initiate the curl with the curl_init()
function. Set the CURLOPT_RETURNTRANSFER
option true using the curl_setopt()
function. Next, set the URL using the CURLOPT_URL
option. Execute the curl with curl_exec()
function with $curl
in the parameter and store it in $res
variable. Use the curl_close()
function to close the $curl
variable. Next, use the json_decode()
function to change the JSON object to PHP object and display the title
object.
Thus, we can get the JSON object from a URL using curl
.
Example Code:
<?php
$curl= curl_init();
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_URL, 'https://jsonplaceholder.typicode.com/posts/1';
$res = curl_exec($curl);
curl_close($curl);
$jo = json_decode($res);
echo $jo->title; ?>
Output:
sunt aut facere repellat provident occaecati excepturi optio reprehenderit
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn