How to Convert Array to Set in JavaScript
-
Use the
set
Constructor to Convert Array to aSet
in JavaScript -
Use the
map()
Function to Convert Array toSet
in JavaScript -
Use the
reduce()
Function to Convert Array toSet
in JavaScript -
Use the
set
Constructor & themap()
Function to Convert an Array of Objects to MultipleSets
in JavaScript
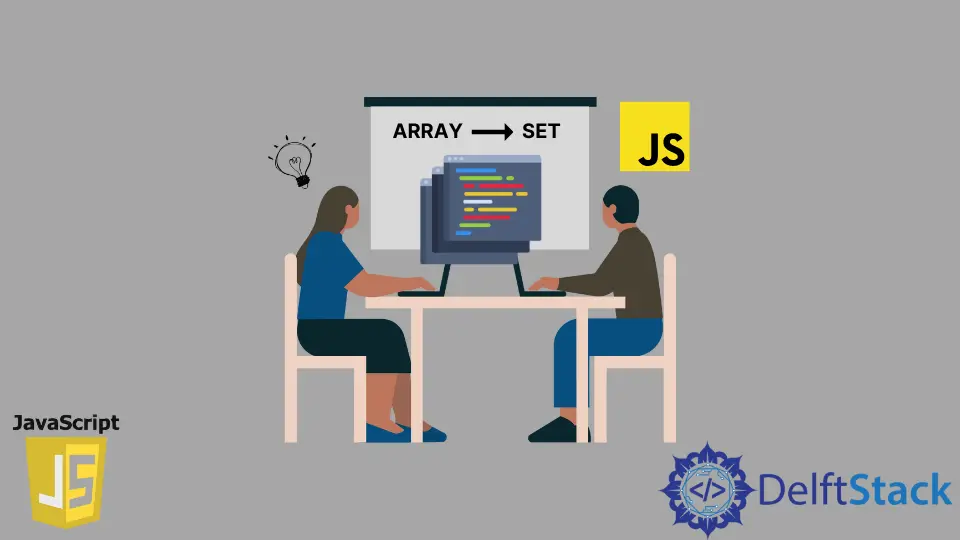
This tutorial shows the procedure for converting an array to a set
and learning about transforming an array of objects into multiple sets using JavaScript.
We can go through different routes for an array to a set
conversion in JavaScript. Some of them are listed below, which we’ll be exploring later in this tutorial.
set
Constructor.map()
Function.reduce()
Function.- Use the
set
Constructor and themap()
Function together.
Use the set
Constructor to Convert Array to a Set
in JavaScript
JavaScript Code:
var array = [5, 4, 6];
var set = new Set(array);
console.log(set.size === array.length);
console.log(set.has(6));
Output:
true
true
We pass the array
to a set
constructor to convert the array to a set
. Then, we compare the size/length of the array and set
using the set.size === array.length
.
Further, we use the has()
method to check whether the set
has the element in the array.
Use the map()
Function to Convert Array to Set
in JavaScript
JavaScript Code:
var array = [5, 5, 4, 6];
var set = new Set();
array.map(arrElement => set.add(arrElement));
set.forEach(item => {
console.log(item);
});
Output:
5
4
6
The map()
method constructs a new array by executing a method once for each array’s element without updating the actual array and the add()
method takes the array element and adds it into the set
.
Lastly, we use the forEach
loop to iterate over the set
and print the elements on the console.
As seen in the output above, it only has one number five(5
) in the set
. The set
contains the unique values only, so always be careful while converting to a set
and never convert to a set
when you have redundant data (repeated data/values).
Use the reduce()
Function to Convert Array to Set
in JavaScript
JavaScript Code:
var array = [5, 5, 4, 6];
var set = new Set();
array.reduce((_, item) => set.add(item), null);
set.forEach(item => {
console.log(item);
});
Output:
5
4
6
We used the reduce()
method to transform an array into a set
. The reduce()
method calls a reducer method for every element of the array and returns the accumulated answer as a single value.
Use the set
Constructor & the map()
Function to Convert an Array of Objects to Multiple Sets
in JavaScript
JavaScript Code:
let array = [
{name: 'Mehvish', age: 36}, {name: 'Thomas', age: 23},
{name: 'Christopher', age: 45}
];
let namesSet = new Set(array.map(item => item.name));
let ageSet = new Set(array.map(item => item.age));
namesSet.forEach(item => {
console.log(item);
});
ageSet.forEach(item => {
console.log(item);
});
Output:
"Mehvish"
"Thomas"
"Christopher"
36
23
45
This last example shows that we can convert an array of objects to multiple sets
. In our case, we made two sets
, one for the names and the other for the age.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript