How to Convert Set to Array in JavaScript
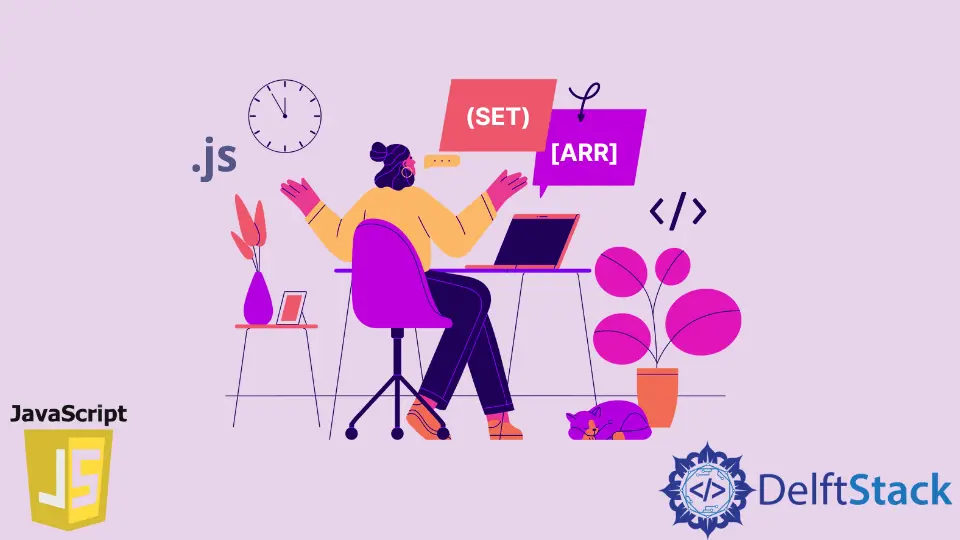
Converting a Set to an Array in JavaScript is a common task that developers encounter. Sets are useful for storing unique values, but sometimes you need to manipulate these values as an array for various operations like sorting, filtering, or mapping. Thankfully, JavaScript provides a few simple methods to accomplish this conversion.
In this article, we will explore these methods in detail, providing clear examples and explanations to help you understand the process. Whether you are a beginner or an experienced developer, this guide will equip you with the knowledge to efficiently convert Sets to Arrays in JavaScript.
Using the Spread Operator
One of the most elegant and straightforward ways to convert a Set to an Array in JavaScript is by using the spread operator (...
). This operator allows you to take the elements of the Set and spread them into a new Array. Here’s how you can do it:
const mySet = new Set([1, 2, 3, 4, 5]);
const myArray = [...mySet];
console.log(myArray);
Output:
[1, 2, 3, 4, 5]
In this example, we start by creating a Set called mySet
with a few numeric values. By using the spread operator, we create a new Array myArray
that contains all the elements from mySet
. The beauty of this method lies in its simplicity and readability. It’s a one-liner that effectively transforms the Set into an Array without any additional complexity. This technique is particularly useful when you need to quickly convert Sets to Arrays in your JavaScript applications.
Using Array.from()
Another effective method to convert a Set to an Array is by using the Array.from()
method. This built-in JavaScript function creates a new Array instance from an array-like or iterable object, including Sets. Here’s how you can use it:
const mySet = new Set(['apple', 'banana', 'cherry']);
const myArray = Array.from(mySet);
console.log(myArray);
Output:
['apple', 'banana', 'cherry']
In this code, we create a Set called mySet
containing three fruit names. By passing mySet
to Array.from()
, we create a new Array myArray
that holds the same values. This method is particularly useful when you want to convert other iterable objects, like Maps or NodeLists, into Arrays as well. The flexibility of Array.from()
makes it a valuable tool in your JavaScript toolkit, especially when working with various data structures.
Using the forEach()
Method
You can also convert a Set to an Array using the forEach()
method. This method allows you to iterate over each element in the Set and push them into a new Array. While this method may not be as concise as the previous ones, it provides a clear way to understand the conversion process. Here’s an example:
const mySet = new Set([10, 20, 30]);
const myArray = [];
mySet.forEach(value => {
myArray.push(value);
});
console.log(myArray);
Output:
[10, 20, 30]
In this example, we initialize an empty Array myArray
. We then use the forEach()
method on mySet
, which allows us to access each value in the Set. Inside the callback function, we push each value into myArray
. This method is particularly useful if you need to perform additional operations on each element during the conversion process. While it may take a few more lines of code, it provides clarity and control over how you handle each value.
Conclusion
Converting a Set to an Array in JavaScript is a straightforward process, thanks to the various methods available. Whether you choose the spread operator for its simplicity, Array.from()
for its versatility, or forEach()
for its control, each method has its unique advantages. Understanding these techniques will enhance your JavaScript skills and make your code more efficient. By mastering the conversion of Sets to Arrays, you open up new possibilities for data manipulation and processing in your applications.
FAQ
-
What is a Set in JavaScript?
A Set is a collection of unique values in JavaScript, meaning it cannot contain duplicate elements. -
Why would I need to convert a Set to an Array?
Converting a Set to an Array allows you to use array methods likemap()
,filter()
, andreduce()
, which are not available on Sets. -
Can I convert an Array back to a Set?
Yes, you can convert an Array back to a Set by passing the Array to the Set constructor, like this:const mySet = new Set(myArray);
. -
Are there performance differences between the methods?
Generally, the performance differences are negligible for small Sets. However, for larger Sets, the spread operator andArray.from()
may perform better thanforEach()
. -
Can I convert a Set with complex objects to an Array?
Yes, you can convert a Set containing complex objects to an Array, and the objects will retain their structure in the new Array.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript