How to Assign a Function to a Variable in JavaScript
- Example of Assigning a Function to a Variable Using JavaScript in HTML
-
Examine the Given
HTML
Code - Alternative Way to Assign a Function to a Variable in JavaScript
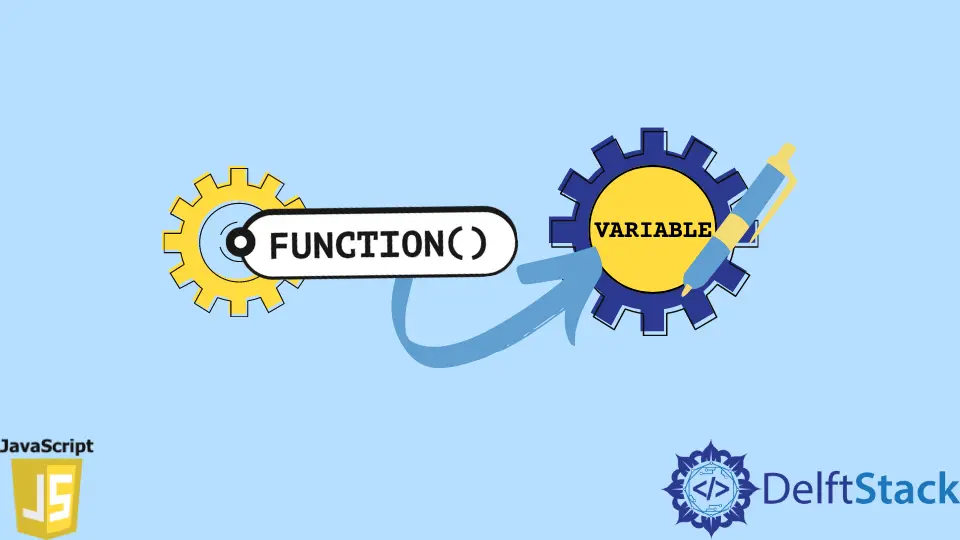
This article explains how we assign a declared function to any variable and check whether the function contains parameters or not. The function associated with the variable may return any value and store that value in a variable; we can also call the function using its assigned variable as many times as we want.
var variable_name = function functionName() {};
Example of Assigning a Function to a Variable Using JavaScript in HTML
We will assign a function to a variable checkValue
that will identify if the given value by the user is even or odd. The function will be triggered whenever the user inserts a value in input and press the submit
button; that function must be called and display an alert box that should say value is even
or value is odd
.
<!DOCTYPE html>
<html>
<head>
<title>
HTML | Assign Function to Variable Example
</title>
<script type="text/javascript">
</script>
</head>
<body>
<h2>Hi Users Check your choosen value is a even or odd.</h2>
<form id="form" onsubmit="return false;">
<input type="text" id="userInput" maxlength="1" placeholder="Enter any number" />
<input type="submit" onclick="checkValue();" />
</form>
<script>
// function to check value is even or odd
var checkValue = function() {
var givenValue = document.getElementById("userInput").value;
if(givenValue % 2 == 0){
alert(givenValue+" is even")
}
else {
alert(givenValue+" is odd")
}
}
</script>
</body>
<html>
In the above HTML
page source, you can see a simple input form type of number to get the integer value from the user, and there is a submit
button to submit the value and proceed with the functionality.
Here, you can see the <script>
tags, which is necessary to use JavaScript
statements in doctype HTML. We have assigned a function to our declared variable checkValue
in those tags.
The function contains a user-given value in the variable givenValue
. In the next step, we used a conditional statement with the modulus operator (%
) to check whether the remainder of the given value equals 2
or not.
In the next step, the function()
is simply displaying an alert box to the user containing given value is even
or given value is odd
based on conditions.
Examine the Given HTML
Code
Follow all the steps below to have a clear understanding of the code:
var variable_name = function functionName() {};
-
Create a text document using a notepad or any other text editing tool.
-
Paste the given code in the created text file.
-
Save that text file with the extension of
.html
and open it with any default browser. -
You can see the input form to insert value and the
submit
button; using that button, you can check your value.
Alternative Way to Assign a Function to a Variable in JavaScript
The same results will also be achieved, as shown below. Assign a function to a variable with a parameter to pass a value, check the value, and return the statement on a conditional basis.
var checkEvenOdd =
function(value) {
// will check passed value
if (value % 2 == 0) {
return value + ' is even'
} else {
return value + ' is odd'
}
}
console.log(checkEvenOdd(2))
Output:
2
In the above examples, checkEvenOdd
assigned with a function in which we have to pass an integer value as a parameter function will check that value with a given if
condition and returns a string. In the next step, the function checkEvenOdd()
is called in console.log()
to display the result.
Related Article - JavaScript Function
- JavaScript Return Undefined
- Self-Executing Function in JavaScript
- How to Get Function Name in JavaScript
- JavaScript Optional Function Parameter
- The Meaning of => in JavaScript
- Difference Between JavaScript Inline and Predefined Functions
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript