How to Check Undefined and Null Variable in JavaScript
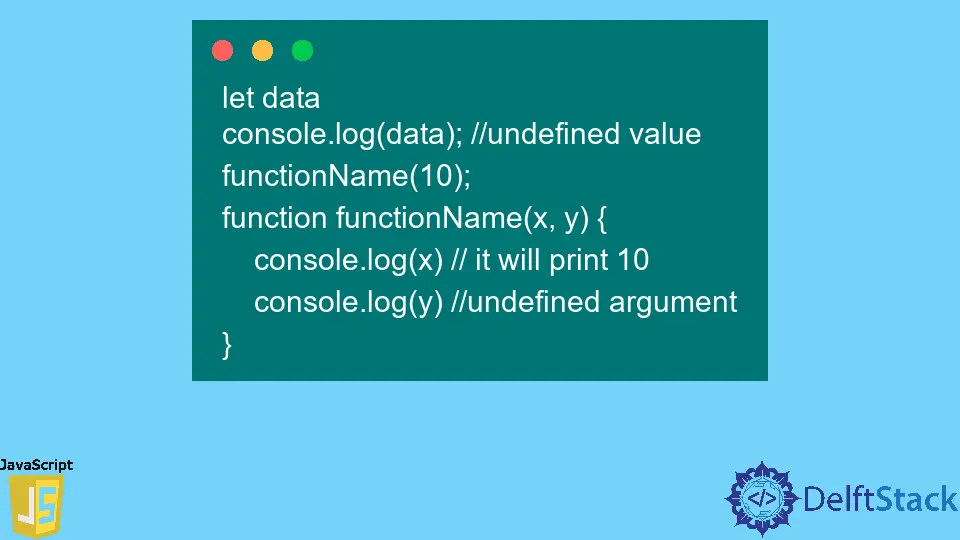
In this article, we will learn about undefined or null values in JavaScript source code and how we can use a conditional statement to check undefined and null variables in JavaScript.
Check Undefined Variable in JavaScript
It is a primitive value that specifies that the value is not assigned, and the variable not assigned with any type of value is known as an undefined variable. JavaScript directly sets the undefined value when programmers do not provide the value.
For example, if we declare a variable and try to display it in logs before initialization, it will get an undefined result by default. Non-existing elements for array returns are undefined also.
In JavaScript, if function arguments are not passed according to the parameters of that function, then the unpassed arguments are set to undefined
.
Syntax:
let data
console.log(data); // undefined value
functionName(10);
function functionName(x, y) {
console.log(x) // it will print 10
console.log(y) // undefined argument
}
Example Code:
let data
checkVariable(data) // it will generate undefined result
data = 10; // now initialized
checkVariable(data) // it will generate defined result
function checkVariable(data) {
if (data === undefined) {
console.log('variable is undefined')
} else {
console.log('variable is defined')
}
}
Output:
"variable is undefined"
// after initialzation
"variable is defined"
In the above code, we declared the data
variable and passed that variable to function checkVariable
as a parameter. That function is declared to get value as an argument and check with the conditional statement if else
if the value is undefined
then it will display "variable is undefined"
result in logs, or else it will display defined.
We have reassigned the data
variable with value 10 and passed it again to the function, and this time it will print "variable is defined"
.
Check Null Variable in JavaScript
There is null which is treated as an object in JavaScript to represent an empty or unknown value. For example, let x = null
the x variable is empty at the moment and may have any value later; it is a null variable.
Undefined and null values are defined as false values in JavaScript. If we use that value in default boolean()
function of JavaScript it will return false
result.
Syntax:
let data = null
console.log(data) // it will get null
Example Code:
let data = null;
checkNull(data) // will print null
data = 10; // now initialized
checkNull(data) // will print not null
function checkNull(data) {
if (data === null) {
console.log('variable is null')
} else {
console.log('variable is not null')
}
}
Output:
"variable is null"
// after initialization
"variable is not null"
In the above code, we declared the data
variable, assigned it with null
, and passed that variable to function checkNull
as a parameter. The function is declared to get value as an argument and check with the conditional statement if else
if the value is equal to null, then it will display "variable is null"
result in logs, or else it will display not null.
We reassigned the data
variable and passed it to the function; this time, it will print "variable is not null"
.