How to Access the Session Variable in JavaScript
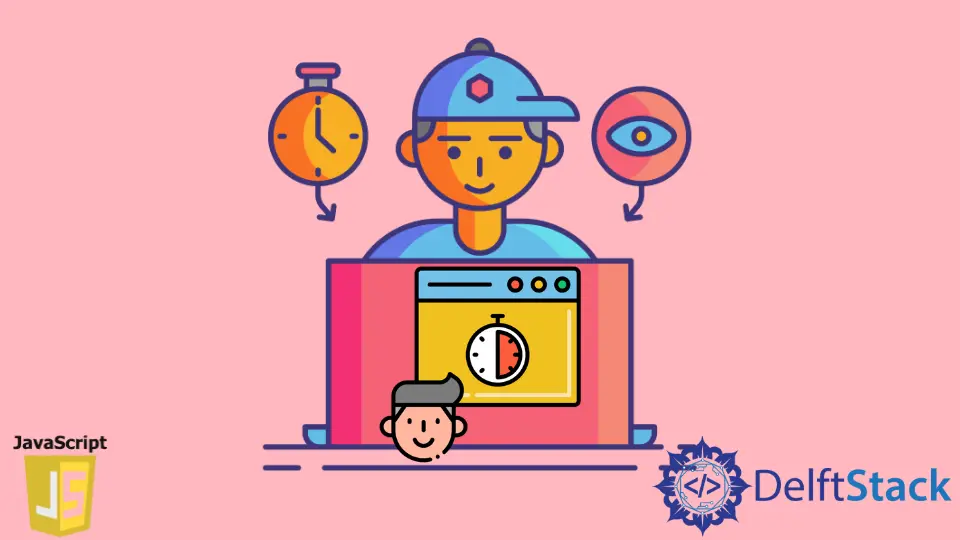
Whenever you open a browser and visit a website, a session is created. During this time, till your browser window is open, whatever you do, everything like unique session id, log-in details, personal information, etc., will be recorded and stored inside a cookie. These details are sent back and forth between the server and the client to know who is accessing the website.
Let’s say you have written a code that stores the username
of the user in ASP.NET or C# with the help of session state.
protected void Page_Load(object sender, EventArgs e) {
Session["UserName"] = "John_Wick";
}
In the C# code above, we have a Page_Load()
method. This method will be called as soon as the page is loaded. This method has a session variable, UserName
, used to store the user’s username.
Access the Session Variables in JavaScript
Accessing the various session is easy in JavaScript. Let’s see how to access it with the help of the below code.
Below we have an HTML document. Inside the head
tag, we have added a jQuery CDN since we will be using jQuery, a JavaScript library. Inside our body
, we have an empty div
tag displaying the user’s username fetched from the session variable. It has an id
of greetings
. Using this id
, we will be targeting this div
inside our jQuery code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- jquery cdn -->
<script src="https://code.jquery.com/jquery-3.6.0.js" integrity="sha256-H+K7U5CnXl1h5ywQfKtSj8PCmoN9aaq30gDh27Xc0jk=" crossorigin="anonymous"></script>
<title>Session Variable</title>
</head>
<body>
<div id="greeting"></div>
<script type="text/javascript">
$(function () {
var name = 'Welcome ' + ' <%= Session["UserName"] %>'
$('#greeting').text(name)
});
</script>
</body>
</html>
Output:
Welcome John_Wick
Inside our script
tag, we only have a single function called when the page will be loaded. To access the session variable which we have defined in our ASP.NET or C# language, we just have to pass that variable name as it is inside this <%= %>
tag as <%= Session["UserName"] %>
. Note that this tag is enclosed inside a string. And then, we are prepending another string called Welcome
before our session variable string.
Finally, we are storing the result inside the name
variable. Then we add the content of the name
variable inside the div
that has an id of greetings
. It will then display the user’s username on the web browser Welcome John_Wick
as an output.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn