Why Global Variables Give Undefined Values in JavaScript
- Scope in JavaScript
- The Issue with Global Variables in JavaScript
- Concept of Variable Hoisting in JavaScript
- Shadowing Global Variables in JavaScript
- Avoiding Undefined Values with Global Variables in JavaScript
- Conclusion
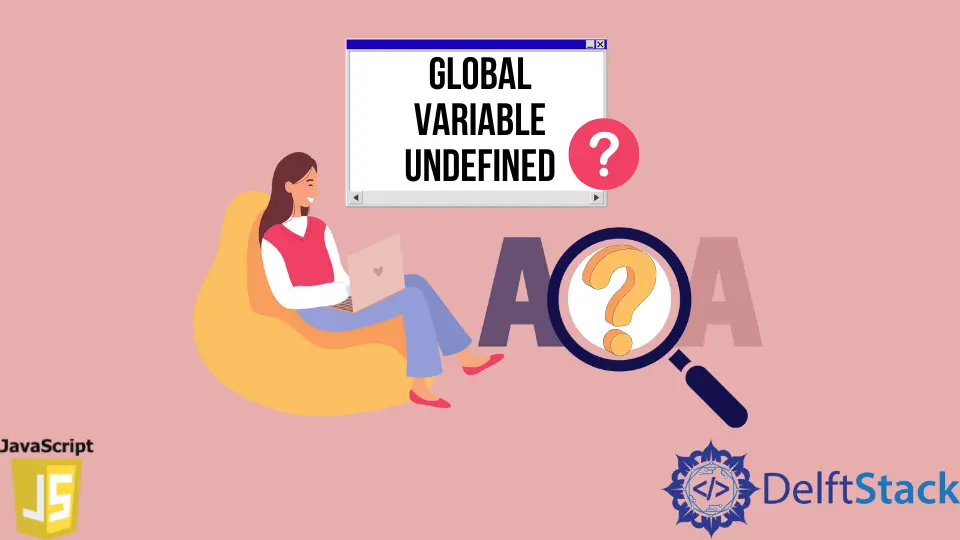
JavaScript, a versatile and widely used programming language, can sometimes be tricky to work with, especially when it comes to variable scope and hoisting. Global variables, those defined outside of any function or block, can exhibit unexpected behavior, often leading to the infamous "undefined"
value.
JavaScript is one of the oldest programming languages. Since it is compiled and executed by the browser, how it works is somewhat different from the systems-level languages like C or Java.
The behavior of the JavaScript programming language can be a little weird to many beginner programmers. To understand the JavaScript language completely, you have to thoroughly understand various concepts from this language, like event loop, bubbling and capturing, call stack, and many more.
In this article, we will explore the intricacies of why global variables can sometimes yield undefined values in JavaScript. We’ll delve into topics such as variable hoisting, shadowing, and best practices to help you navigate this common issue.
Scope in JavaScript
Before we dive into the specifics of global variables, it’s crucial to understand the concept of scope in JavaScript. Scope determines the accessibility or visibility of variables in a particular part of your code during runtime. JavaScript has three main scopes:
- Global Scope: Variables declared outside of any function or block have global scope. They can be accessed anywhere in the code, making them available to all functions and blocks.
- Function Scope: Variables declared within a function have function scope. They are only accessible within that function and any nested functions.
- Block Scope (introduced in ECMAScript 6): Variables declared with
let
orconst
inside a block (e.g., inside anif
statement or a loop) are only accessible within that block.
The Issue with Global Variables in JavaScript
Global variables in JavaScript are variables declared outside of any function or block. They have a global scope, meaning they can be accessed from anywhere in the code.
Global variables play a crucial role in storing data or configurations that need to be accessible throughout an entire program. However, their unrestricted availability can lead to unexpected behaviors, especially in complex applications.
Global variables, while convenient, can lead to unexpected behavior due to their wide accessibility. This becomes particularly apparent when variables are declared but not assigned a value.
Consider the following example:
var globalVariable;
function setGlobalValue() {
globalVariable = 42;
}
setGlobalValue();
console.log(globalVariable);
In this example, globalVariable
is declared in the global scope but not assigned any value. When setGlobalValue()
is called, it assigns the value 42
to globalVariable
. Finally, console.log(globalVariable)
prints 42
to the console.
However, if we omit the call to setGlobalValue()
, the output will be undefined
. This is because, by default, variables declared without an explicit value are assigned the value undefined
.
Concept of Variable Hoisting in JavaScript
JavaScript has a concept called “hoisting”, where all the variable declarations present in the JavaScript code are taken out and assigned an undefined
value during compile time. Similarly, all the function declarations inside JavaScript are taken out and stored inside the global scope.
This is called variable hoisting and is another factor that contributes to the “undefined” issue with global variables. This is regardless of whether the variable or function scope is local or global.
Global variables are hoisted to the top of the global execution context, which means they can be accessed even before they are officially declared in the code (even if you declare a variable later in your code, it’s as if it was declared at the beginning).
Let’s now understand how the concept of hoisting works in JavaScript and how it assigns undefined
values to variables in JavaScript. Consider the following example:
function hoistingExample() {
console.log(myVariable); // Output: undefined
var myVariable = 10;
}
In this example, myVariable
is accessed before it’s declared. However, due to hoisting, it’s effectively moved to the top of the function scope. This results in undefined
being logged to the console.
In another example, we have defined a global variable called abc
and a function a()
.
let abc;
function a() {
console.log('This is a function...');
}
console.log(abc);
debugger
abc = 5;
console.log(abc);
Output:
undefined
5
Here, have you noticed that you can use the variable abc
even before it is initialized? Have you ever wondered how this is possible?
This thing is not allowed in other programming languages. The reason this is happening is because of hoisting.
The above code represents the first console statement. Here, adding a debugger is important to see the state of the variables inside the Chrome dev tools.
After scanning the JavaScript code, the JavaScript compiler scans all the variables and functions, adds them to the global scope, and assigns undefined to the abc
variable.
Then the JavaScript engine starts executing the code line by line, and as soon as it finds the variable abc
, it is assigned some value, in this case, 5
. It will assign 5
to the variable abc
in the global scope.
The below output represents the second console statement after the variable has been assigned a value of 5
.
This is how the concept of variables and function hoisting works. This is the actual reason why the global variables in JavaScript give the undefined
value.
Shadowing Global Variables in JavaScript
Another potential source of confusion is when a local variable inside a function shares the same name as a global variable. This situation is known as “variable shadowing”.
In this scenario, the local variable takes precedence over the global variable within the function.
Consider the following example:
var shadowedVariable = "global";
function shadowingExample() {
var shadowedVariable = "local";
console.log(shadowedVariable); // Output: local
}
shadowingExample();
Here, we have a global variable, shadowedVariable
, and a local variable with the same name inside the function shadowingExample()
. When console.log(shadowedVariable)
is called, it refers to the local variable, resulting in the output local
.
Avoiding Undefined Values with Global Variables in JavaScript
In order to prevent encountering undefined values with global variables, it’s advisable to follow these best practices:
- Always Initialize Global Variables: Ensure that global variables are initialized with a value when they are declared.
- Use
let
andconst
for Block Scope: Prefer usinglet
andconst
for variables with limited scope, as they are block-scoped and can help avoid unintentional global declarations. - Avoid Variable Shadowing: Be mindful of naming conflicts between global and local variables. Use distinct names to minimize potential issues.
- Use Strict Mode: Enable strict mode in your JavaScript files (
"use strict";
). This mode enforces better coding practices and provides clearer error messages.
Conclusion
This article explores global variable behavior in JavaScript, focusing on why they can return undefined values. It covers scope concepts, variable hoisting, and shadowing.
Key tips include initializing global variables, using let
and const
for block scope, avoiding variable shadowing, and enabling strict mode for better coding practices. Understanding these nuances empowers developers to write more reliable and efficient code.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn