How to Mask Variable Value in JavaScript
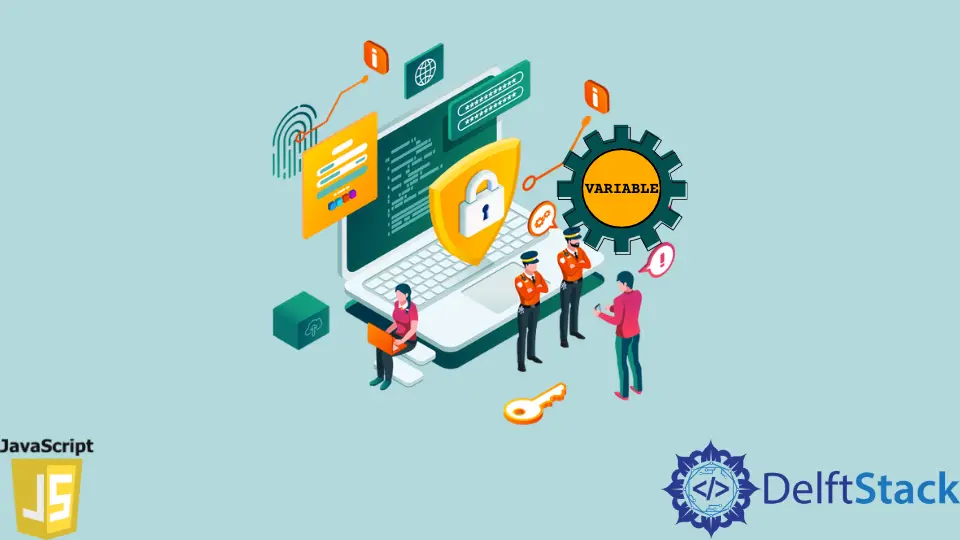
In this article, we will learn how to mask the variable values in a JavaScript source code and the benefits of masking in JavaScript.
Use the replace()
Method With Regular Expression to Mask Variable Value in JavaScript
In programming, Mask is a user-predefined pattern used to make changes in data that can be provided by the user or can be initialized to a variable as a variable value. This alteration process is known as masking.
Masking is the process of intentionally securing the data, and we can de-mask it to re-generate its original form. In JavaScript, we have a default method of replace()
, and we can use that method with the regular expression regex
to alter and mask our data.
the replace()
Function in JavaScript
In JavaScript, replace()
is a pre-defined method, and we use it on strings to replace the defined portion of that string with another. It searches the defined string portion from the complete declared string and replaces it with the given value.
The original string does not change by the replace()
method. It returns the updated string.
Example:
let string = 'Delft stack is a good website to learn programming.';
let result = string.replace('good', 'best'); // it will replace good with best
console.log(result);
Output:
Delft stack is a best website to learn programming.
Regular Expression in JavaScript
The regular expression is the sequence of characters that helps us search for a specific pattern.
let regex = /hi/i
It can be used for operations like text search or text replacement. We can alter the existing value with another defined value if we use regex in the replace()
method.
Alteration:
let regex = /hi/i
let text = 'hi world'
let result = text.replace(/hi/i, 'hello'); // it will replace hi with hello
console.log(result);
Output:
hello world
In the example below, we will use the replace()
method and regex
together to perform masking on the initialized phone number.
Example of Masking Variable Value:
let regex = /^(\d{3})(\d{4})(\d{4}).*/
let value = '11122223333';
let altered = value.replace(regex, '$1-$2-$3');
console.log('original value ' + value)
console.log('altered value ' + altered)
Output:
original value 11122223333
altered value 111-2222-3333
In the above JavaScript source code, we have initialized the regex pattern in the variable and assigned numbers data in the value
variable. Then, we have called the replace()
method on the value
variable and passed the defined regex to alter the number data with -
separating the data.
Finally, we have displayed the result and comparison of the original and altered data in the log box using console.log()
.