How to Declare Multiple Variables in JavaScript
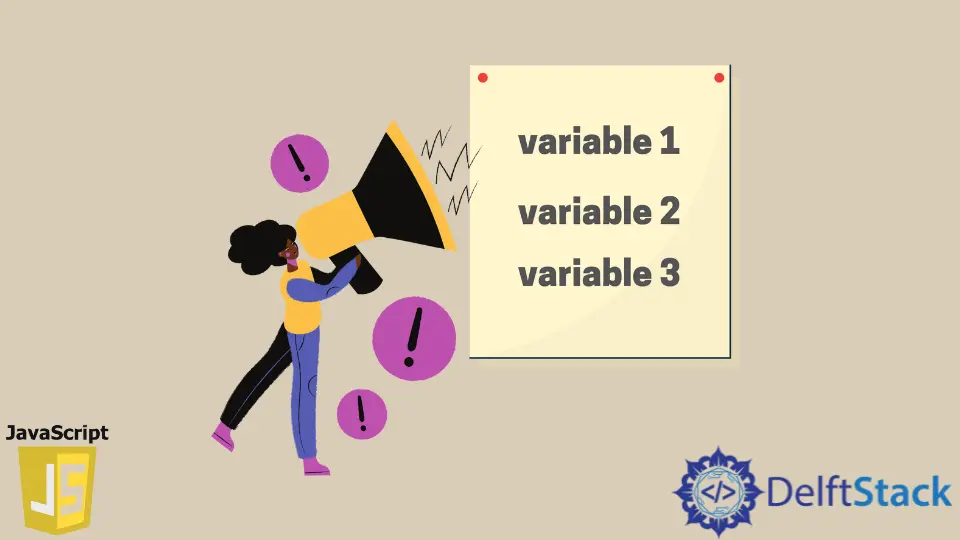
Variables are containers in JavaScript that hold reusable data. They’re like cups filled with stuff that can be used repeatedly depending on how we want to use it.
Here are some JavaScript tips for declaring multiple variables.
Declare Multiple Variables in JavaScript
The most common method for declaring and initializing JavaScript variables is to write each variable on its line.
Example Code:
var cost = 25;
const name = 'Onion';
let currency = 'NPR';
However, there are shorter ways to declare multiple variables in JavaScript. First, you can only use one variable keyword (var
, let
, or const
) and declare variable names and values separated by commas.
Example Code:
let name = 'Shiv', age = 22, message = 'What\'s Up?';
The declaration above is only one line long, but it is more challenging to read than separated declarations. In addition, all variables are declared with the same keyword, let
.
Other variable keywords, such as const
and var,
are not permitted. You can also use comma-separated declarations to create a multi-line declaration.
Example Code:
let name = 'Shiv', age = 22, message = 'What\'s Up?';
Finally, the de-structuring assignment can be used to reveal and preprocess multiple variables in a single line.
Example Code:
const [name, age, message] = ['Shiv', 22, 'What\'s Up?'];
console.log(name);
console.log(age);
console.log(message);
The array elements are extracted and assigned to the variables declared on the =
operator’s left side in the code above.
Output:
"Shiv"
22
"What's Up?"
The code examples above demonstrate how to declare multiple variables in a single line of JavaScript. Still, declaring variables one by one is the most common method because it separates the declaration into its line, like the following example below.
Example Code:
const name = 'Shiv';
let age = 22;
let message = 'What\'s Up?';
console.log(name);
console.log(age);
console.log(message);
As your project grows, the code above will be the easiest to change.
Output:
"Shiv"
22
"What's Up?"
When using comma-separated declaration and de-structuring assignment, you can only use one variable keyword, so you can’t change the variables from const
to let
without changing them all. In their own way, these variable types are distinct and help speed up code development.
However, it is recommended to use let
whenever possible. You can use const
whenever the variable’s value must remain constant.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn