JavaScript Optional Function Parameter
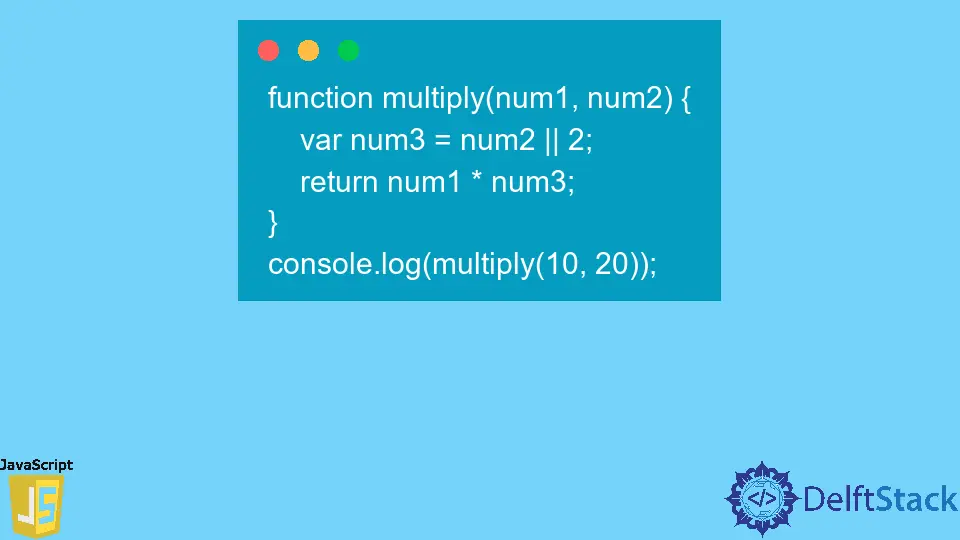
Initializing the optional function parameter makes the code easier to implement, improves the readability of the code, and simplifies the code without getting errors and repeats. It allows customization of the code by adding numerous arguments and parameters.
This article will discuss how to use optional function parameters in JavaScript.
JavaScript Optional Function Parameter
Parameters are the definitions used to define functions. In JavaScript, we use two main parameters in a function call. The mandatory and optional parameters.
It is a must to pass the mandatory parameters; otherwise, it will throw an error. It returns undefined
, but in optional parameters, when no parameters or undefined passed, it will be initialized to the default value.
When declaring optional function parameters, we can mainly take three approaches.
- Using Undefined Property
- Using Arguments Variable
- Using Logical OR Operator
Use Undefined Property
When calling functions in JavaScript, we can omit parameters and fill in the rest parameters with the value undefined
.
Code:
function number(num1, num2) {
if (num1 === undefined) {
num1 = 2;
}
if (num2 === undefined) {
num2 = 2;
}
return num1 * num2;
}
console.log(number(5, 6));
console.log(number(5));
Output:
30
10
In the above snippet, the function number()
is called two times. At first, it passed two parameters for num1
and num2
to the function and assigned only one parameter for num1
.
Even though the code works fine and displays the output as 30
at the first calling, in the second calling, it states the value 10
after considering the given value as num1
. When the function checks whether the number
variable is undefined or not, the value of num2
remains as 2
.
However, with this method, we can only make the last argument optional and not make the first, middle, or combination of parameters optional.
Use Arguments Variable
Arguments are built-in objects in JavaScript functions. It returns an array of parameters when the function call.
This array’s length refers to the number of parameters passed. Using a conditional statement, it checks the number of parameters passed and passes default values in place of undefined parameters.
Then, we can find out all of the parameters while looping through the argument objects.
Code:
function number(num1, num2) {
if (arguments.length == 0) {
num1 = 1;
num2 = 2;
}
if (arguments.length == 1) {
num2 = 2;
}
return num1 * num2;
}
console.log(number(10, 15)); // first calling
console.log(number(10)); // second calling
Output:
150
20
According to the above snippet, the function number()
checks the number of parameters used in the arguments.length
property. If the result gets 0
, no parameters are passed to the function; if it gets 1
, only the num1
parameter is passed.
After that, the function number()
is called twice by getting two and one parameters. Even in the first calling, it worked fine and displayed the output as 150
; in the second calling, it showed the result as 20
after assigning 2
for num2
as the arguments.length
is equal to 1
.
Use Logical OR
(||
) Operator
In this method, the optional parameter is logical OR
(||
) with the default value within the function’s body. Here, the short-circuit OR operator returns true
if the left side of the left argument is true
; otherwise, it checks and returns true
if the right-hand argument is true
.
Using OR operator is a simple method for creating flexible and readable optional arguments and enables easy management of optional parameters. Furthermore, False
, 0
, null
, undefined
, the empty string " "
, and NaN
are all invalid arguments.
Code (first calling):
function multiply(num1, num2) {
var num3 = num2 || 2;
return num1 * num3;
}
console.log(multiply(10, 20));
Output:
200
Code (second calling):
function multiply(num1, num2) {
var num3 = num2 || 2;
return num1 * num3;
}
console.log(multiply(10));
Output:
20
In the example above, it has declared a function named multiply
with two parameters, num1
and num2
are called two times. Here, we have to call the two functions separately.
At the first calling, it passed two parameters and displayed the output as 200
, while in the second calling, it only passed one parameter and displayed the result as 20
. In the second calling, as num2
is undefined, 2
is assigned instead.
As in the undefined property method, we can only make the last argument optional and not make the first, middle, or combination of parameters optional.
Conclusion
The optional function parameter can identify as a nice feature in JavaScript. Even using optional parameters performs best in simplifying code; it is an infrequently used functionality.
If we want to call a function frequently with the same values for some parameters, we can avoid repetition by making those parameters optional. For that, we can use the above methods as per suitability.