JavaScript Return Undefined
-
undefined
in JavaScript -
Scenarios That Return
undefined
as the Output -
Difference Between
undefined
andnull
- Conclusion
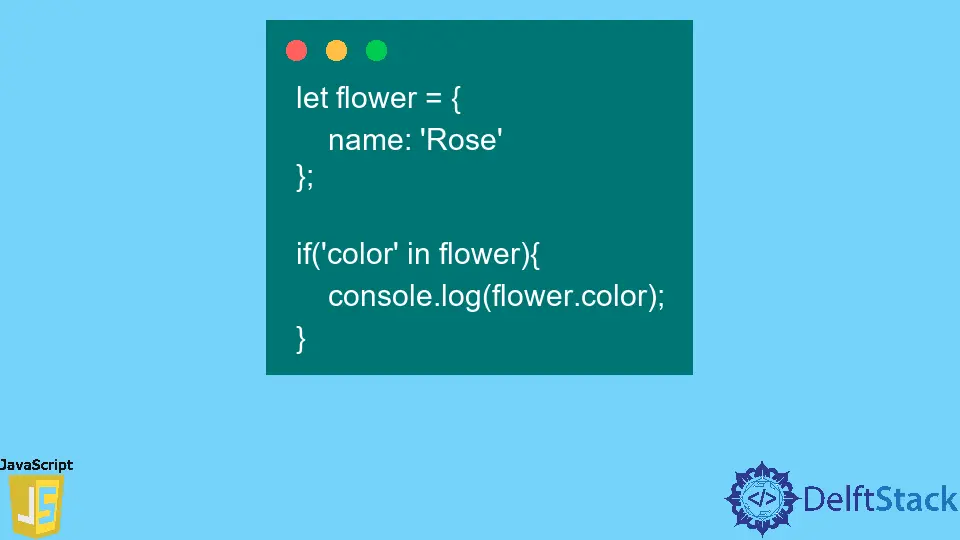
JavaScript has fewer libraries or frameworks than other programming languages. null
and undefined
represent empty values in JavaScript.
To reduce the errors that occur along with undefined
, it is a must to have a clear understanding of the reason for returning undefined
. Let’s have a quick overview of undefined
in JavaScript.
undefined
in JavaScript
Among the six primitive types of JavaScript, undefined
is a special type of primitive with its value as undefined. Simply the only value the undefined type has is undefined.
undefined
is a variable of global scope that is a non-writable and non-configurable property. According to the ECMA specifications, the value undefined is returned when a variable has not been assigned a value.
// uninitialized variable
let letters;
console.log(letters);
// non-existing array elements
let vowels = ['a', 'e'];
console.log(vowels[3]);
// non-existing object properties
let flower = {name: 'Rose'};
console.log(flower.color);
Output:
undefined
undefined
undefined
As it seems in the output, the value undefined
is returned when accessing an uninitialized variable, non-existing array elements or object properties. After running each code chunk separately, we can get three undefined
outputs.
Let’s see the situations where JavaScript returns undefined
and some best practices to resolve returning undefined
.
Scenarios That Return undefined
as the Output
Accessing an Uninitialized Variable
If we declare a variable without initializing a value, the variable will return undefined.
Code:
let letters;
console.log(letters);
Output:
undefined
As in the code chunk, none of the values is assigned to the declared variable letters
. As you see in the output above, it returns undefined
with the calling of the variable, but if we assign a value for the variable, it returns the value below.
Code:
let letters = 5;
console.log(letters);
Output:
5
Accessing a Non-Existing Property
In the case of accessing a non-existing property, undefined
is returned instead of throwing an error. The below example proves that well.
Code:
let flower = {name: 'Rose'};
console.log(flower.color);
Output:
undefined
Here the object property flower
has one property as the name
. But when accessing, we have called the color
property, which is a non-existing property of that object.
As shown in the output, it returns the value undefined
as the output.
As the best practice, we can check the property’s availability using the below syntax before accessing it. Then we can get rid of returning undefined
as the output.
Syntax:
'propertyName' in objectName
Code:
let flower = {name: 'Rose'};
if ('color' in flower) {
console.log(flower.color);
}
Calling Function Parameters
When we call a function with fewer arguments instead of the defined number of arguments, it returns undefined
as it doesn’t pass all arguments. Therefore, we have to call the function with the same number of arguments.
Code:
function multiply(a, b, c) {
a;
b;
c;
return a * b;
}
console.log(multiply(5, 3));
Output:
15
It has assigned values for the a
and b
, 5
and 3
, respectively when calling the function with two arguments so that it returns 15
as the output after assigning those values.
The following code calls the same function with the same two values after altering the expression of the return statement to multiply a
, b
and c
altogether.
Code:
function multiply(a, b, c) {
a;
b;
c;
return a * b * c;
}
console.log(multiply(5, 3));
Output:
NaN
As shown in the output, it returns NaN
instead of computational values. The reason for that appearing is as it doesn’t pass all arguments and the value for c
remains undefined
.
the return
Statement of a Function
Another instance where the output returns as undefined
are if any function doesn’t have a return
statement or has a return
statement without an expression nearby.
For example, let’s declare a function named sq_num
that generates a square number according to the given input.
Code:
// doesn't have a return statement
function sq_num(x) {
const result = x * x;
}
console.log(sq_num(2));
// return statement without an expression
function sq_num(x) {
const result = x * x;
return;
}
console.log(sq_num(2));
Output:
undefined
undefined
We must declare the return
statement and an expression mentioned in the below code to work this function sq_num
as expected. Through that, we can get the computational result of the function as below.
Code:
function sq_num(x) {
const result = x * x;
return result;
}
console.log(sq_num(2));
Output:
4
Accessing Out-Of-Bound Elements in Arrays
If we access elements not defined within the array, it can be considered as accessing out-of-bound elements. If such a situation occurs, the undefined
value is returned.
Code:
const animals = ['elephant', 'tiger', 'monkey'];
console.log(animals[5]);
console.log(animals[-1]);
Output:
undefined
undefined
In the same way, sparse arrays present the same problem. Sparse arrays are arrays with gaps and non-indexed elements.
When accessing the empty slots of the array, it also returns the value undefined.
Code:
const SparseArray = ['elephant', , 'monkey'];
console.log(SparseArray);
Output:
["elephant", undefined, "monkey"]
Here the array SparseArray
is created with three elements, missing the second. As seen below, it returns undefined when accessing the second element.
Code:
const SparseArray = ['elephant', , 'monkey'];
console.log(SparseArray[1]);
Output:
undefined
Apart from the above scenarios, the value undefined is returned along with the void operator. Below are examples of how it returns undefined despite the evaluation result.
Code:
void 2;
console.log(void (true));
Output:
undefined
Difference Between undefined
and null
JavaScript has both null
and undefined
values representing empty values.
When a variable does not have an explicitly assigned value, JavaScript assigns it the primitive value of undefined. As the name implies, null is a primitive value representing an intentional absence of any other assigned value.
Using the typeof
operator, we can see a distinction between null and undefined as below.
typeof undefined;
typeof null;
undefined
object
Overall, null indicates missing objects, while undefined is the value used in an uninitialized variable.
Conclusion
This article describes the occurrence of undefined along with the uninitialized variables, non-existing properties, out-of-bound indexes and the void operator.
Along with that discussed, some of the best practices can apply while using along with the examples. Apart, we looked at the difference between null and the defined values and their usage instances.
As a good practice, we should be responsible for reducing the occurrence of undefined by reducing the usage of uninitialized variables and sparse arrays.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.