How to Check If String is Anagram in JavaScript
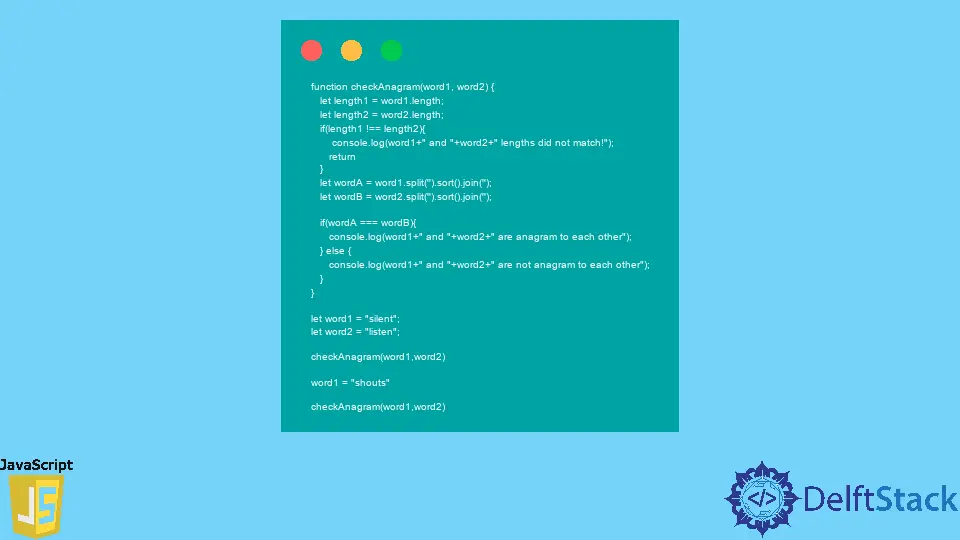
In this article, we will learn the concept of anagram in JavaScript. We will learn how to check if a string is an anagram of another string in JavaScript.
The Concept of Anagram
Two words or strings that have the same characters with the same numbers are known as anagram words. The length of a word should also be the same.
Using anagram words, we can rearrange one word’s characters to generate another. Here are a few examples of anagram words:
listen
andsilent
triangle
andintegral
the eyes
andthey see
As we know, we can rearrange the characters of one word and transform the second word with the help of an algorithm.
Anagram Finder in JavaScript
In JavaScript, we can create a program to check whether two words or strings are an anagram of each other or not. We can create a function that can check whether the words are anagrams and return the boolean result as true or false.
First, we should sort both strings and compare the sorted string to find out whether they are identical.
The following example will use a JavaScript program to perform an anagram checking algorithm for two words. We will use the default JavaScript methods split()
, sort()
, and join()
to check the provided words.
The split()
method is used to split the string characters and generate an array of characters. The sort()
method is used to sort the array of elements.
The join()
method is used to join the array of elements and generate a string of elements.
function checkAnagram(word1, word2) {
let length1 = word1.length;
let length2 = word2.length;
if (length1 !== length2) {
console.log(word1 + ' and ' + word2 + ' lengths did not match!');
return
}
let wordA = word1.split('').sort().join('');
let wordB = word2.split('').sort().join('');
if (wordA === wordB) {
console.log(word1 + ' and ' + word2 + ' are anagram to each other');
} else {
console.log(word1 + ' and ' + word2 + ' are not anagram to each other');
}
}
let word1 = 'silent';
let word2 = 'listen';
checkAnagram(word1, word2)
word1 = 'shouts'
checkAnagram(word1, word2)
Output:
"silent and listen are anagram to each other"
"shouts and listen are not anagram to each other"
As shown above, we declared a let
type function checkAnagram()
in which we will take words as a parameter. Inside that function, we have used a conditional statement on passed words.
Using a conditional statement, we have checked first whether the length of both words is equal or not. If not, we display the lengths did not match
message in logs.
Then, we used split()
, sort()
, and join()
on passed words to perform sorting on words and store sorted words in separate variables word1
and word2
.
Then, we need to check whether both variables’ values are equal or not using the conditional statement if-else
. If equal, the words are an anagram; if not, the words are not an anagram.
We have initialized two words, silent
and listen
, and passed them to the checkAnagram()
function as an argument, updated the word1
, and called checkAnagram()
again.
The console.log()
method displayed the result in logs. You can copy and save the above source code and use the JavaScript compiler to see the result.