How to Sort 2D Array in Java
- Understanding 2D Arrays in Java
-
Sort a 2D Array in Java Column-Wise Using the
Comparator
Interface -
Sort a 2D Array in Java Row-Wise Using the
Arrays.sort()
Method - Conclusion
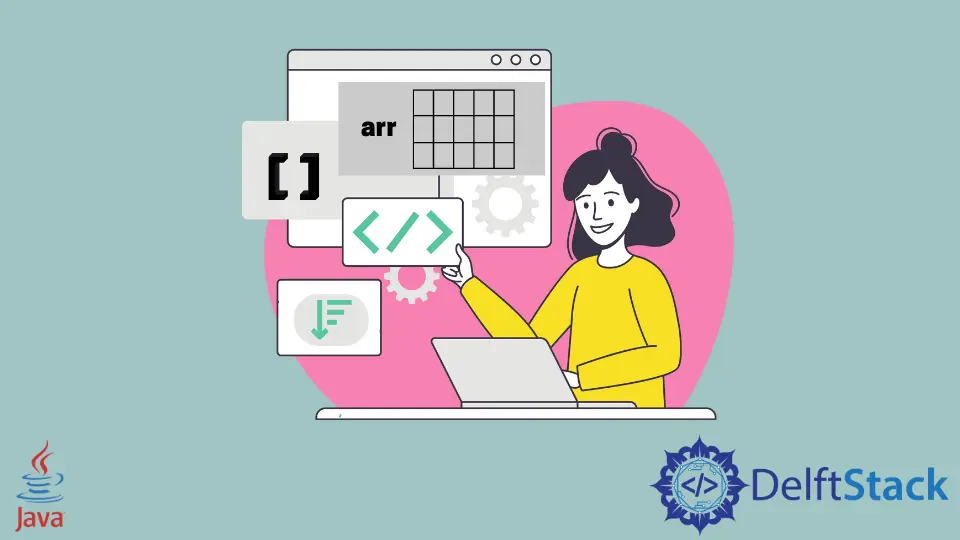
Sorting a 2D array is a common task in Java, essential for various applications ranging from data analysis to algorithmic problem-solving. In this article, we will explore multiple approaches to sorting a 2D array in Java, providing you with a deep understanding of the techniques involved.
Understanding 2D Arrays in Java
Before diving into sorting methods, let’s establish a foundation on 2D arrays in Java. A 2D array is essentially an array of arrays where elements are arranged in rows and columns.
Each row in a 2D array can be considered an independent 1D array.
int[][] twoDArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Sort a 2D Array in Java Column-Wise Using the Comparator
Interface
Sorting a 2D array in Java is a common task, and doing so column-wise provides valuable insights into the data. One effective approach involves utilizing the Comparator
interface along with the Arrays.sort()
method from the java.util
package.
The Comparator
interface in Java provides a way to define custom ordering for objects. It contains a single method, compare()
, which takes two arguments and determines their order.
A negative, zero, or positive value is returned if the first argument is less than, equal to, or greater than the second, respectively.
When sorting a 2D array column-wise, we will create a Comparator
that compares elements based on a specified column. The Arrays.sort()
method will then use this comparator to rearrange the array accordingly.
Sort a 2D Array in Java Column-Wise Code Example
Let’s consider a 2D array named multi
with three rows and three columns. We’ll sort this array based on its third column using the Comparator
interface.
import java.util.Arrays;
import java.util.Comparator;
public class Sort2DArrayColumnWise {
public static void main(String args[]) {
int[][] multi = {{4, 9, 8}, {7, 5, 2}, {3, 0, 6}};
displayArray(multi);
sort2DArrayColumnWise(multi, 3);
System.out.println("After sorting column-wise");
displayArray(multi);
}
public static void sort2DArrayColumnWise(int[][] array, final int columnNumber) {
Arrays.sort(array, new Comparator<int[]>() {
@Override
public int compare(int[] first, int[] second) {
return Integer.compare(first[columnNumber - 1], second[columnNumber - 1]);
}
});
}
public static void displayArray(int[][] array) {
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) System.out.print(array[i][j] + " ");
System.out.println();
}
}
}
Here, we begin by declaring a class named Sort2DArrayColumnWise
. In the main
method, a 2D array named multi
is created and initialized with integer values. This array represents the data we want to sort.
int[][] multi = {{4, 9, 8}, {7, 5, 2}, {3, 0, 6}};
Before sorting, the displayArray
method is called to print the original contents of the 2D array. The displayArray
method uses nested loops to iterate through the rows and columns of the array, printing each element.
displayArray(multi);
Next, the sort2DArrayColumnWise
method is invoked to perform the column-wise sorting. This method utilizes the Arrays.sort()
method, which accepts the 2D array and a custom comparator.
sort2DArrayColumnWise(multi, 3);
Inside the sort2DArrayColumnWise
method, an anonymous class implementing the Comparator<int[]>
interface is defined. The compare
method of this interface is overridden to specify the sorting criteria.
Arrays.sort(array, new Comparator<int[]>() {
@Override
public int compare(int[] first, int[] second) {
return Integer.compare(first[columnNumber - 1], second[columnNumber - 1]);
}
});
The comparator compares two rows based on the values in a specified column (columnNumber
).
The Integer.compare()
method is used to compare the elements in the chosen column. This comparator is responsible for determining the order of rows during the sorting process.
Finally, after sorting, the program prints the sorted 2D array using the displayArray
method.
Code Output:
This output demonstrates the 2D array sorted column-wise based on the values in the third column. Understanding and utilizing the Comparator
interface provides a versatile tool for custom sorting in Java.
Sort a 2D Array in Java Row-Wise Using the Arrays.sort()
Method
Now, let’s delve into the process of sorting a 2D array in Java, but this time, we’ll sort it row-wise. Individually sorting rows can be beneficial in scenarios where you want to maintain the integrity of each row while arranging the rows themselves.
Row-wise sorting involves sorting each row of the 2D array independently of the others. For this purpose, we will use the Arrays.sort()
method.
The Arrays.sort()
method in Java is a convenient tool for sorting arrays. When applied to a 2D array, it can sort each row individually, providing a row-wise arrangement.
Unlike column-wise sorting using a comparator, row-wise sorting using Arrays.sort()
doesn’t require a custom comparator; it sorts based on the natural order of the elements.
Sort 2D Array Row-Wise Code Example
Consider a 2D array named array
with 4 rows and 4 columns. The following code demonstrates how to sort each row in ascending order using the Arrays.sort()
method:
import java.util.Arrays;
public class Sort2DArrayRowWise {
public static void main(String args[]) {
int array[][] = {{7, 8, 2, 1}, {0, 3, 2, 9}, {6, 5, 3, 2}, {8, 3, 7, 9}};
displayArray(array);
sortRowWise(array);
System.out.println("After sorting row-wise");
displayArray(array);
}
static void sortRowWise(int arr[][]) {
for (int i = 0; i < arr.length; i++) {
Arrays.sort(arr[i]);
}
}
public static void displayArray(int[][] array) {
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) System.out.print(array[i][j] + " ");
System.out.println();
}
}
}
In this Java program, we start by declaring a class named Sort2DArrayRowWise
. In the main
method, a 2D array named array
is created and initialized with integer values. This array represents the data we want to sort.
int array[][] = {{7, 8, 2, 1}, {0, 3, 2, 9}, {6, 5, 3, 2}, {8, 3, 7, 9}};
Before sorting, the displayArray
method is called to print the original contents of the 2D array. The displayArray
method uses nested loops to iterate through the rows and columns of the array, printing each element.
displayArray(array);
Then, the sortRowWise
method is invoked to perform the row-wise sorting. This method utilizes the Arrays.sort()
method, which operates on 1D arrays.
Inside the method, a loop iterates through each row, and the Arrays.sort()
method is applied to sort each row individually.
sortRowWise(array);
Finally, after sorting, the program prints the sorted 2D array using the displayArray
method.
System.out.println("After sorting row-wise");
displayArray(array);
The sortRowWise
method is responsible for sorting each row of the 2D array independently. It contains a loop that iterates through each row, and the Arrays.sort()
method is applied to sort the elements in ascending order.
static void sortRowWise(int arr[][]) {
for (int i = 0; i < arr.length; i++) {
Arrays.sort(arr[i]);
}
}
The displayArray
method is a utility function to print the contents of a 2D array. It uses nested loops to traverse the array and print each element.
public static void displayArray(int[][] array) {
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[i].length; j++) System.out.print(array[i][j] + " ");
System.out.println();
}
}
Code Output:
This output demonstrates the 2D array sorted row-wise, preserving the relative order of elements within each row. Row-wise sorting can be beneficial in scenarios where maintaining the integrity of each row is essential.
Conclusion
Sorting a 2D array in Java is a fundamental task that can be achieved through various approaches, each tailored to specific requirements. Whether sorting column-wise using the Comparator
interface or row-wise with the simplicity of Arrays.sort()
, Java provides versatile tools for organizing multi-dimensional data.
When sorting column-wise, using the Comparator
interface allows us to define custom ordering criteria. This approach provides flexibility for complex sorting scenarios, giving control over how elements are compared and arranged.
On the other hand, row-wise sorting using the Arrays.sort()
method offers a straightforward solution, particularly when the natural order of elements suffices. This method simplifies the sorting process by applying it directly to each row without the need for a custom comparator.
Both methods enable the efficient handling of 2D arrays in Java. Whether you need to arrange data based on specific columns or simply sort rows independently, these approaches provide valuable tools for enhancing the organization and readability of your Java programs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn