How to Return Empty Array in Java
-
Return an Empty Array Using
new int[0]
in Java - Return an Empty Array Using Empty Curly Braces in Java
-
Return an Empty Array Using
org.apache.commons.lang3.ArrayUtils
- Return an Empty Array Using Empty Array Declaration in Java
- Conclusion
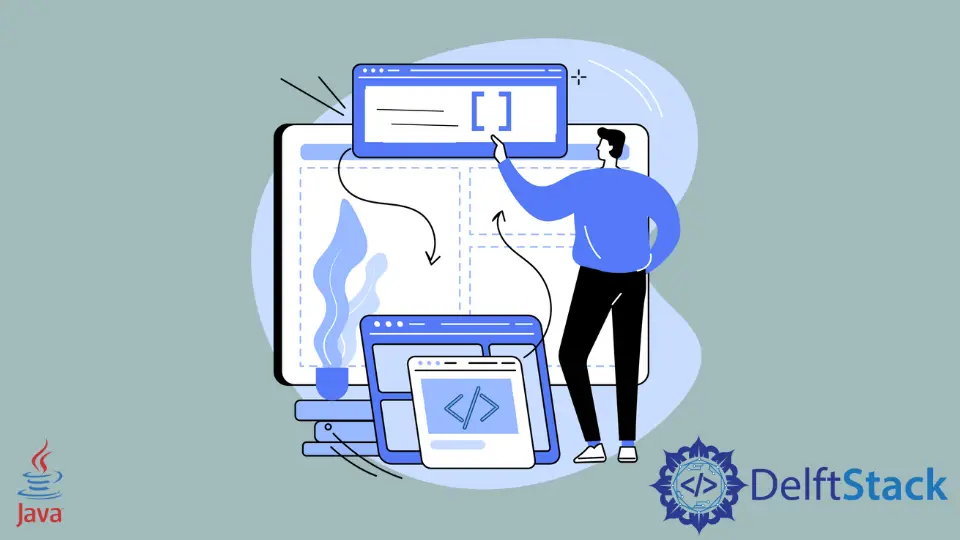
Empty arrays can be useful in scenarios where representing the absence of elements is necessary, particularly when dealing with APIs that may return null arrays.
In this article, we will discuss four approaches to achieve this: using new int[0]
, empty curly braces {}
, ArrayUtils
from Apache Commons Lang, and empty array declaration int[]{}
.
Return an Empty Array Using new int[0]
in Java
Every array has a fixed size that we can specify when we create the array. If the array has a length of zero, then it does not contain any element.
To return an empty array from a function, we can create a new array with a zero size using the expression new int[0]
. This expression initializes a new integer array with zero length, effectively resulting in an empty array.
It’s a concise and straightforward method to represent an array without any elements.
In the example below, we define a method named returnEmptyArray()
that returns an empty array of integers using new int[0]
. We then demonstrate how to use this method and retrieve the length of the empty array.
public class EmptyArrayExample {
public static void main(String[] args) {
int[] emptyArray = returnEmptyArray();
System.out.println("Length of the empty array: " + emptyArray.length);
}
private static int[] returnEmptyArray() {
return new int[0];
}
}
Output:
Length of the empty array: 0
As you can see, we defined a method named returnEmptyArray()
that returns an empty array using new int[0]
. In the main
method, we then called returnEmptyArray()
to obtain an empty array and then print its length.
After we ran the program, it printed the length of the empty array, which is 0.
Return an Empty Array Using Empty Curly Braces in Java
Empty curly braces, { }
, in Java, represent an empty array or collection. When used in the context of array initialization, it denotes an array with zero elements, essentially an empty array.
This approach allows us to explicitly declare an array with no elements, providing a clear and direct way to represent such data structures.
In the example below, we define a method named returnEmptyArray
that returns an empty array of integers using empty curly braces. We then demonstrate how to use this method and retrieve the length of the empty array.
public class EmptyArrayExample {
public static void main(String[] args) {
int[] emptyArray = returnEmptyArray();
System.out.println("Length of the empty array: " + emptyArray.length);
}
private static int[] returnEmptyArray() {
int[] emptyArr = {};
return emptyArr;
}
}
Output:
Length of the empty array: 0
In the main
method, we declared an int
array named emptyArray
and assigned the result of calling the returnEmptyArray
method. This method call is used to obtain an empty array.
Then, the length of this empty array is printed to the console using System.out.println
.
The returnEmptyArray
method simply declares an empty integer array emptyArr
using empty curly braces {}
, indicating an array with zero elements. The method then returns this empty array.
After running the program, it should print the length of the empty array, which is 0.
Return an Empty Array Using org.apache.commons.lang3.ArrayUtils
Before using ArrayUtils
, you need to ensure you have Apache Commons Lang library added to your Java project. You can download the JAR file from the Apache Commons website and include it in your project’s build path.
Alternatively, if you are using a build tool like Maven, you can add the following dependency to your pom.xml
:
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version> <!-- Use the latest version available -->
</dependency>
This dependency ensures that we have access to ArrayUtils
and other utility functions provided by Apache Commons Lang.
The ArrayUtils
class offers various static fields to return empty arrays of different types, including boolean
, char
, and String
. By utilizing the ArrayUtils
class to return empty arrays, we simplify the process of array initialization, especially when we need an empty array of a specific data type.
Rather than manually initializing an empty array using curly braces or the new
keyword, we can use the appropriate constant from ArrayUtils
.
Using EMPTY_STRING_ARRAY
of the ArrayUtils
Class
To return an empty array using ArrayUtils
, you can use the EMPTY_STRING_ARRAY
, EMPTY_BYTE_ARRAY
, EMPTY_INT_ARRAY
, EMPTY_LONG_ARRAY
, etc., fields, depending on the type of array you need.
In this example, we’ll focus on using ArrayUtils.EMPTY_STRING_ARRAY
to return an empty array of the String
type.
import org.apache.commons.lang3.ArrayUtils;
public class EmptyArray {
public static void main(String[] args) {
String[] getEmptyArray = returnEmptyArray();
System.out.println("Length of the empty array: " + getEmptyArray.length);
}
private static String[] returnEmptyArray() {
return ArrayUtils.EMPTY_STRING_ARRAY;
}
}
When we run the program, it outputs the length of the empty array, which is 0.
Length of the empty array: 0
To get started, we imported the ArrayUtils
class at the beginning of the code. Following this, we defined a class named EmptyArray
, which hosts the main
method—the starting point of our Java program.
Inside the main
method, we called a method named returnEmptyArray()
to obtain an empty array of strings.
The returnEmptyArray()
method is encapsulated within the EmptyArray
class, marked as private, indicating that it’s accessible only within this class. Inside this method, we simply returned ArrayUtils.EMPTY_STRING_ARRAY
, a predefined constant representing an empty array of strings.
Finally, we printed the length of the empty array we obtained using System.out.println
. The length of an empty array is zero since it doesn’t contain any elements.
Using the toArray()
Method of the ArrayUtils
Class
In addition to using ArrayUtils
’ constants like EMPTY_STRING_ARRAY
, the Apache Commons Lang library also provides the toArray()
method in the ArrayUtils
class. This method allows us to convert an empty list into an array.
While it’s designed for converting a list, we can cleverly utilize it to create an empty array.
Let’s explore how to achieve this:
import org.apache.commons.lang3.ArrayUtils;
public class EmptyArray {
public static void main(String[] args) {
String[] getEmptyArray = returnEmptyArray();
System.out.println("Length of the empty array: " + getEmptyArray.length);
}
private static String[] returnEmptyArray() {
// Converting an empty list to an array (effectively creating an empty array)
return ArrayUtils.toArray();
}
}
Output:
Length of the empty array: 0
In this example, we’ve updated the returnEmptyArray()
method to use ArrayUtils.toArray()
. This method returns an empty array, which is what we need.
private static String[] returnEmptyArray() {
return ArrayUtils.toArray();
}
When we run the program, it still prints the length of the empty array, confirming that we’ve successfully obtained an empty array using toArray()
.
Return an Empty Array Using Empty Array Declaration in Java
In Java, an array declaration involves specifying the data type and the variable name, along with optional square brackets []
to denote an array. An empty array declaration, such as int[]{}
, is a way to represent an array structure without any specified elements.
The empty brackets indicate an array structure, but since there are no elements specified within the brackets, the array remains empty.
Let’s illustrate the process of returning an empty array using the empty array declaration int[]{}
with a simple example:
public class EmptyArrayExample {
public static void main(String[] args) {
int[] emptyArray = returnEmptyArray();
System.out.println("Length of the empty array: " + emptyArray.length);
}
private static int[] returnEmptyArray() {
return new int[] {};
}
}
Output:
Length of the empty array: 0
As we can see, inside main
, we called another method named returnEmptyArray()
. Inside this method, we used the empty array declaration new int[]{}
to create an empty array of integers in Java.
Back in the main
method, we obtained this empty array by calling returnEmptyArray()
, and then we printed the length of this empty array using System.out.println
. The length of an empty array is zero, as there are no elements in it.
Conclusion
We have explored various methods to return an empty array in Java.
The new int[0]
and empty curly braces {}
methods provide a direct and straightforward way to represent an array without any elements. On the other hand, ArrayUtils
from Apache Commons Lang offers predefined constants and utility methods for creating empty arrays of various types, simplifying array initialization.
Lastly, the empty array declaration int[]{}
allows for an explicit declaration of an empty array structure. Each method achieves the same result of returning an empty array, providing developers with flexibility based on their preferences and project requirements.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn